在 JavaScript 中從字串中刪除字元
Kirill Ibrahim
2023年1月30日
2020年12月19日
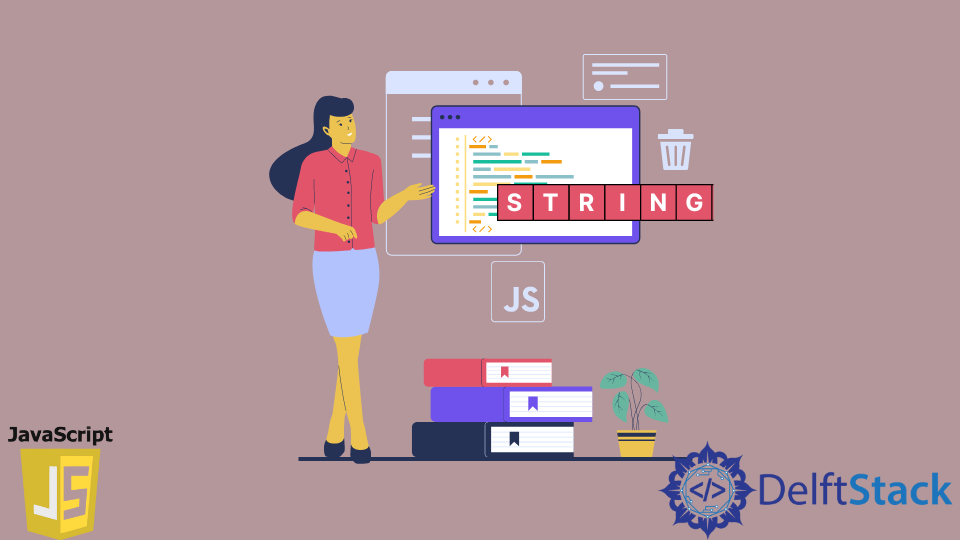
JavaScript 有不同的方法來刪除字串中的特定字元。我們將介紹如何在 JavaScript 中刪除字串中的字元。
在 JavaScript 中使用正規表示式的 replace()
方法
在 JavaScript 中,我們使用正規表示式的 replace()
方法來刪除字串中指定字元的所有例項。
JavaScript replace()
正規表示式語法
replace(/regExp/g, '');
例子:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove all instances of the specified character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove all instances of the specified character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace(/t/g, '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
輸出:
The original string is DelftStack
New Output is: DelfSack
在 JavaScript 中刪除指定索引處的指定字元
當我們需要刪除一個字元時,當一個字串中有多個該字元的例項時,例如,從字串 DelftStack
中刪除字元 t
,我們可以使用 slice()
方法得到給定索引前後的兩個字串,並將其連線起來。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove Specified Character at a Given Index in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove Specified Character at a Given Index in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString(5)">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = (position) => {
originalWord = 'DelftStack';
newWord = originalWord.slice(0, position - 1)
+ originalWord.slice(position, originalWord.length);
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
在 JavaScript 中刪除字串中字元的第一個例項
我們可以使用 replace()
方法,但不使用正規表示式,在 JavaScript 中只刪除字串中的第一個字元例項。我們把要刪除的字元作為第一個引數,把空字串''
作為第二個引數。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove First Instance of Character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove First Instance of Character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace('t', '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>