JavaScript 中的 Map 索引
Muhammad Muzammil Hussain
2022年5月10日
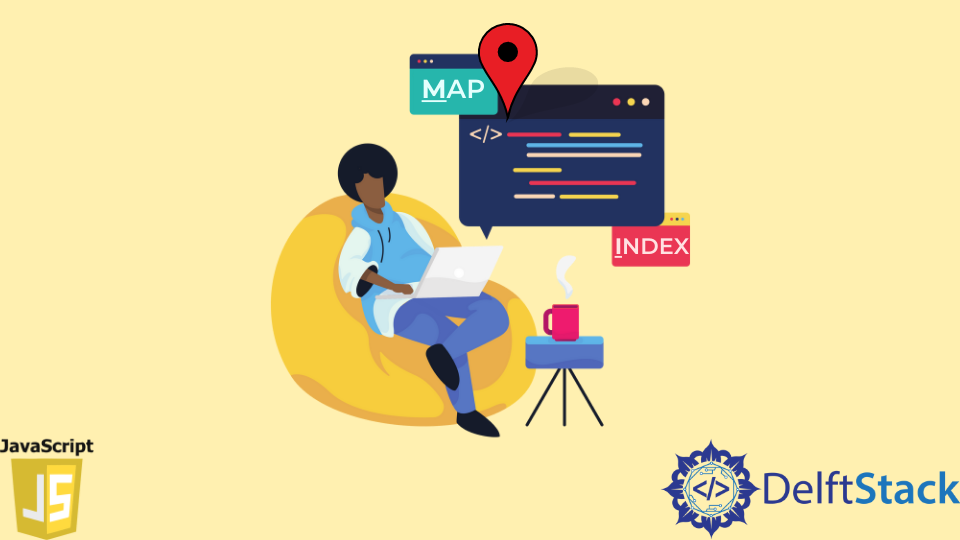
JavaScript map 方法很容易實現,我們將討論它的不同引數以及它們在不同場景中的使用方式。
在 JavaScript 中使用 array.map()
方法
JavaScript array.map()
方法為陣列的每個元素呼叫一個特定的函式,並因此返回一個新陣列。
重要的是該方法不會更改原始陣列。它返回一個新的處理陣列。
語法:
array.map(call_Back_Function(element, index, arr){
// Code to perform operation on array
}, this)
array.map()
方法的不同引數是
call_Back_Function
是一個特定的函式,它在對舊陣列執行定義的操作後產生新的陣列元素。element
是我們將操作的原始陣列的當前元素。index
是我們將對其執行特定操作的當前元素的索引。arr
是原始陣列,我們將在其上執行所需的操作以獲取新的已處理陣列。這裡它的值是可選的。- 回撥函式值執行期間的
this
用作this
。它的值也是可選的。
返回
作為 array.map()
方法的結果,我們得到一個新陣列,其每個元素都是從 call_Back_Function
生成的。
以下是未呼叫的 array.map()
方法:
- 從未設定的索引。
- 所有這些索引已被刪除。
何時不使用 array.map()
我們不能在以下情況下使用 array.map()
方法:
- 如果沒有我們需要執行所需操作的陣列,請不要使用
array.map()
方法。 - 如果你需要陣列元素保持不變而不執行任何操作,請避免使用
array.map()
方法。
在下面的程式碼行中,我們將把一個數字陣列
對映到一個平方根陣列
。
var numbers = [1, 4, 9];
var square_roots = numbers.map(function(numbers) {
return Math.sqrt(numbers)
})
// So, orignal array remained the same, numbers = [1, 4, 9]
// new processed array as output is, square_roots = [1, 2, 3]
在下文中,array.map()
方法在對十進位制數陣列
進行操作後返回一個新的已處理整數陣列。
var orignal_array = [1.5, 4.3, 6.4, 8.7];
var new_array = orignal_array.map(Math.round);
document.writeln(new_array);
// Here orignal_array will remain same [1.5, 2.3, 5.4]
// new processed array output is [2, 4, 6, 9]
同樣,我們可以使用 array.map()
方法重新格式化 array
物件。
var array =[{id:1,name:"owais"},
{id:2,name:"ali"},
{id:3,name:"ahmed"}]
array.map(function(obj){
console.log(obj.id)
console.log(obj.name)
})
// console output
1
"owais"
2
"ali"
3
"ahmed"
此外,以下示例演示了我們可以在何處以及如何使用 JavaScript array.map()
方法。
示例 1:
<html>
<head>
<title>
JS map() method explanation
</title>
</head>
<body>
<h2>
JavaScript array map() method demonstration
</h2>
<!-- javascript array map method -->
<script>
let employees = ["Owais", "Ahmad","Hassan", "Mughira", "Wasim"];
employees.map((emp, index) =>
{
alert("Greetings__Mr. " + emp + "\n");
index++;
alert("On employee rank, You have got Position : " + index + "\n");
});
</script>
</body>
</html>
輸出:
Greetings__Mr. Owais
On employee rank, You have got position : 1
Greetings__Mr. Ahmad
On employee rank, You have got position : 2
Greetings__Mr. Hassan
On employee rank, You have got position : 3
Greetings__Mr. Mughira
On employee rank, You have got position : 4
Greetings__Mr. Wasim
On employee rank, You have got position : 5
示例 2:
<html>
<head>
<title> JavaScript map method demonstration </title>
</head>
<body>
<h1 style="color: brown;"> <u> map index in javascript array </u></h1>
<p><b> Here we have a word "DELFTSTACK" and by using JavaScript
map index we will identify element on every index.</b> </p>
<script>
const name = [ 'D', 'E', 'L', 'F', 'T', 'S','T','A','C','K'];
name.map((element, index) => {
document.write("Iteration no : " + index);
document.write("<br>");
document.write("Element = " + element);
document.write("<br>");
document.write("<br>");
return element;
});
</script>
</body>
</html>
輸出:
map index in javascript array
Here we have the word "DELFTSTACK," and we will identify elements on every index using JavaScript map index.
Iteration no : 0
Element = D
Iteration no : 1
Element = E
Iteration no : 2
Element = L
Iteration no : 3
Element = F
Iteration no : 4
Element = T
Iteration no : 5
Element = S
Iteration no : 6
Element = T
Iteration no : 7
Element = A
Iteration no : 8
Element = C
Iteration no : 9
Element = K
上面的例子描述了 JavaScript 中的 array.map()
方法和 callback
函式中作為引數的 indexing
方法。