JavaScript 中的陣列過濾
Jagathish
2023年1月30日
2022年5月1日
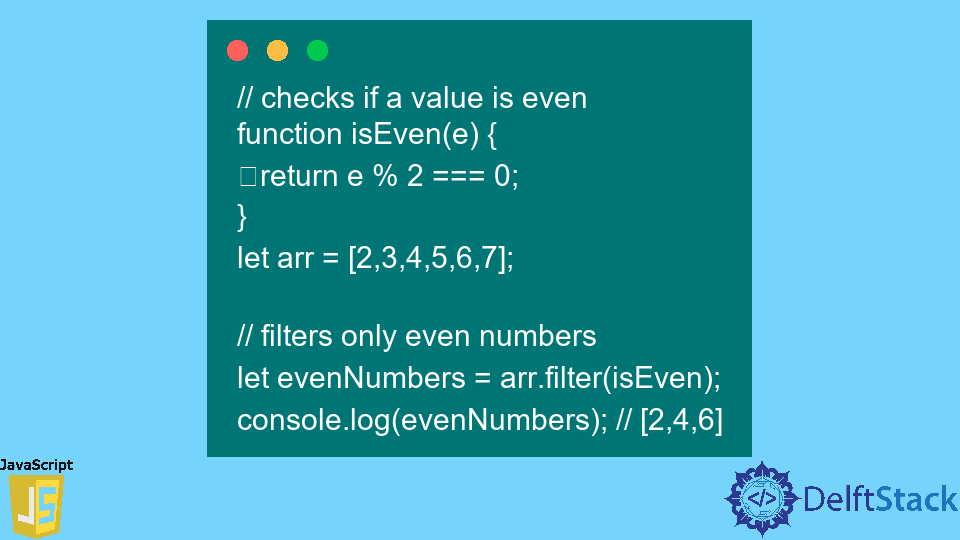
JavaScript 中的 filter()
方法返回一個新陣列,其中包含通過指定函式測試的所有元素。這篇文章將討論如何獲取匹配特定條件的陣列元素。
在 JavaScript 中使用 isEven()
函式
我們在一個陣列中有數字,我們需要選擇所有的偶數
元素。
例子:
// checks if a value is even
function isEven(e) {
return e % 2 === 0;
}
let arr = [2,3,4,5,6,7];
// filters only even numbers
let evenNumbers = arr.filter(isEven);
console.log(evenNumbers); // [2,4,6]
輸出:
input : [2,3,4,5,6,7]
output : [2,4,6]
在上面的程式碼中:
- 建立了一個函式
isEven
,它將接受一個引數。如果引數是偶
數,此函式返回true
。否則,將返回false
。 - 建立一個陣列
arr
,並以isEven
函式作為引數呼叫arr
的filter
方法。 filter
方法將遍歷所有陣列元素併為每個陣列元素執行isEven
方法。filter
方法將選擇isEven
方法返回2,4,6
值的所有元素。
在 JavaScript 中使用 isPalindrome()
函式
我們有一個字串陣列,我們需要選擇所有迴文
字串(一個向後讀和向前讀相同的字串,例如,mom
、dad
、madam
)。
例子:
// checks if a string is palindrome
function isPalindrome(str) {
// split each character of the string to an array and reverse the array and join all the characters in the array.
let reversedStr = str.split("").reverse().join("");
return str === reversedStr;
}
let arr = ['dad', 'god', 'love', 'mom']
// filters only palindrome string
let palindromeArr = arr.filter(isPalindrome);
console.log(palindromeArr); // ['dad', 'mom']
輸出:
input : ['dad', 'god', 'love', 'mom']
output : ['dad', 'mom']
在上面的程式碼中:
- 建立了一個函式
isPalindrome
,它將採用一個字串引數。如果引數是迴文,此函式返回true
。否則,將返回false
。 - 建立一個陣列
arr
,並以isPalindrome
函式作為引數呼叫arr
的filter
方法。 filter
方法將遍歷所有陣列元素併為每個陣列元素執行isPalindrome
方法。filter
方法將選擇isPalindrome
方法返回值'dad'
、'mom'
的所有元素。