在 Java 中更新 Hashmap 的值
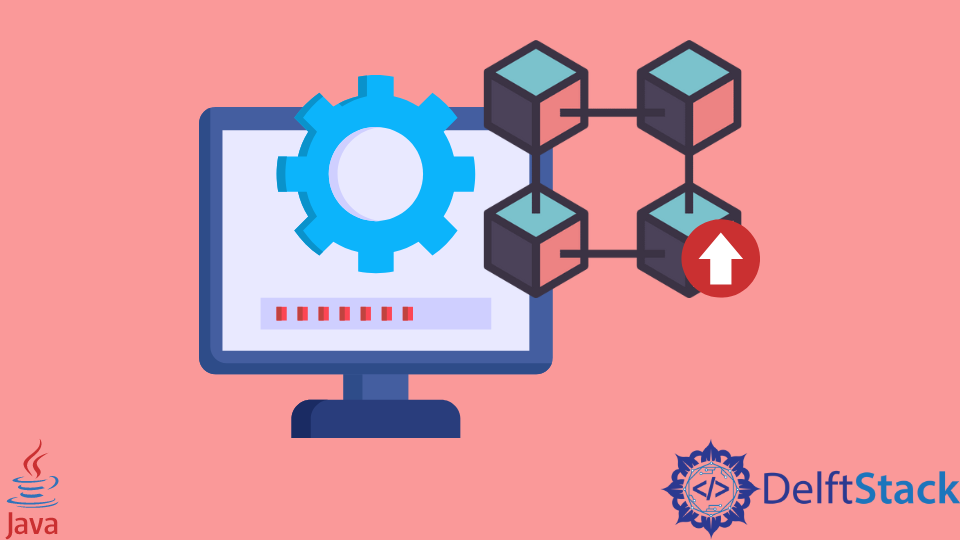
本文介紹瞭如何在 Java 中使用 HashMap 類中包含的兩個方法-put()
和 replace()
更新 HashMap 中的值。
在 Java 中使用 hashmap.put()
更新 Hashmap 中的值
當我們要在 HashMap
中插入一個值時,我們使用 put()
方法。而我們也可以用它來更新 HashMap
裡面的值。在下面的例子中,我們建立了一個 HashMap
的物件,這個物件是由鍵值對組成的,在初始化的時候需要定義鍵和值的資料型別。
我們對鍵和值都使用字串型別,我們可以使用鍵查詢或對值進行操作。下面,我們用一個新的值來替換有 key three
的值。如果在 HashMap
中沒有我們想要更新的存在,使用 put()
方法,就會插入一個新的值。輸出顯示的是更新後的值。
import java.util.HashMap;
public class UpdateHashmap {
public static void main(String[] args) {
HashMap<String, String> ourHashmap = new HashMap<>();
ourHashmap.put("one", "Alex");
ourHashmap.put("two", "Nik");
ourHashmap.put("three", "Morse");
ourHashmap.put("four", "Luke");
System.out.println("Old Hashmap: "+ourHashmap);
ourHashmap.put("three", "Jake");
System.out.println("New Hashmap: "+ourHashmap);
}
}
輸出:
Old Hashmap: {four=Luke, one=Alex, two=Nik, three=Morse}
New Hashmap: {four=Luke, one=Alex, two=Nik, three=Jake}
在 Java 中使用 hashmap.replace()
更新 Hashmap 中的值
HashMap
類的另一個方法是 replace()
,它可以更新或替換 HashMap
中的現有值。put()
和 replace()
的最大區別是,當 HashMap
中不存在一個鍵時,put()
方法會把這個鍵和值插入 HashMap
裡面,但 replace()
方法會返回 null。這使得 replace()
在更新 HashMap
中的值時,使用 replace()
更加安全。
在下面的例子中,我們建立一個 HashMap
並插入一些鍵值對。然後更新附加在鍵 three
上的值,我們使用 ourHashMap.replace(key, value)
,它需要兩個引數,第一個是我們要更新的鍵,第二個是值。
import java.util.HashMap;
public class UpdateHashmap {
public static void main(String[] args) {
HashMap<String, String> ourHashmap = new HashMap<>();
ourHashmap.put("one", "Alex");
ourHashmap.put("two", "Nik");
ourHashmap.put("three", "Morse");
ourHashmap.put("four", "Luke");
System.out.println("Old Hashmap: "+ourHashmap);
ourHashmap.replace("three", "Jake");
System.out.println("New Hashmap: "+ourHashmap);
}
}
輸出:
Old Hashmap: {four=Luke, one=Alex, two=Nik, three=Morse}
New Hashmap: {four=Luke, one=Alex, two=Nik, three=Jake}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn