Java 中的堆疊 pop 和 push 方法
Rupam Yadav
2023年10月12日
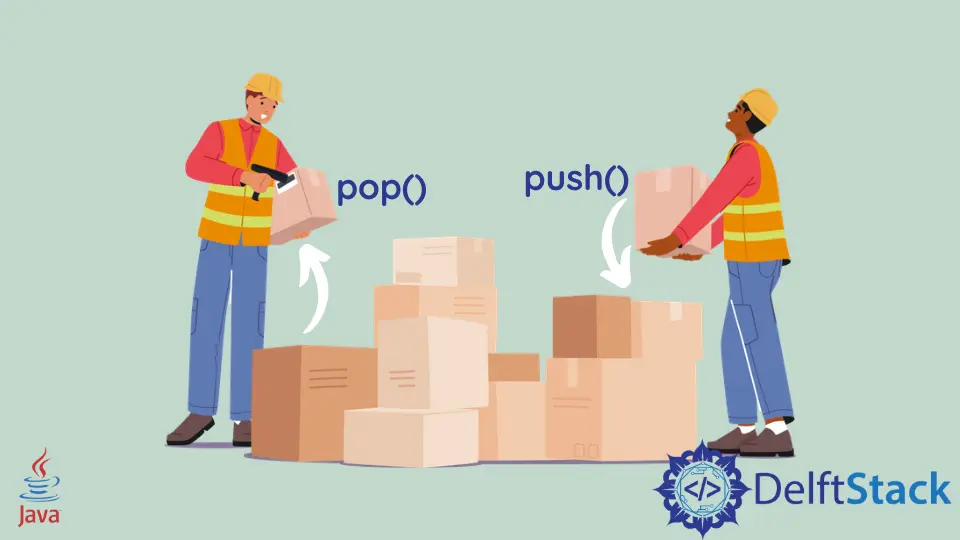
push 操作將一個元素新增到堆疊的最頂部位置,而 pop 操作刪除堆疊的最頂部元素。
我們將在下面的部分中介紹如何將堆疊的概念與 push 和 pop 操作一起使用。
在 Java 中使用 ArrayList
使用 Push Pop 堆疊
以下示例使用 ArrayList
來實現堆疊。首先,我們建立兩個類,一個是 ExampleClass1
,另一個是 StackPushPopExample
,我們在其中建立了堆疊中的 push 和 pop 操作的邏輯。
push()
方法:採用int
引數型別並將其新增到我們建立的列表的第一個位置。堆疊遵循後進先出的 LIFO 概念,將每個新專案新增到第一個位置並移動舊專案。pop()
函式:首先檢查堆疊是否為空,如果不是,則繼續刪除列表第零索引處的元素或堆疊的最頂部元素。
例子:
import java.util.ArrayList;
import java.util.List;
public class ExampleClass1 {
public static void main(String[] args) {
StackPushPopExample stackPushPopExample = new StackPushPopExample(5);
stackPushPopExample.push(2);
stackPushPopExample.push(3);
stackPushPopExample.push(4);
stackPushPopExample.push(7);
stackPushPopExample.push(1);
System.out.println("Topmost Element of the stack: " + stackPushPopExample.peek());
System.out.println("All Stack Items:");
for (Integer allItem : stackPushPopExample.getAllItems()) {
System.out.println(allItem);
}
stackPushPopExample.pop();
System.out.println("All Stack Items After popping one item:");
for (Integer allItem : stackPushPopExample.getAllItems()) {
System.out.println(allItem);
}
}
}
class StackPushPopExample {
private final List<Integer> intStack;
public StackPushPopExample(int stackSize) {
intStack = new ArrayList<>(stackSize);
}
public void push(int item) {
intStack.add(0, item);
}
public int pop() {
if (!intStack.isEmpty()) {
int item = intStack.get(0);
intStack.remove(0);
return item;
} else {
return -1;
}
}
public int peek() {
if (!intStack.isEmpty()) {
return intStack.get(0);
} else {
return -1;
}
}
public List<Integer> getAllItems() {
return intStack;
}
}
輸出:
Topmost Element of the stack:: 1
All Stack Items:
1
7
4
3
2
All Stack Items After popping one item:
7
4
3
2
為了檢視堆疊中的元素,我們建立了兩個函式,返回堆疊頂部專案的 peek()
方法和返回堆疊中所有專案的 getAllItems()
方法。
最後,pop()
函式刪除堆疊的第一個元素,然後再次列印堆疊以檢視該元素是否被刪除。
使用 Java 中的 Stack
類使用 Push Pop 堆疊
Java 中的集合框架提供了一個名為 Stack
的類,它為我們提供了在堆疊中執行所有基本操作的方法。在下面的程式碼片段中,我們將建立一個型別引數為 String
的 Stack
物件。
例子:
import java.util.Stack;
public class ExampleClass1 {
public static void main(String[] args) {
Stack<String> stack = new Stack<>();
stack.push("Item 1");
stack.push("Item 2");
stack.push("Item 3");
stack.push("Item 4");
stack.push("Item 5");
System.out.println("Topmost Element of the stack: " + stack.peek());
stack.pop();
System.out.println("After popping one item:");
System.out.println("Topmost Element of the stack: " + stack.peek());
}
}
輸出:
Topmost Element of the stack: Item 5
After popping one item:
Topmost Element of the stack: Item 4
要將元素新增到堆疊中,我們呼叫 push()
方法,要列印堆疊的第一個元素,我們呼叫 peek()
函式。
然後我們使用 pop()
方法刪除頂部專案,然後我們再次呼叫 peek()
方法來檢查 pop()
方法是否刪除了頂部專案。
與上一個示例相比,此方法的好處是執行相同操作所需的程式碼更少。
作者: Rupam Yadav
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn