Java 中 size 和 length 的區別
-
Java 中陣列的
length
屬性 -
Java 陣列中
.length
的示例 -
Java 陣列中
size()
方法的示例 -
使用 Java 中的
length()
方法查詢長度 -
Java Collections
size()
方法 - Java 中大小和長度的區別
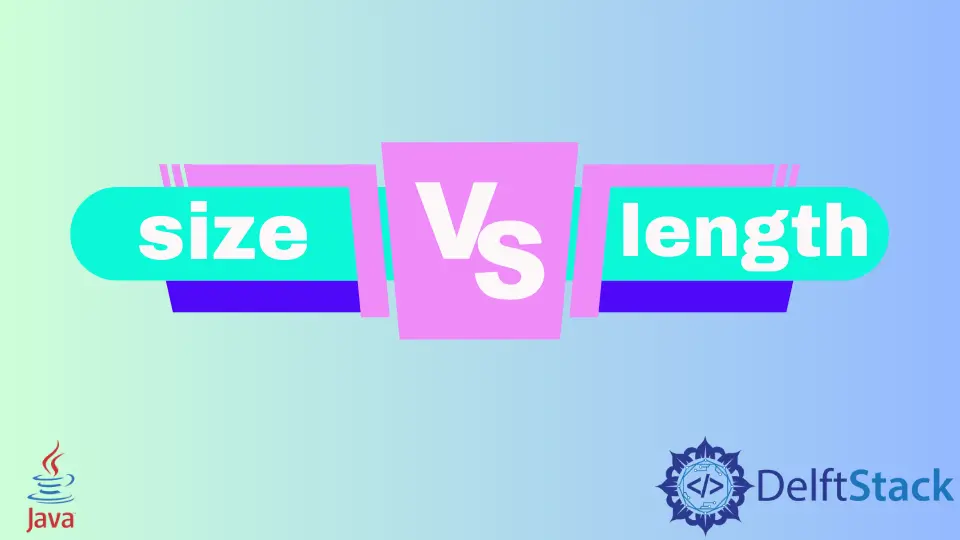
本教程介紹了 Java 中大小和長度之間的區別。我們還列出了一些示例程式碼以幫助你理解該主題。
Java 有一個 size()
方法和一個 length
屬性。初學者可能認為它們是可以互換的並執行相同的任務,因為它們聽起來有些相同。在 Java 中,大小和長度是兩個不同的東西。在這裡,我們將瞭解兩者之間的差異。
Java 中陣列的 length
屬性
陣列以有序的方式儲存固定數量的相同型別的資料。Java 中的所有陣列都有一個長度欄位,用於儲存為該陣列的元素分配的空間。它是一個常數值,用於找出陣列的最大容量。
- 請記住,該欄位不會為我們提供陣列中存在的元素數,而是可以儲存的最大元素數(無論元素是否存在)。
Java 陣列中 .length
的示例
在下面的程式碼中,我們首先初始化一個長度為 7
的陣列。即使我們沒有新增任何元素,該陣列的長度欄位仍顯示 7
。這個 7
只是表示最大容量。
public class Main {
public static void main(String[] args) {
int[] intArr = new int[7];
System.out.print("Length of the Array is: " + intArr.length);
}
}
輸出:
Length of the Array is: 7
現在,讓我們使用它們的索引將 3 個元素新增到陣列中,然後列印長度欄位。還是顯示 7。
public class Main {
public static void main(String[] args) {
int[] intArr = new int[7];
intArr[0] = 20;
intArr[1] = 25;
intArr[2] = 30;
System.out.print("Length of the Array is: " + intArr.length);
}
}
輸出:
Length of the Array is: 7
長度欄位是常量,因為陣列的大小是固定的。我們必須定義在初始化期間我們將儲存在陣列中的最大元素數(陣列的容量),我們不能超過這個限制。
Java 陣列中 size()
方法的示例
陣列沒有 size()
方法;它會返回一個編譯錯誤。請參考下面的示例。
public class SimpleTesting {
public static void main(String[] args) {
int[] intArr = new int[7];
System.out.print("Length of the Array is: " + intArr.size());
}
}
輸出:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot invoke size() on the array type int[]
at SimpleTesting.main(SimpleTesting.java:7)
使用 Java 中的 length()
方法查詢長度
Java 字串只是字元的有序集合,與陣列不同,它們具有 length()
方法而不是 length
欄位。此方法返回字串中存在的字元數。
請參考下面的示例。
public class Main {
public static void main(String[] args) {
String str1 = "This is a string";
String str2 = "Another String";
System.out.println("The String is: " + str1);
System.out.println("The length of the string is: " + str1.length());
System.out.println("\nThe String is: " + str2);
System.out.println("The length of the string is: " + str2.length());
}
}
輸出:
The String is: This is a string
The length of the string is: 16
The String is: Another String
The length of the string is: 14
請注意,我們不能在字串上使用 length
屬性,並且 length()
方法不適用於陣列。下面的程式碼顯示了我們濫用它們時的錯誤。
public class SimpleTesting {
public static void main(String[] args) {
String str1 = "This is a string";
System.out.println("The length of the string is: " + str1.length);
}
}
輸出:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
length cannot be resolved or is not a field
at SimpleTesting.main(SimpleTesting.java:7)
同樣,我們不能在陣列上使用字串 length()
方法。
public class SimpleTesting {
public static void main(String[] args) {
int[] intArray = {1, 2, 3};
System.out.println("The length of the string is: " + intArray.length());
}
}
輸出:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot invoke length() on the array type int[]
at SimpleTesting.main(SimpleTesting.java:7)
Java Collections size()
方法
size()
是 java.util.Collections
類的一個方法。Collections
類被許多不同的集合(或資料結構)使用,例如 ArrayList
、LinkedList
、HashSet
和 HashMap
。
size()
方法返回集合中當前存在的元素數。與陣列的 length
屬性不同,size()
方法返回的值不是常數,而是根據元素的數量而變化。
Java 中 Collection Framework
的所有集合都是動態分配的,因此元素的數量可能會有所不同。size()
方法用於跟蹤元素的數量。
在下面的程式碼中,很明顯,當我們建立一個沒有任何元素的新 ArrayList
時,size()
方法返回 0。
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
}
}
輸出:
The ArrayList is: []
The size of the ArrayList is: 0
但是這個值會隨著我們新增或刪除元素而改變。新增三個元素後,大小增加到 3。接下來,我們刪除了兩個元素,列表的大小變為 1。
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<Integer>();
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
list.add(20);
list.add(40);
list.add(60);
System.out.println("\nAfter adding three new elements:");
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
list.remove(0);
list.remove(1);
System.out.println("\nAfter removing two elements:");
System.out.println("The ArrayList is: " + list);
System.out.println("The size of the ArrayList is: " + list.size());
}
}
輸出:
The ArrayList is: []
The size of the ArrayList is: 0
After adding three new elements:
The ArrayList is: [20, 40, 60]
The size of the ArrayList is: 3
After removing two elements:
The ArrayList is: [40]
The size of the ArrayList is: 1
Java 中大小和長度的區別
儘管 size 和 length 有時可以在同一上下文中使用,但它們在 Java 中具有完全不同的含義。
陣列的 length
欄位用於表示陣列的最大容量。最大容量是指可以儲存在其中的最大元素數。此欄位不考慮陣列中存在的元素數量並保持不變。
字串的 length()
方法用於表示字串中出現的字元數。Collections Framework
的 size()
方法用於檢視該集合中當前存在的元素數量。Collections
具有動態大小,因此 size()
的返回值可能會有所不同。