解決 Java 中的 IllegalArgumentException
- Java 中 IllegalArgumentException 類的層次結構
- Java 中的執行時異常與編譯時異常
- 在 Java 中丟擲並解決 IllegalArgumentException
-
在 Java 中使用
Try
和Catch
使用 Scanner 確定 IllegalArgumentException
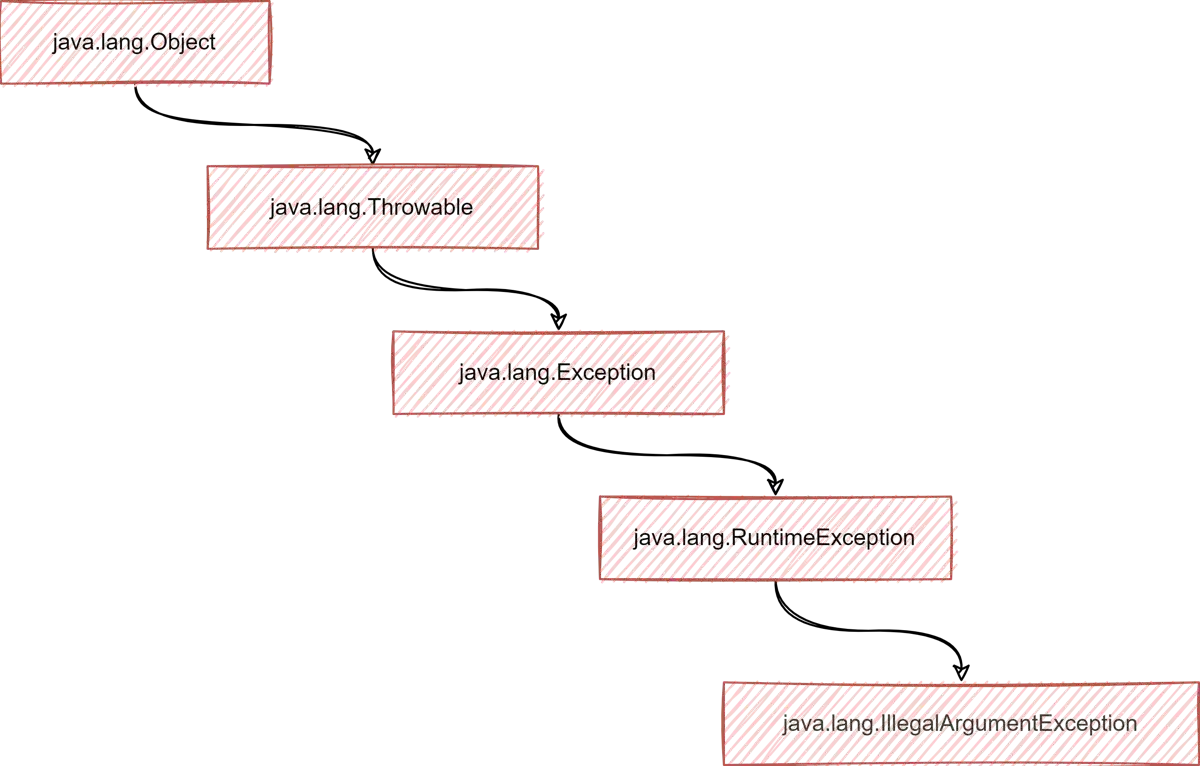
本教程將通過顯示 java.lang.object
類中的 java.lang.IllegalArgumentException
類對異常層次結構進行分類。之後,我們將執行三個 Java 編碼塊來演示非法引數異常。
最後,我們將展示如何擺脫它們並解釋如何自定義它們。
Java 中 IllegalArgumentException 類的層次結構
觸發此異常是因為我們可能給 Java 函式提供了無效引數。此異常擴充套件了執行時異常類,如下圖所示。
因此,Java 虛擬機器執行時可能會觸發一個異常。但是,要完全掌握它,請檢視以下 Java 類:
java.lang.object
:類層次結構的根是 Class 物件。java.lang.Throwable
:Throwable 類是所有 Java 錯誤和異常的超類。java.lang.Exception
:擴充套件 Throwable 類,該類識別實際應用程式可能想要捕獲的情況。java.lang.RuntimeException
:執行時異常是 Java 虛擬機器正常執行時可能產生的異常的超類。java.lang.IllegalArgumentException
:丟擲以通知向方法提供了非法或不適當的引數。
illegalargumentexception 的層次結構圖。
Java 中的執行時異常與編譯時異常
儘管大多數人都熟悉其中的區別,但許多人仍然不瞭解其簡單性。
下表說明了 Java 中執行時和編譯時錯誤/異常型別之間的區別。
編號 | 主題 | 執行時錯誤 | 編譯時錯誤 |
---|---|---|---|
1 | 異常 | 在 JVM 的正常執行過程中丟擲 | 當 Java 程式的語義規則被破壞時,JVM 會將這個錯誤作為一個異常拋給程式。 |
2 | 參考資料 | 類執行時異常 | 編譯器異常 |
3 | 解決方案 | 在執行和識別程式碼之後。 | 在程式碼的開發過程中。 |
在 Java 中丟擲並解決 IllegalArgumentException
下面的程式會丟擲一個非法引數異常,因為我們不能在陣列列表的初始容量中傳遞一個負值作為引數。
示例 1:
package com.IllegalAE.util;
import java.util.ArrayList;
import java.util.List;
public class Example1 {
public static void main(String[] args) {
List<String> demoList = new ArrayList<>(-1);
demoList.add("Red");
demoList.add("Blue");
demoList.add("Green");
System.out.println(demoList);
}
}
輸出:
如果我們沒有在初始列表容量中傳遞負值,例如:List<String> demoList = new ArrayList<>(1);
,我們會得到以下結果。
輸出:
[RED, GREEN, BLUE]
示例 2:
首先,你應該注意 int a
的值是 1000,而 int b
的值是 0。
package com.IllegalAE.util;
// class
public class Example2 {
// main function
public static void main(String[] args) {
// constructor
Demo2 examp2 = new Demo2();
// add 2 numbers
System.out.println(" The total of A and B is: " + examp2.addTwoNums(10, 5));
}
}
class Demo2 {
public int addTwoNums(int a, int b) {
// if the value of b is less than the value of a (trigger IllegalArgumentException with a
// message)
if (b < a) {
throw new IllegalArgumentException("B is not supposed to be less than " + a);
}
// otherwise, add two numbers
return a + b;
}
} // class
輸出:
主函式包含通過建構函式傳遞的物件。由於 b
的值小於 a
,所以觸發了非法引數異常。
如果我們像下面的程式碼一樣滑動 int a
和 b
的值,
System.out.println(" The total of A and B is: " + examp2.addTwoNums(0, 1000));
輸出:
The total of A and B is: 15
你還可以檢視下面的演示。
你可以設定非法引數條件以獲取正確的使用者輸入並控制你的邏輯流程。
同樣,Java 不允許我們通過傳遞非法引數來破壞方法。它是為了讓使用者編寫乾淨的程式碼並遵循標準的做法。
另一個原因是避免可能危及程式碼塊流的非法執行。
在 Java 中使用 Try
和 Catch
使用 Scanner 確定 IllegalArgumentException
編寫程式結構以使用 try
和 catch
異常以及 Java 掃描程式類來確定非法引數異常。
以下是你需要首先考慮的幾點:
-
理解
java.util.Scanner
類。Scanner mySc = new Scanner(System.in); int demo = mySc.nextInt();
請注意,上面的程式碼允許使用者閱讀 Java 的
System.in
。 -
try...catch
異常處理:try { // It allows us to define a block of code that will be tested for errors while it runs. } catch (Exception e) { // It enables us to create a code block that will be invoked if an error occurs in the try block. }
我們將能夠使用
try...catch
方法來利用非法引數異常,並以適合我們的方式對其進行修改。
例子:
package com.IllegalAE.util;
import java.util.Scanner;
public class IllegalArgumentExceptionJava {
public static void main(String[] args) {
String AddProduct = "+";
execute(AddProduct);
}
static void execute(String AddProduct) {
// allows user to read System.in
// tries code block for testing errors
try (Scanner nxtLINE = new Scanner(System.in)) {
// to ignore user specified case consideration
while (AddProduct.equalsIgnoreCase("+")) {
// The code inside try block will be tested for errors
try {
System.out.println("Please enter a new product ID: ");
int Productid = nxtLINE.nextInt();
if (Productid < 0 || Productid > 10)
throw new IllegalArgumentException();
System.out.println(
"You entered: " + Productid); // if try block has no error, this will print
}
// The code within the catch block will be run if the try block has any error
catch (IllegalArgumentException i) {
System.out.println(
"Invalid Entry Detected!. Do you want to try it again?"); // if try block had an
// error, this would print
AddProduct = nxtLINE.next();
if (AddProduct.equalsIgnoreCase("AddProduct"))
execute(AddProduct);
}
}
}
}
}
輸出:
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn