在 Java 中以 UTF-8 編碼字串
-
通過將字串轉換為位元組陣列並使用
new String()
將字串編碼為 UTF-8 -
使用
StandardCharsets.UTF_8.encode
和StandardCharsets.UTF_8.decode(byteBuffer)
將字串編碼為 UTF-8 -
使用
Files.readString()
將檔案中的字串編碼為 UTF-8
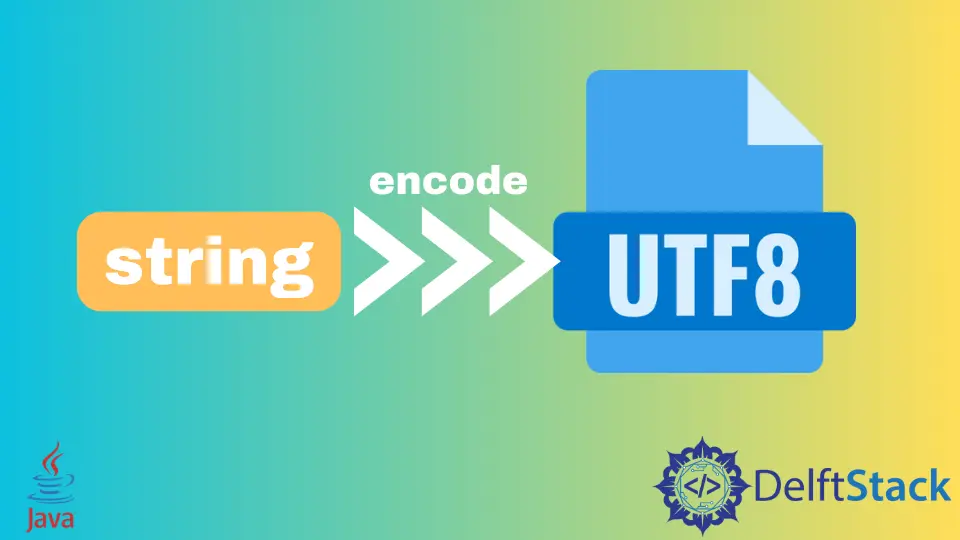
當我們使用字串時,我們需要使用編碼和解碼的概念,並且我們希望將該字串轉換為另一個字符集。
UTF-8 是 Unicode Transformation Format - 8 bit 的縮寫,是一種可變寬度標準,它為每個程式碼點或字元分配從 1 到 4 的不同位元組數。
下面我們看看如何將字串和檔案的內容編碼為 UTF-8 標準。
通過將字串轉換為位元組陣列並使用 new String()
將字串編碼為 UTF-8
我們首先在第一種方法中將字串轉換為位元組陣列,並使用 UTF-8 編碼建立字串。
我們建立一個包含日文字元的字串 japaneseString
。接下來,我們將字串轉換為 byte
陣列,因為我們無法將字串直接編碼為 UTF-8。japaneseString.getBytes()
返回 byte
型別的陣列。
現在我們使用 new String()
建立一個新字串並傳入兩個引數,第一個引數是 byte
陣列 japaneseBytesArray
,第二個引數是我們要使用的編碼格式。
我們使用 StandardCharsets
類來獲取編碼字符集並訪問 UTH_8
欄位。encodedString
包含一個用 UTF-8 編碼的字串。
import java.nio.charset.StandardCharsets;
public class JavaExample {
public static void main(String[] args) {
String japaneseString = "これはテキストです";
byte[] japaneseBytesArray = japaneseString.getBytes();
String encodedString = new String(japaneseBytesArray, StandardCharsets.UTF_8);
System.out.println(encodedString);
}
}
輸出:
これはテキストです
使用 StandardCharsets.UTF_8.encode
和 StandardCharsets.UTF_8.decode(byteBuffer)
將字串編碼為 UTF-8
我們可以使用 StandardCharsets
類將字串編碼為指定的字符集,如 UTF-8。
我們建立一個 japaneseString
,然後呼叫 charsets
型別的 StandardCharsets.UTF_8
的 encode()
。在 encode()
方法中,我們傳遞 japaneseString
,返回一個 ByteBuffer
物件。
該字串目前是一個 ByteBuffer
的形式,所以我們呼叫 StandardCharsets.UTF_8
的 decode()
方法,它接受 ByteBuffer
物件作為引數,最後,我們將結果轉換為使用 toString()
的字串。
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
public class JavaExample {
public static void main(String[] args) {
String japaneseString = "これはテキストです";
ByteBuffer byteBuffer = StandardCharsets.UTF_8.encode(japaneseString);
String encodedString = StandardCharsets.UTF_8.decode(byteBuffer).toString();
System.out.println(encodedString);
}
}
輸出:
これはテキストです
使用 Files.readString()
將檔案中的字串編碼為 UTF-8
在最後一個示例中,我們不是將單個字串編碼為 UTF-8 格式,而是讀取一個檔案並對檔案中的所有字串進行編碼。
首先,我們建立一個文字檔案並新增一些文字以使用 UTF-8 標準進行編碼。要獲取檔案的路徑,我們使用 Paths.get()
並將檔案的路徑作為返回 Path
物件的引數傳入。
我們呼叫 Files
類的 readString()
方法,它接受兩個引數,第一個引數是 Path
物件,第二個引數是我們使用 StandardCharsets.UTF_8
訪問的字符集。
我們得到編碼字串 readString
並將其列印在輸出中。
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class JavaExample {
public static void main(String[] args) {
try {
Path path = Paths.get(
"C:\\Users\\User1\\IdeaProjects\\Java Examples\\src\\main\\java\\example_file.txt");
String readString = Files.readString(path, StandardCharsets.UTF_8);
System.out.println(readString);
} catch (IOException e) {
e.printStackTrace();
}
}
}
輸出:
これはテキストです
Tämä on tekstiä
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn