在 Java 中轉換字串為輸入流
-
在 Java 中使用
ByteArrayInputStream()
把一個字串轉換為InputStream
-
使用
StringReader
和ReaderInputStream
將字串轉換為InputStream
-
使用
org.apache.commons.io.IOUtils
將字串轉換為InputStream
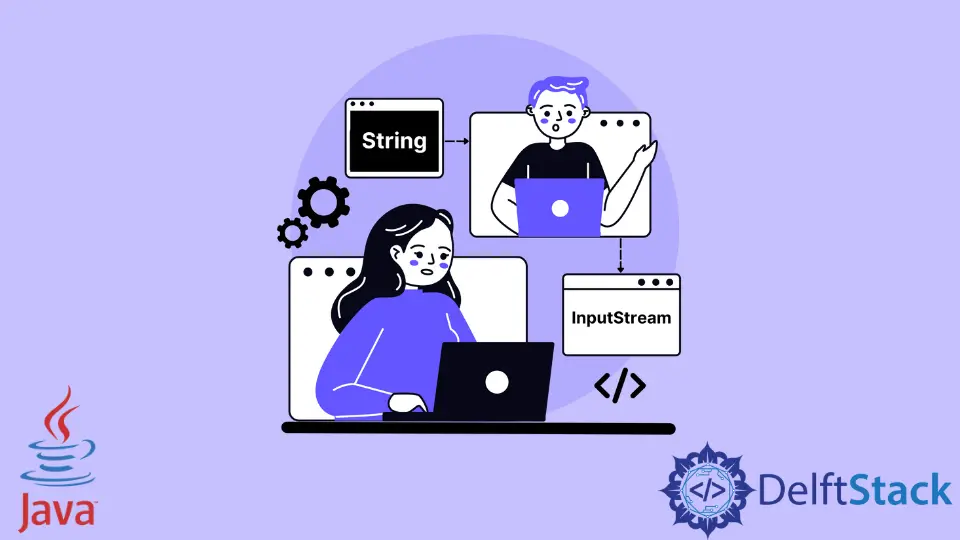
我們將討論如何在 Java 中使用幾種方法將一個字串轉換為一個 InputStream
。字串是一組字元,而 InputStream 是一組位元組。讓我們看看如何在 Java 中把字串轉換為 InputStream
。
在 Java 中使用 ByteArrayInputStream()
把一個字串轉換為 InputStream
Java 的 Input/Output 包中有一個類 ByteArrayInputStream
,可以將位元組陣列讀取為 InputStream
。首先,我們使用 getBytes()
從 exampleString
中獲取字符集為 UTF_8
的位元組,然後傳遞給 ByteArrayInputStream
。
為了檢查我們的目標是否成功,我們可以使用 read()
讀取 inputStream
,並將每個位元組轉換為 char
。這將返回我們的原始字串。
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream inputStream =
new ByteArrayInputStream(exampleString.getBytes(StandardCharsets.UTF_8));
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
輸出:
This is a sample string
使用 StringReader
和 ReaderInputStream
將字串轉換為 InputStream
將字串轉換為 InputStream
的第二種技術使用兩個方法,即 StringReader
和 ReaderInputStream
。前者用於讀取字串並將其包入 reader
中,而後者則需要兩個引數,一個 reader
和 charsets。最後,我們得到 InputStream
。
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.input.ReaderInputStream;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
StringReader stringReader = new StringReader(exampleString);
InputStream inputStream = new ReaderInputStream(stringReader, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
輸出:
This is a sample string
使用 org.apache.commons.io.IOUtils
將字串轉換為 InputStream
我們還可以使用 Apache Commons 庫來使我們的任務變得更簡單。這個 Apache Commons 庫的 IOUtls
類有一個 toInputStream()
方法,它接受一個字串和要使用的 charset
。這個方法是最簡單的,因為我們只需要呼叫一個方法就可以將 Java 字串轉換為 InputStream
。
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.IOUtils;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream is = IOUtils.toInputStream(exampleString, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = is.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
輸出:
This is a sample string
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn