在 Java 中將 Stream 元素轉換為對映
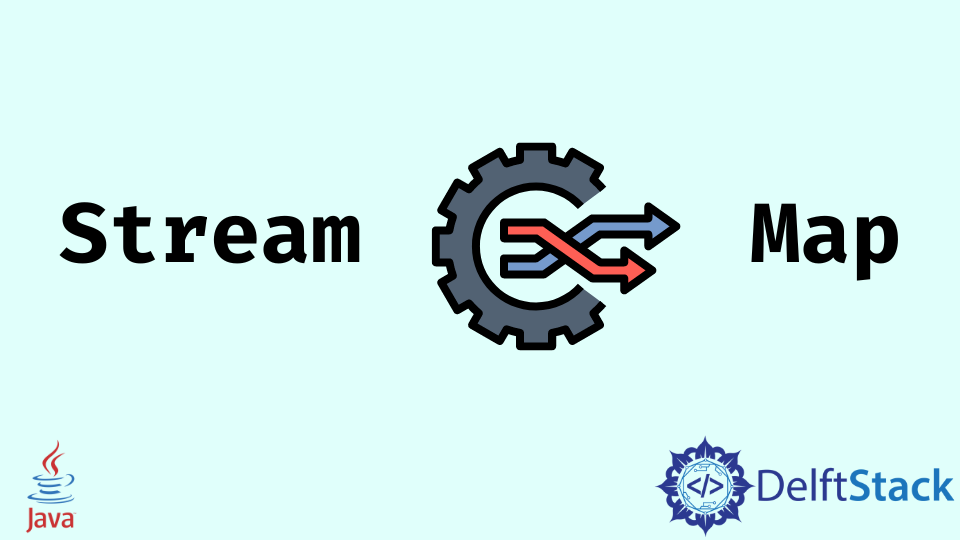
我們將討論 Java Streams
的實際用途以及如何將流元素轉換為對映元素。
要理解本文,你必須對 Java 8 有基本的瞭解,尤其是 lambda 表示式和 Stream
API。但是,如果你是新手,請不要擔心;我們將解釋一切。
Java 中要對映的字串流
你一定想知道字串本質上是一個元素列表,而不是鍵或值的列表,不是嗎?要將字串流轉換為對映,你必須瞭解以下方法的基礎知識。
Collectors.toMap()
- 它執行不同的功能歸約操作,例如將元素收集到集合中。toMap
- 它返回一個Collector
,它將元素收集到一個帶有鍵和值的Map
中。
Map<String, String> GMS =
stream.collect(Collectors.toMap(k->k[0],k-> k[1]));
知道了?我們將指定適當的對映函式來定義如何從流元素中檢索鍵和值。
Example1.java
的實現:
package delftstackStreamToMapJava;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Example1
{
// Method to get stream of `String[]`
private static Stream<String[]> MapStringsStream()
{
return Stream.of(new String[][] {
{"Sky", "Earth"},
{"Fire", "Water"},
{"White", "Black"},
{"Ocean", "Deep"},
{"Life", "Death"},
{"Love", "Fear"}
});
}
// Program to convert the stream to a map in Java 8 and above
public static void main(String[] args)
{
// get a stream of `String[]`
Stream<String[]> stream = MapStringsStream();
// construct a new map from the stream
Map<String, String> GMS =
stream.collect(Collectors.toMap(k->k[0],k-> k[1]));
System.out.println(GMS);
}
}
輸出:
{Sky=Earth, White=Black, Love=Fear, Fire=Water, Life=Death, Ocean=Deep}
從 Java 中的 Stream 物件建立 Map
該程式是使用 Collectors.toMap()
方法的另一個簡單演示。
此外,它還使用[引用函式/lambda 表示式]。因此,我們建議你檢查幾分鐘。
另外,如果你以前沒有使用過 [Function.identity()
],請閱讀它。
-
檢視我們的三個流:
``java
ListL1 = Arrays.asList(1.1,2.3)。
ListL2 = Arrays.asList(“A”, “B”, “C”);
ListL3 = Arrays.asList(10,20,30); 我們將三個陣列列表定義為具有不同資料型別的流。
-
為我們的主要對映方法新增更多內容:
2.1 從Stream
物件生成Map
需要一個鍵和值對映器。
2.2 在這種情況下,Stream
是我們的陣列列表,然後將其傳遞給Collectors
進行歸約,同時藉助.toMap
對映到鍵中。Map<Double, Object> printL1 = L1.stream() .collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1)); System.out.println("Stream of Double to Map: " + printL1);
Example2.java
的實現:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Example2 {
public static void main(String[] args) {
// Stream of Integers
List<Double> L1 = Arrays.asList(1.1, 2.3);
List<String> L2 = Arrays.asList("A", "B", "C");
List<Integer> L3 = Arrays.asList(10, 20, 30);
Map<Double, Object> printL1 = L1.stream()
.collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of Double to Map: " + printL1);
Map<String, Object> printL2 = L2.stream()
.collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of String to Map: " + printL2);
Map<Integer, Object> printL3 = L3.stream()
.collect(Collectors.toMap(Function.identity(), String::valueOf, (k1, k2) -> k1));
System.out.println("Stream of Integers to Map: " + printL3);
}
}
輸出:
Stream of Double to Map: {2.3=2.3, 1.1=1.1}
Stream of String to Map: {A=A, B=B, C=C}
Stream of Integers to Map: {20=20, 10=10, 30=30}
在 Java 中將 Stream 轉換為 Map 時確定值的長度
以下程式碼塊將字串轉換為 Map
,其中鍵表示字串值,值表示每個單詞的長度。這是我們的最後一個示例,儘管這屬於對映基本示例的基本流的類別。
解釋:
Collectors.toMap
收集器接受兩個 lambda 函式作為引數。
stream-> stream
- 確定Stream
值並將其作為對映的鍵返回。stream-> stream.length
- 找到當前流值,找到它的長度並將其返回給給定鍵的對映。
Example3.java
的實現:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.Map;
import java.util.stream.Collectors;
public class Example3 {
public static Map<String, Integer> toMap(String str) {
Map<String, Integer> elementL = Arrays.stream(str.split("/"))
.collect(Collectors.toMap(stream -> stream, stream -> stream.length()));
return elementL;
}
public static void main(String[] args) {
String stream = "We/Will/Convert/Stream/Elements/To/Map";
System.out.println(toMap(stream));
}
}
輸出:
{Convert=7, Stream=6, Will=4, To=2, Elements=8, Map=3, We=2}
在 Java 中為唯一的產品鍵將流轉換為對映
我們將轉換整數流 pro_id
和字串的 productname
。不過,不要混淆!
這是流集合對映值的又一個實現。
它是順序的,這裡的鍵是唯一的 pro_id
。整個程式除概念外與示例二相同。
這是使用流對映更現實的情況。它還將使你瞭解可以構建一個從陣列物件中提取唯一值和鍵的自定義程式有多少種方法。
Products.java
的實現:
package delftstackStreamToMapJava;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Products {
private Integer pro_id;
private String productname;
public Products(Integer pro_id, String productname) {
this.pro_id = pro_id;
this.productname = productname;
}
public Integer extractpro_id() {
return pro_id;
}
public String getproductname() {
return productname;
}
public String toString() {
return "[productid = " + this.extractpro_id() + ", productname = " + this.getproductname() + "]";
}
public static void main(String args[]) {
List<Products> listofproducts = Arrays.asList(new Products(1, "Lamda 2.0 "), new Products(2, "Gerrio 3A ultra"),
new Products(3, "Maxia Pro"), new Products(4, "Lemna A32"), new Products(5, "Xoxo Pro"));
Map<Integer, Products> map = listofproducts.stream()
.collect(Collectors.toMap(Products::extractpro_id, Function.identity()));
map.forEach((key, value) -> {
System.out.println(value);
});
}
}
輸出:
[productid = 1, productname = Lamda 2.0 ]
[productid = 2, productname = Gerrio 3A ultra]
[productid = 3, productname = Maxia Pro]
[productid = 4, productname = Lemna A32]
[productid = 5, productname = Xoxo Pro]
IllegalArgumentException
。Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn