如何在 Java 中從字串中刪除字元
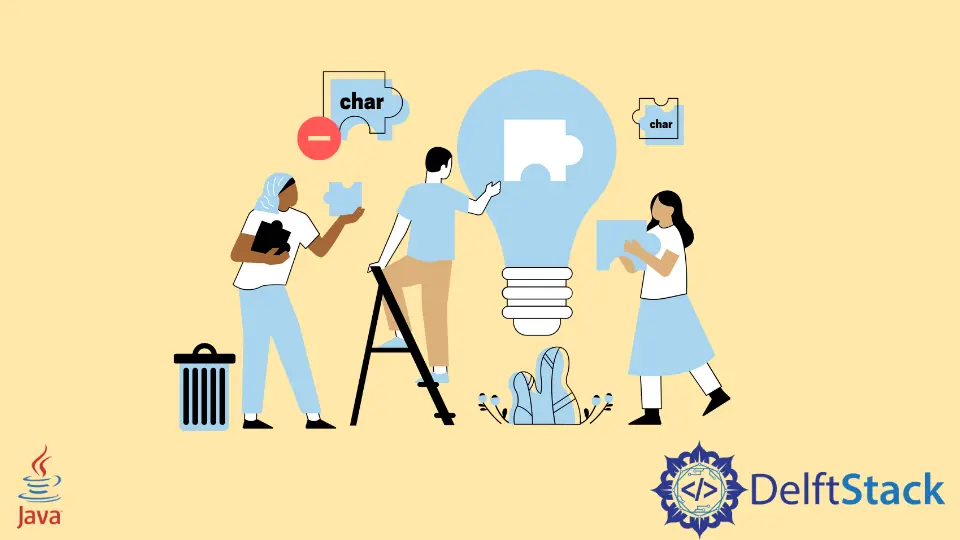
本教程文章將介紹如何在 Java 中從字串中刪除一個字元。
有幾個內建的函式可以從字串中刪除特定的字元,如下所示。
replace
函式deleteCharAt
函式substring
函式
在 Java 中使用 replace
函式從字串中刪除字元
在 Java 中,replace
函式可以用來從字串中刪除特定的字元。
replace
函式有兩個引數,第一個引數是要刪除的字元,第二個引數是空字串。
replace
函式用空字串替換字元,結果是刪除了與空字串一起傳遞的特定字元。
在 Java 中使用 replace
函式從字串中刪除一個字元的示例程式碼如下。
public class RemoveCharacter
{
public static void main(String[] args)
{
String MyString = "Hello World";
System.out.println("The string before removing character: " + MyString);
MyString = MyString.replace(" ", "");
System.out.println("The string after removing character: " + MyString);
}
}
在上面這段程式碼中,我們刪除了 Hello
和 World
之間的空格。我們在空字串的同時傳遞一個空格,然後用空字串代替空格,或者換句話說,從 Hello World
字串中刪除了空格。
程式碼的輸出如下。
The string before removing character : Hello World The string after removing character : HelloWorld
在 Java 中使用 deleteCharAt
方法從字串中刪除字元
deleteCharAt
方法是 StringBuilder
類的一個成員方法,在 Java 中也可以用來從一個字串中刪除一個字元。要從一個字串中刪除一個特定的字元,如果我們要使用 deleteCharAt
方法刪除該字元,我們必須知道該字元的位置。
deleteCharAt()
方法將我們要從字串中刪除的那個特定字元的位置。所以我們在使用 deleteCharAt
方法從字串中刪除該特定字元時,需要知道該特定字元的位置。
在 Java 中使用 deleteCharAt
方法從字串中刪除一個字元的示例程式碼如下。
public class RemoveCharacter
{
public static void main(String[] args)
{
StringBuilder MyString = new StringBuilder("Hello World");
System.out.println("The string before removing character: " + MyString);
MyString = MyString.deleteCharAt(5);
System.out.println("The string after removing character: " + MyString);
}
}
在上面的程式碼中,我們刪除了 Hello
和 World
之間的空格。我們傳遞了空格的位置,也就是 5,在字串 Hello World
中,因為在 Java 中,索引從 0 開始。
程式碼的輸出如下。
The string before removing character: Hello World
The string after removing character: HelloWorld
在 Java 中使用 substring
方法從字串中刪除一個字元
substring
方法也可以用於在 Java 中從字串中刪除一個字元。要使用 substring
方法刪除一個特定的字元,我們必須傳遞起始位置和刪除字元之前的位置。之後,我們從字串中我們的字元所在的位置開始連線字串。
substring
方法根據起始和結束索引分割字串,然後通過覆蓋我們要從字串中刪除的字元來連線同一個字串。
在 Java 中使用 substring
方法從字串中刪除一個字元的示例程式碼如下。
public class RemoveCharacter
{
public static void main(String[] args)
{
String MyString = "Hello World";
int Position = 5;
System.out.println("The string before removing character: " + MyString);
MyString = MyString.substring(0,Position) + MyString.substring(Position+1);
System.out.println("The string after removing character: " + MyString);
}
在上面這段程式碼中,我們刪除了 Hello
和 World
之間的空格。我們知道空格在一個變數中的位置是 5,我們使用 substring
方法將 Hello World
從第 0 位分割到第 5 位,並從第 6 位開始連線字串的其他部分。通過這種方法,我們去掉了 Hello World
中的空白。
程式碼的輸出如下。
The string before removing character: Hello World
The string after removing character: HelloWorld
相關文章 - Java String
- 如何在 Java 中以十六進位制字串轉換位元組陣列
- 如何在 Java 中執行字串到字串陣列的轉換
- 如何將 Java 字串轉換為位元組
- 如何從 Java 中的字串中刪除子字串
- 用 Java 生成隨機字串
- Java 中的交換方法