Java 中的屬性檔案
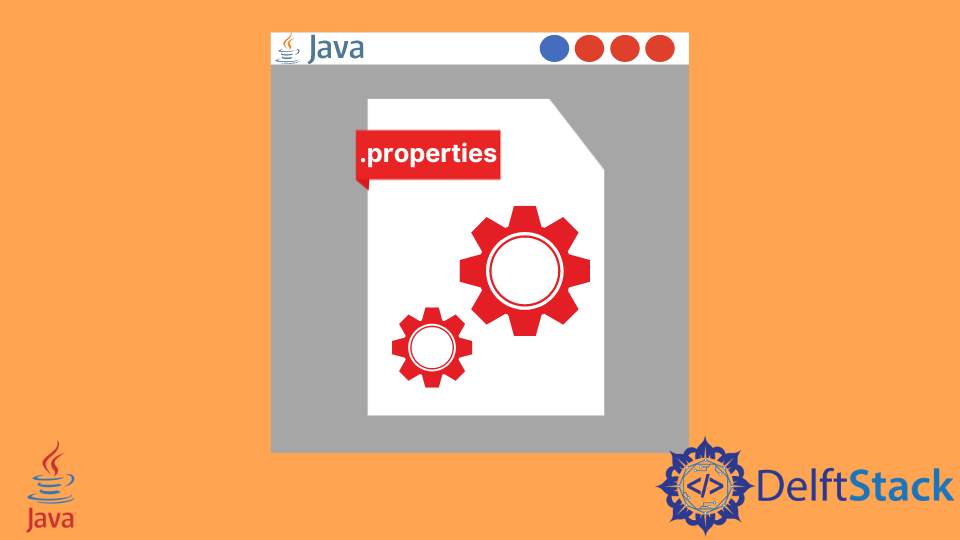
Java 中的屬性是我們需要在 Java 應用程式中使用的配置值。
屬性檔案是在鍵值對中包含這些值的檔案。這種型別的檔案通常具有 .properties
副檔名。
在 Java 屬性檔案中建立和設定值
在本教程的第一部分,我們編寫了一個程式來建立一個屬性檔案,然後將屬性設定為鍵值對。
Java 為我們提供了 java.util
包的 Properties
類,其中包含幾個方法來執行設定或刪除屬性、列出所有屬性等操作。
在我們繼續設定屬性之前,我們需要從它所在的目錄中獲取檔案屬性,如果它不存在,我們建立一個新檔案。我們建立一個 FileOutputStream()
物件,並在其建構函式中傳遞屬性檔案的路徑,該建構函式返回一個 OutputStream
例項。
我們使用 new Properties()
建立一個 Properties
物件並呼叫 setProperty()
方法來設定檔案中的屬性值。setProperty()
方法有兩個引數,第一個是鍵,第二個是值。
在示例中,我們設定了三個屬性,最後將屬性儲存在檔案中,我們呼叫 store()
方法將 outputStream
和可選註釋作為引數傳遞。應該建立屬性檔案並在其中插入屬性。
import java.io.*;
import java.util.Properties;
public class JavaExample {
public static void main(String[] args) {
try {
OutputStream outputStream = new FileOutputStream("myConfigs.properties");
Properties properties = new Properties();
properties.setProperty("version", "1.1");
properties.setProperty("projectName", "PropertiesFileExample");
properties.setProperty("date", "2021/12/27");
properties.store(outputStream, "Properties are stored here");
System.out.println(properties);
} catch (IOException e) {
e.printStackTrace();
}
}
}
輸出:
{date=2021/12/27, projectName=PropertiesFileExample, version=1.1}
myConfigs.properties
檔案:
#Properties are stored here
#Mon Dec 27 16:52:38 IST 2021
date=2021/12/27
projectName=PropertiesFileExample
version=1.1
從 Java 屬性檔案中獲取屬性
本節使用 Properties
類及其方法從屬性檔案中提供特定屬性。與前面的示例不同,我們使用 InputStream
而不是 OutputStream
,因為我們在此程式中讀取檔案。
我們建立一個 Properties
類的物件,呼叫 load()
方法,並將 InputStream
物件作為其引數傳遞。載入檔案後,我們呼叫 getProperty()
函式並傳遞我們要獲取的值的鍵。
我們得到值作為我們在輸出中列印的結果。
import java.io.*;
import java.util.Properties;
public class JavaExample {
public static void main(String[] args) {
try {
InputStream inputStream = new FileInputStream("myConfigs.properties");
Properties properties = new Properties();
properties.load(inputStream);
System.out.println(properties.getProperty("date"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
輸出:
2021/12/27
從 Java 屬性檔案中獲取所有鍵和值
list()
是 Properties
類的函式,用於將屬性列印到輸出流。我們在程式中讀取屬性檔案,建立一個 Properties
物件,並使用 load()
函式載入檔案。
要列印屬性,我們呼叫 list()
方法並將 System.out
作為引數傳遞,該引數是在控制檯上列印的輸出流。
import java.io.*;
import java.util.Properties;
public class JavaExample {
public static void main(String[] args) {
try {
InputStream inputStream = new FileInputStream("myConfigs.properties");
Properties properties = new Properties();
properties.load(inputStream);
properties.list(System.out);
} catch (IOException e) {
e.printStackTrace();
}
}
}
輸出:
-- listing properties --
date=2021/12/27
projectName=PropertiesFileExample
version=1.1
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn