Java 中的冪運算
Mohammad Irfan
2023年1月30日
2020年12月19日
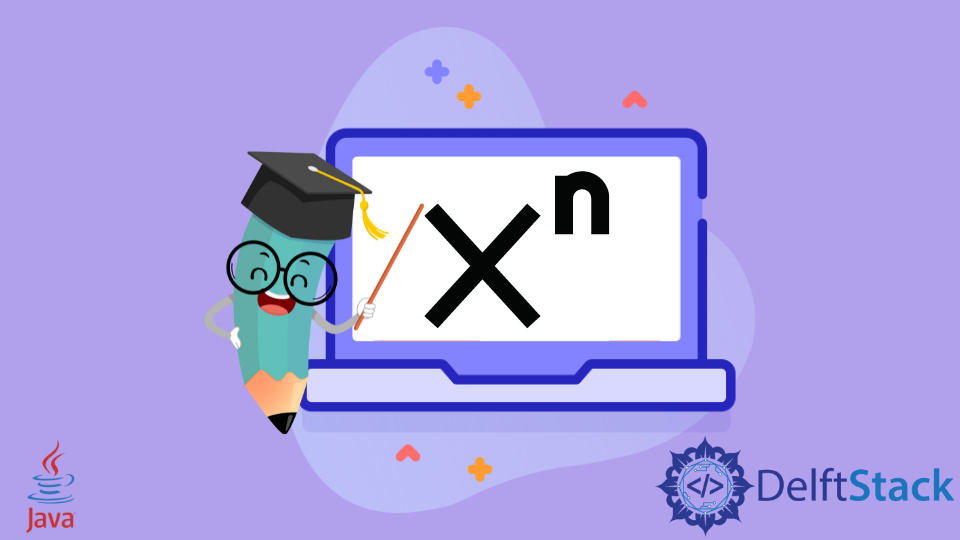
本教程介紹瞭如何在 Java 中進行冪操作,並列舉了一些示例程式碼來理解這個話題。
要在 Java 中做冪運算,我們可以使用 Math
類的 pow()
方法,或者使用迴圈或遞迴技術的自定義程式碼。讓我們看看一些例子。
在 Java 中使用 pow()
方法做冪運算
pow()
方法屬於 Java 中的 Math
類,用於生成給定數字的冪。這是一種簡單明瞭的方法,因為 pow()
是一個內建的方法,減少了編寫自定義程式碼的工作量。請看下面的例子。
public class SimpleTesting{
public static void main(String[] args) {
double a = 20;
double power = 2;
double result = Math.pow(a, power);
System.out.println(a+" power of "+power + " = "+result);
}
}
輸出:
20.0 power of 2.0 = 400.0
使用 Java 中的 while
迴圈做冪運算
如果你不想使用內建的 pow()
方法,那就使用下面的自定義程式碼。我們在這段程式碼裡面使用 while
迴圈來生成一個數字的冪。請看下面的例子。
public class SimpleTesting{
public static void main(String[] args) {
double a = 20;
double power = 2;
double result = 1;
double temp = power;
while (temp != 0)
{
result *= a;
--temp;
}
System.out.println(a+" power of "+power + " = "+result);
}
}
輸出:
20.0 power of 2.0 = 400.0
使用 Java 中的 for
迴圈做冪運算
如果你不想使用內建的 pow()
方法,可以使用下面的自定義程式碼。我們在這段程式碼裡面使用 for
迴圈來生成一個數字的冪。請看下面的例子。
public class SimpleTesting{
public static void main(String[] args) {
double a = 20;
double power = 2;
double result = 1;
double temp = power;
for (;temp != 0; --temp)
{
result *= a;
}
System.out.println(a+" power of "+power + " = "+result);
}
}
輸出:
20.0 power of 2.0 = 400.0
使用 Java 中的遞迴做冪運算
這是另一種方法,我們可以在 Java 中使用遞迴做冪運算。遞迴是一種函式重複呼叫自身直到滿足基本條件的技術。在這裡,我們建立一個遞迴方法,pow()
。請看下面的例子。
public class SimpleTesting{
static double result = 1;
static void pow(double n,double p)
{
if(p<=0)
{
return;
}
else if(n==0 && p>=1)
{
result=0;
return;
}
else
result=result*n;
pow(n,p-1);
}
public static void main(String[] args) {
double a = 20;
double power = 2;
pow(a,power);
System.out.println(a+" power of "+power + " = "+result);
}
}
輸出:
20.0 power of 2.0 = 400.0