在 Java 中實現鍵值對
-
在 Java 中使用
HashMap
實現鍵值對 -
在 Java 中使用
Map.Entry
實現鍵值對 -
使用 Java 中的
AbstractMap.SimpleEntry
類實現鍵值對 -
在 Java 中使用
Map.entry
實現鍵值對 -
在 Java 中使用
AbstractMap.SimpleImmutableEntry
實現鍵值對 -
在 Java 中使用
Maps.immutableEntry
實現鍵值對 -
使用 Java 中的
Properties
類實現鍵值對
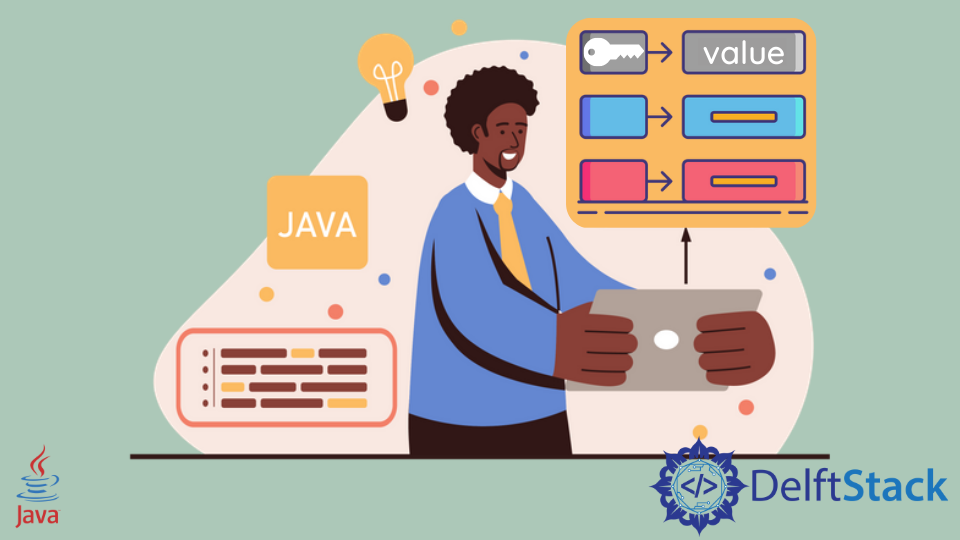
本教程介紹瞭如何在 Java 中實現鍵值對。
在 Java 中,為了處理鍵-值
對,使用了 Map
介面及其實現類。我們可以使用諸如 HashMap
和 TreeMap
之類的資料將資料儲存到鍵-值
對中。除了這些內建的類之外,我們還可以建立自己的類,該類可以容納鍵-值
對。
在這裡,我們將使用 HashMap
,使用者定義的類,AbstractMap
,Map.entry()
,AbstractMap.SimpleImmutableEntry()
和屬性
等。讓我們仔細看一下這些示例。
在 Java 中使用 HashMap
實現鍵值對
Collection
框架中的 Java Map 介面可用於將資料儲存到鍵-值
對中。在這裡,我們使用 HashMap
類來儲存字串型別的鍵值對。請參見下面的示例。
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting extends Thread{
public static void main(String[] args) {
Map<String, String> map = new HashMap<>();
map.put("name", "Rohan");
map.put("sname", "Kumar");
System.out.println(map);
}
}
輸出:
{sname=Kumar, name=Rohan}
在 Java 中使用 Map.Entry
實現鍵值對
在這裡,我們使用 Map.Entry
介面建立一個自定義類,該類將以鍵值對的形式儲存資料。我們建立了一個 Student
類,其中有兩個例項變數來儲存鍵和值對。我們還建立了 getters 和 setters 方法來為此類的每個例項設定值。請參見下面的示例。
import java.util.Map;
class Student<K, V> implements Map.Entry<K, V> {
private final K key;
private V value;
public Student(K key, V value) {
this.key = key;
this.value = value;
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
@Override
public V setValue(V value) {
V old = this.value;
this.value = value;
return old;
}
}
public class SimpleTesting extends Thread{
public static void main(String[] args) {
Student<String, String> student = new Student<>("name","Rohan");
String key = student.getKey();
String value = student.getValue();
System.out.println("{"+key+":"+value+"}");
}
}
輸出:
{name:Rohan}
使用 Java 中的 AbstractMap.SimpleEntry
類實現鍵值對
在這裡,我們使用 AbstractMap
類來實現鍵值對。getKey()
和 getValue()
方法分別用於獲取鍵和值。請參見以下示例。
import java.util.AbstractMap;
import java.util.Map;
public class SimpleTesting extends Thread{
public static void main(String[] args) {
Map.Entry<String,String> entry =
new AbstractMap.SimpleEntry<String, String>("name", "Rohan");
String key = entry.getKey();
String value = entry.getValue();
System.out.println("{"+key+":"+value+"}");
}
}
輸出:
{name:Rohan}
在 Java 中使用 Map.entry
實現鍵值對
我們可以使用 Map.entry
將資料儲存到鍵和值對中。在這裡,我們使用 Entry
介面及其方法 getKey()
和 getValue()
分別獲取鍵和值。請參見以下示例。
import java.util.Map;
import java.util.Map.Entry;
public class SimpleTesting extends Thread{
public static void main(String[] args) {
Entry<String, String> entry = Map.entry("name", "Rohan");
String key = entry.getKey();
String value = entry.getValue();
System.out.println("{"+key+":"+value+"}");
}
}
輸出:
{name:Rohan}
在 Java 中使用 AbstractMap.SimpleImmutableEntry
實現鍵值對
我們可以使用 SimpleImmutableEntry
來建立一組不可變的鍵值對。請參見以下示例。
import java.util.AbstractMap;
import java.util.Map.Entry;
public class SimpleTesting extends Thread{
public static void main(String[] args) {
Entry<String, String> entry = new AbstractMap.SimpleImmutableEntry<>("name","Rohan");
String key = entry.getKey();
String value = entry.getValue();
System.out.println("{"+key+":"+value+"}");
}
}
輸出:
{name:Rohan}
在 Java 中使用 Maps.immutableEntry
實現鍵值對
在這裡,我們使用 Map.immutableEntry
在 Java 中建立鍵值對。我們使用 getKey()
和 getValue()
方法分別獲取鍵和值。
import java.util.AbstractMap;
import java.util.Map;
import java.util.Map.Entry;
import com.google.common.collect.Maps;
public class MainClass extends Thread{
public static void main(String[] args) {
Map.Entry<String, String> entry = Maps.immutableEntry("name", "Rohan");
String key = entry.getKey();
String value = entry.getValue();
System.out.println("{"+key+":"+value+"}");
}
}
輸出:
{name:Rohan}
使用 Java 中的 Properties
類實現鍵值對
Java 集合的 Properties
類可用於將資料儲存為鍵值對。Properties
類的 getProperty()
方法返回與鍵關聯的值。請參見以下示例。
import java.util.Properties;
public class MainClass extends Thread{
public static void main(String[] args) {
Properties props = new Properties();
props.setProperty("name", "Rohan"); // (key, value)
String value = props.getProperty("name");
System.out.println("{name:"+value+"}");
}
}
輸出:
{name:Rohan}