Java 中的字串駐留
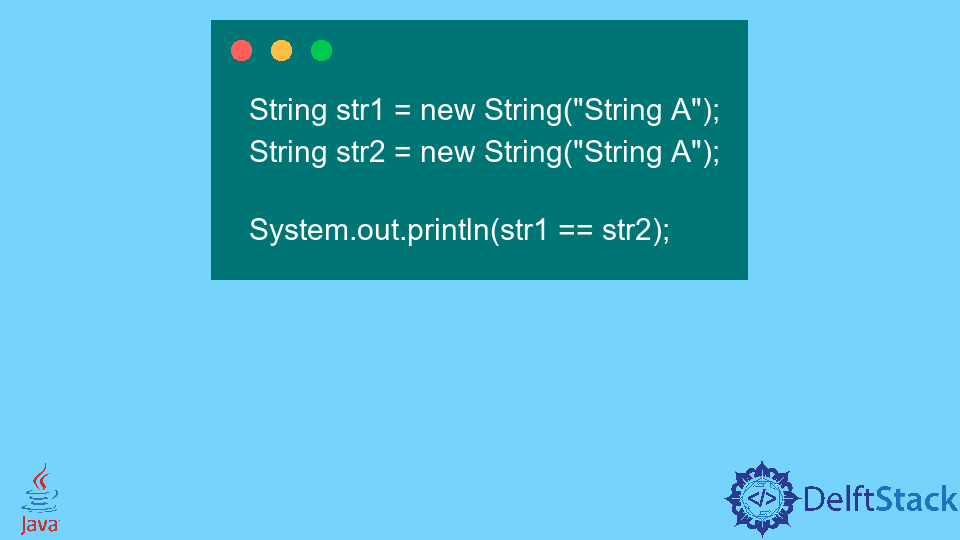
今天我們將討論 String 駐留或 String 類的 intern()
方法。在以下幾點中,我們將通過一個示例瞭解什麼是 String Interning 以及為什麼需要使用它。
什麼是 Java 中的字串駐留以及何時使用它
Java 是一種物件導向的程式語言。當我們建立任何物件或變數時,它會佔用一些記憶體,這意味著如果我們建立一百個 String
類的例項,它們將像堆一樣儲存在記憶體中的不同位置,這會消耗大量空間。
為了解決這個問題,Java 開發人員引入了字串常量池 (SCP) 的概念,其中包含共享相同記憶體池的物件。
SCP 包含具有相同內容的所有 String 物件,這使得獲取物件變得簡單且節省記憶體,因為我們不必查詢多個物件。我們只需要一個可以引用的物件。
為了更好地理解它,我們可以看一個簡單的例子:
String str1 = new String("String A");
String str2 = new String("String A");
System.out.println(str1 == str2);
輸出:
false
在上面的程式碼中,有 String
類 str1
和 str2
的兩個物件,但它們都包含相同的內容。如果我們使用 ==
運算子比較它們以檢查它們是否相等,輸出顯示 false
,使它們不相等。
發生這種情況是因為它們位於堆中的不同位置。除了是兩個物件之外,兩個物件都沒有顯著差異。
如果我們使用 String Interning 的概念,我們只建立一個物件讓我們假設它為 strObj
,現在它包含物件的內容。當我們要訪問物件時,我們可以引用這個物件並獲取內容。
在 Java 中使用字串駐留
在下面的示例中,我們看到了如何使用 intern()
來使用 String Interning 方法。我們建立了五個字串變數。
第一個字串變數 str
是使用 new String()
建立的,字串在建構函式中傳遞。接下來,我們使用 new
關鍵字建立另一個字串物件,但我們從 String()
建構函式呼叫 intern()
方法。
最後三個變數是字串文字,其中最後一個變數 str5
包含與所有其他字串變數不同的內容。請注意,我們沒有對最後三個變數使用任何 intern()
方法,因為字串文字已經放置在 SCP 中,這意味著駐留會自動應用於它們。
現在我們比較變數以檢查它們是否匹配。如果物件引用了確切的記憶體位置,我們使用返回 true
的 ==
運算子。由於我們沒有使用第一個字串 str1
呼叫 intern()
方法,它位於不同的記憶體位置,因此 str1 == str2
不會執行。
str2
在 SCP 中,因為我們用它呼叫了 intern()
,當我們將它與字串字面量 str3
進行比較時,它返回 true
並執行輸出。接下來,我們比較兩個具有相同內容的字串字面量,str3
和 str4
,它們在控制檯中輸出訊息。
public class JavaExample {
public static void main(String[] args) {
String str1 = new String("String A");
String str2 = new String("String A").intern();
String str3 = "String A";
String str4 = "String A";
String str5 = "String B";
if (str1 == str2) {
System.out.println("str1 and str2 are in the same SCP");
}
if (str1 == str3) {
System.out.println("str1 and str3 are in the same SCP");
}
if (str2 == str3) {
System.out.println("str2 and str3 are in the same SCP");
}
if (str3 == str4) {
System.out.println("str3 and str4 are in the same SCP");
}
if (str4 == str5) {
System.out.println("str3 and str4 are in the same SCP");
}
}
}
輸出:
str2 and str3 are in the same SCP
str3 and str4 are in the same SCP
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn