獲取 Java 中的物件型別
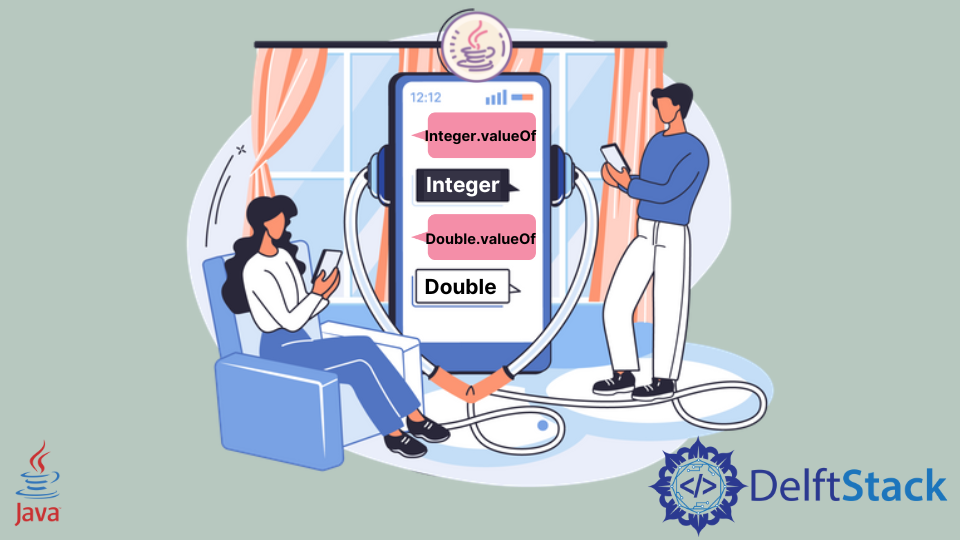
在本文中,我們將學習如何在 Java 中獲取物件的型別。當物件來自源時,檢查物件型別會很有幫助。這是我們無法驗證物件型別的地方,例如來自我們無權訪問的 API 或私有類。
在 Java 中使用 getClass()
獲取物件型別
在第一種方法中,我們檢查包裝類的 Object 型別,如 Integer
和 String
。我們有兩個物件,var1
和 var2
,用於檢查型別。我們將使用 Object
類的 getClass()
方法,Java 中所有物件的父類。
我們使用 if
條件檢查類。由於包裝類還包含一個返回型別的欄位類,我們可以檢查誰的型別與 var1
和 var2
匹配。下面,我們檢查三種型別的物件。
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = String.valueOf(var1);
if (var1.getClass() == Integer.class) {
System.out.println("var1 is an Integer");
} else if (var1.getClass() == String.class) {
System.out.println("var1 is a String");
} else if (var1.getClass() == Double.class) {
System.out.println("var1 is a Double");
}
if (var2.getClass() == Integer.class) {
System.out.println("var2 is an Integer");
} else if (var2.getClass() == String.class) {
System.out.println("var2 is a String");
} else if (var2.getClass() == Double.class) {
System.out.println("var2 is a Double");
}
}
}
輸出:
var1 is an Integer
var2 is a String
在 Java 中使用 instanceOf
獲取物件型別
在 Java 中獲取物件型別的另一種方法是使用 instanceOf
函式;如果物件的例項與給定的類匹配,則返回。在此示例中,我們使用以下三種型別檢查物件 var1
和 var2
:Integer
、String
和 Double
;如果任何一個條件滿足,我們就可以執行不同的程式碼。
因為 var1
是 Integer
型別,條件 var1 instanceOf Integer
將變為真,而 var2
是 Double
,因此,var2 instanceOf Double
將變為真。
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = Double.valueOf("10");
if (var1 instanceof Integer) {
System.out.println("var1 is an Integer");
} else if (var1 instanceof String) {
System.out.println("var1 is a String");
} else if (var1 instanceof Double) {
System.out.println("var1 is a Double");
}
if (var2 instanceof Integer) {
System.out.println("var2 is an Integer");
} else if (var2 instanceof String) {
System.out.println("var2 is a String");
} else if (var2 instanceof Double) {
System.out.println("var2 is a Double");
}
}
}
輸出:
var1 is an Integer
var2 is a Double
在 Java 中獲取手動建立的類物件的型別
我們檢查了包裝類的型別,但是在這個例子中,我們得到了三個手動建立的類的三個物件的型別。我們建立了三個類:ObjectType2
、ObjectType3
和 ObjectType4
。
ObjectType3
繼承了 ObjectType4
,ObjectType2
繼承了 ObjectType3
。現在我們想知道所有這些類的物件型別。我們有三個物件,obj
、obj2
和 obj3
;我們使用了我們在上面的例子中討論的 getClass()
和 instanceOf
兩種方法。
但是,obj2
的型別之間存在差異。obj2
變數返回型別 ObjectType4
,而其類是 ObjectType3
。發生這種情況是因為我們繼承了 ObjectType3
中的 ObjectType4
類,而 instanceOf
檢查所有類和子類。obj
返回 ObjectType3
,因為 getClass()
函式只檢查直接類。
public class ObjectType {
public static void main(String[] args) {
Object obj = new ObjectType2();
Object obj2 = new ObjectType3();
Object obj3 = new ObjectType4();
if (obj.getClass() == ObjectType4.class) {
System.out.println("obj is of type ObjectType4");
} else if (obj.getClass() == ObjectType3.class) {
System.out.println("obj is of type ObjectType3");
} else if (obj.getClass() == ObjectType2.class) {
System.out.println("obj is of type ObjectType2");
}
if (obj2 instanceof ObjectType4) {
System.out.println("obj2 is an instance of ObjectType4");
} else if (obj2 instanceof ObjectType3) {
System.out.println("obj2 is an instance of ObjectType3");
} else if (obj2 instanceof ObjectType2) {
System.out.println("obj2 is an instance of ObjectType2");
}
if (obj3 instanceof ObjectType4) {
System.out.println("obj3 is an instance of ObjectType4");
} else if (obj3 instanceof ObjectType3) {
System.out.println("obj3 is an instance of ObjectType3");
} else if (obj3 instanceof ObjectType2) {
System.out.println("obj3 is an instance of ObjectType2");
}
}
}
class ObjectType2 extends ObjectType3 {
int getAValue3() {
System.out.println(getAValue2());
a = 300;
return a;
}
}
class ObjectType3 extends ObjectType4 {
int getAValue2() {
System.out.println(getAValue1());
a = 200;
return a;
}
}
class ObjectType4 {
int a = 50;
int getAValue1() {
a = 100;
return a;
}
}
輸出:
obj is of type ObjectType2
obj2 is an instance of ObjectType4
obj3 is an instance of ObjectType4
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn