在 Java 中建立併發集
Sheeraz Gul
2023年1月30日
2022年4月26日
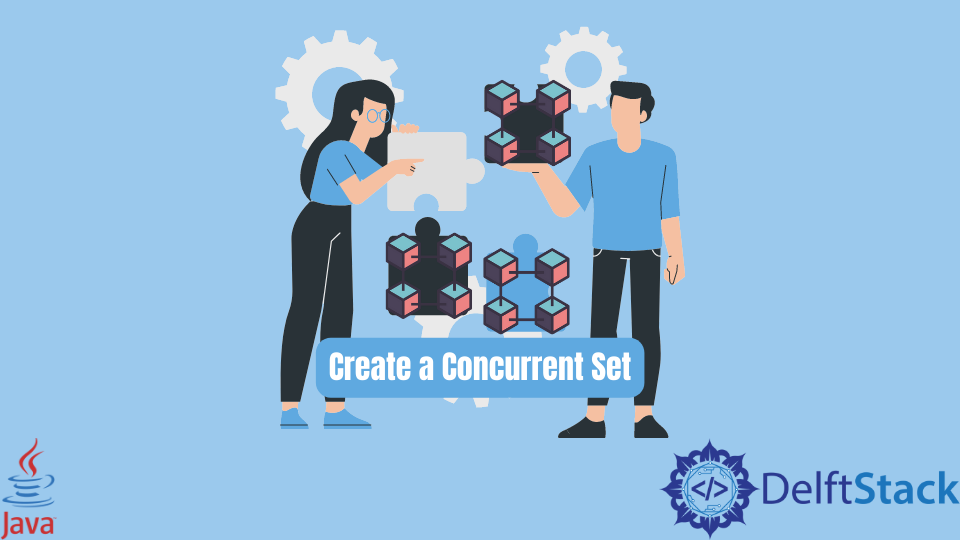
在 JDK 8 之前,Java 不支援併發雜湊集。現在,ConcurrentHashMap
用於在 Java 中建立併發集。
ConcurrentHashMap
具有函式 KeySet()
和 newKeySet()
在 Java 中建立併發雜湊集。
本教程演示瞭如何在 Java 中建立併發雜湊集。
在 Java 中使用 ConcurrentHashMap
和 KeySet()
函式建立併發集
使用 ConcurrentHashMap
和 KeySet()
我們可以在 Java 中建立一個併發集。
package delftstack;
import java.io.*;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
class ConcurrentSet {
public static void main (String[] args) {
// Creating a concurrent hash map
ConcurrentHashMap<String,Integer> demo_map = new ConcurrentHashMap<>();
demo_map.put("Delftstack",10);
demo_map.put("Jack",20);
demo_map.put("John", 30);
demo_map.put("Mike",40);
demo_map.put("Michelle", 50);
// use keySet() to create a set from the concurrent hashmap
Set keyset_conc_set = demo_map.keySet();
System.out.println("The concurrent set using keySet() function is : " + keyset_conc_set);
}
}
該程式碼從併發 HashMap 建立一組併發名稱。我們不能使用 add()
方法向集合中新增更多成員;它會丟擲一個錯誤。
輸出:
The concurrent set using keySet() function is : [Michelle, Mike, Delftstack, John, Jack]
在 Java 中使用 ConcurrentHashMap
和 newKeySet()
函式建立併發集
newKeySet()
用於建立一個可以稍後操作的併發集合,從集合中新增或刪除元素。
package delftstack;
import java.io.*;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
class ConcurrentSet {
public static void main (String[] args) {
// Create a concurrent set using concorrentHashMap and newkeyset()
Set <String> newKeySet_conc_set = ConcurrentHashMap.newKeySet();
newKeySet_conc_set.add("Mike");
newKeySet_conc_set.add("Michelle");
newKeySet_conc_set.add("John");
newKeySet_conc_set.add("Jack");
// Print the concurrent set
System.out.println("The concurrent set before adding the element: " + newKeySet_conc_set);
// Add new element
newKeySet_conc_set.add("Delftstack");
// Show the change
System.out.println("The concurrent set after adding the element: " + newKeySet_conc_set);
// Check any element using contains
if(newKeySet_conc_set.contains("Delftstack")){
System.out.println("Delftstack is a member of the set");
}
else{
System.out.println("Delftstack is not a member of the set");
}
// Remove any element from the concurrent set
newKeySet_conc_set.remove("Delftstack");
System.out.println("The concurrent set after removing the element: " + newKeySet_conc_set);
}
}
上面的程式碼從 ConcurrentHashMap
和 newKeySet
函式生成一個併發集,然後新增、刪除和檢查元素。
輸出:
The concurrent set before adding the element: [Michelle, Mike, John, Jack]
The concurrent set after adding the element: [Michelle, Mike, Delftstack, John, Jack]
Delftstack is a member of the set
The concurrent set after removing the element: [Michelle, Mike, John, Jack]
Author: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook