Java 中的 abs() 方法
Mehvish Ashiq
2023年10月12日
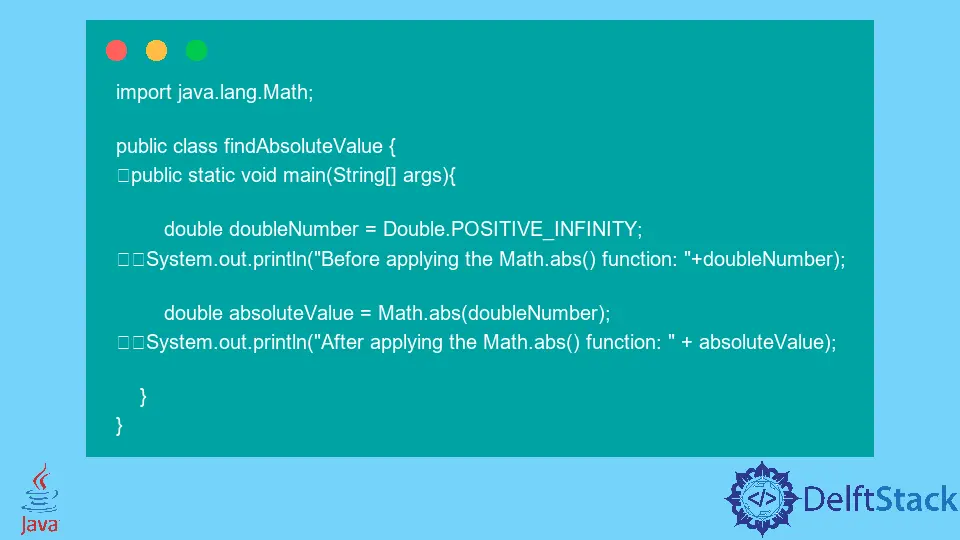
我們將學習 Java 中的 abs()
方法來查詢指定數字的絕對值。我們將通過編寫和練習各種程式碼示例來學習。
Java 中的絕對值是什麼
絕對值是指指定數字的非負值。例如,-4 的絕對值為 4。
我們使用 java.lang.Math
包的 abs()
方法。它只接受一個型別為 int
、double
、float
或 long
的引數並返回其非負(絕對)值。
以下是一些經驗法則,你必須記住要成為專家,同時找到給定數字的絕對值。
- 如果引數的資料型別是
float
或double
:
1.1abs()
返回正值;傳遞的引數是正數還是負數都沒有關係。
1.2 如果我們將Infinity
作為引數傳遞,它的結果是POSITIVE_INFINITY
。
1.3 如果它的引數是NaN
,它返回NaN
。
1.4 如果abs()
方法得到一個負零或正零,它返回一個正零。 - 如果引數的資料型別是
long
或int
:
2.1 如果Long.MIN_VALUE
或Integer.MIN_VALUE
的值等於abs()
方法的引數,輸出將是相同的,一個負值。
在 Java 中使用 abs()
方法查詢數字的絕對值
在本節中,我們將編寫不同的程式碼示例來練習上面給出的所有規則。
示例程式碼(當將負數作為引數傳遞時):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
int intNumber = -8;
System.out.println("Before applying the Math.abs() function: " + intNumber);
int absoluteValue = Math.abs(intNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
輸出:
Before applying the Math.abs() function: -8
After applying the Math.abs() function: 8
在 main
方法中,我們宣告並初始化一個變數以儲存一個值,該值進一步傳遞給 Math.abs()
函式以計算給定數字的絕對值(正值)。
我們在應用 Math.abs()
函式之前和之後列印一個數字的值。在接下來的示例中將遵循相同的過程,但變數名稱和型別將被更改。
示例程式碼(當一個正數作為引數傳遞時):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
int intNumber = 8;
System.out.println("Before applying the Math.abs() function: " + intNumber);
int absoluteValue = Math.abs(intNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
輸出:
Before applying the Math.abs() function: 8
After applying the Math.abs() function: 8
示例程式碼(當 infinity
作為引數傳遞時):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
double doubleNumber = Double.POSITIVE_INFINITY;
System.out.println("Before applying the Math.abs() function: " + doubleNumber);
double absoluteValue = Math.abs(doubleNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
輸出:
Before applying the Math.abs() function: Infinity
After applying the Math.abs() function: Infinity
示例程式碼(當 NaN
作為引數傳遞時):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
double doubleNumber = Double.NaN;
System.out.println("Before applying the Math.abs() function: " + doubleNumber);
double absoluteValue = Math.abs(doubleNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
輸出:
Before applying the Math.abs() function: NaN
After applying the Math.abs() function: NaN
示例程式碼(當正零或負零作為引數傳遞時):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
int number, absoluteValue;
number = -0;
System.out.println("Before applying the Math.abs() function: " + number);
absoluteValue = Math.abs(number);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
number = 0;
System.out.println("Before applying the Math.abs() function: " + number);
absoluteValue = Math.abs(number);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
輸出:
Before applying the Math.abs() function: 0
After applying the Math.abs() function: 0
Before applying the Math.abs() function: 0
After applying the Math.abs() function: 0
示例程式碼(當 Long.MIN_VALUE
或 Integer.MIN_VALUE
作為引數傳遞時):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
long longNumber = Long.MIN_VALUE;
System.out.println("Before applying the Math.abs() function: " + longNumber);
long longAbsVal = Math.abs(longNumber);
System.out.println("After applying the Math.abs() function: " + longAbsVal);
int intNumber = Integer.MIN_VALUE;
System.out.println("Before applying the Math.abs() function: " + intNumber);
int intAbsVal = Math.abs(intNumber);
System.out.println("After applying the Math.abs() function: " + intAbsVal);
}
}
輸出:
Before applying the Math.abs() function: -9223372036854775808
After applying the Math.abs() function: -9223372036854775808
Before applying the Math.abs() function: -2147483648
After applying the Math.abs() function: -2147483648
作者: Mehvish Ashiq