如何在 Java 中刪除字串中的最後一個字元
Mohammad Irfan
2023年1月30日
2020年10月15日
-
使用 Java 中的
substring()
方法從字串中刪除最後一個字元 -
在 Java 中使用
replaceFirst()
方法從字串中刪除最後一個字元 -
使用 Java 中的
removeEnd()
方法從字串中刪除最後一個字元 -
使用 Java 中的
chop()
方法從字串中刪除最後一個字元 -
使用 Java 中的
substring()
方法從字串中刪除最後一個字元 - 在 Java 8 或更高版本中刪除字串中的最後一個字元
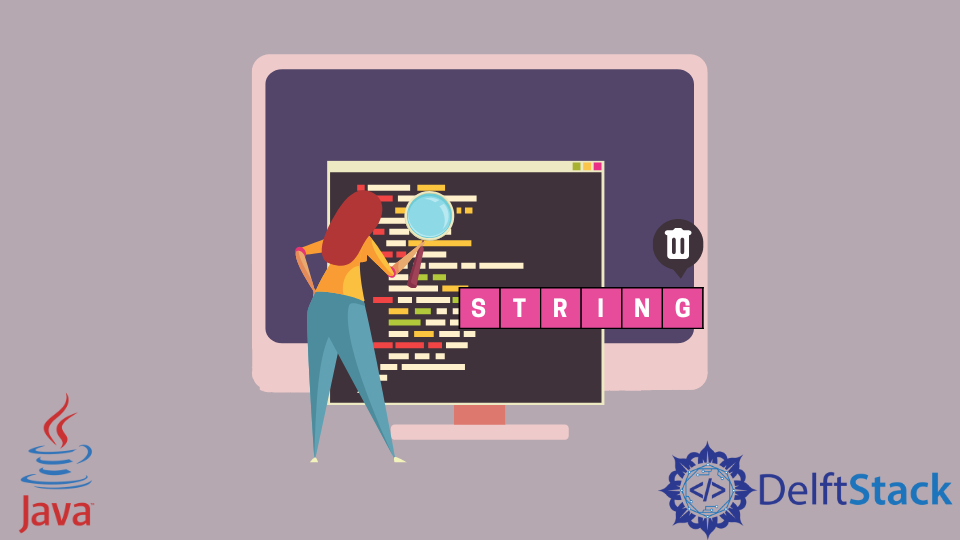
本教程介紹瞭如何在 Java 中刪除字串中的最後一個字元,還列舉了一些示例程式碼來理解這個主題。刪除最後一個字元的方法有好幾種,比如 substring()
方法、StringUtils
類等。讓我們來仔細看看這些例子。
使用 Java 中的 substring()
方法從字串中刪除最後一個字元
String
類的 substring()
方法用於從字串物件中獲取子字串。它需要兩個引數:起始索引和最後索引。在這裡,我們使用 substring()
方法在排除最後一個字元後得到一個字串。請看下面的例子。
public class SimpleTesting
{
public static void main(String args[])
{
String str = "removea";
str = str.substring(0, str.length()-1);
System.out.println(str);
}
}
輸出:
remove
在 Java 中使用 replaceFirst()
方法從字串中刪除最後一個字元
我們可以使用 replaceFirst()
方法從字串中刪除最後一個字元。它在替換了這個字元後返回一個字串。請看下面的例子。
public class SimpleTesting
{
public static void main(String args[])
{
String str = "removea";
str = str.replaceFirst(".$","");
System.out.println(str);
}
}
輸出:
remove
使用 Java 中的 removeEnd()
方法從字串中刪除最後一個字元
如果你正在使用 Apache commons lang
庫,最好使用 StringUtils
類,它提供 removeEnd()
方法來刪除字串的最後一個字元。請看下面的例子。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting
{
public static void main(String args[])
{
String str = "removea";
str = StringUtils.removeEnd(str, "a");
System.out.println(str);
}
}
輸出:
remove
使用 Java 中的 chop()
方法從字串中刪除最後一個字元
另一個方法,StringUtils
類的 chop()
,從指定的字串中刪除最後一個字元後返回一個字串。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting
{
public static void main(String args[])
{
String str = "removea";
str = StringUtils.chop(str);
System.out.println(str);
}
}
輸出:
remove
使用 Java 中的 substring()
方法從字串中刪除最後一個字元
StringUtils
類提供了 substring()
方法,該方法需要三個引數。第一個是一個字串,第二個是一個起始索引,第三個是字串的結束索引。它返回一個字串,也就是指定字串的子字串。請看下面的例子。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting
{
public static void main(String args[])
{
String str = "removea";
str = StringUtils.substring(str, 0, -1);
System.out.println(str);
}
}
輸出:
remove
在 Java 8 或更高版本中刪除字串中的最後一個字元
如果你使用的是更高版本的 Java,你可以使用 Optional
類來避免 null 異常,並採用函式方法從字串中刪除最後一個字元。
import java.util.Optional;
public class SimpleTesting
{
public static void main(String args[])
{
String str = "removea";
str = Optional.ofNullable(str)
.filter(s -> s.length() != 0)
.map(s -> s.substring(0, s.length() - 1))
.orElse(str);
System.out.println(str);
}
}
輸出:
remove