如何在 Java 中檢查一個字串是否是一個數字
Mohammad Irfan
2023年10月12日
- 在 Java 中檢查一個字串是否是數字
-
使用 Java 中的
Character
類檢查字串是否是數字 - 在 Java 中使用 Apache 庫檢查一個字串是否是一個數字
-
使用 Java 中的
Double
類檢查一個字串是否是一個數字 - 在 Java 8 中檢查一個字串是否是一個數字
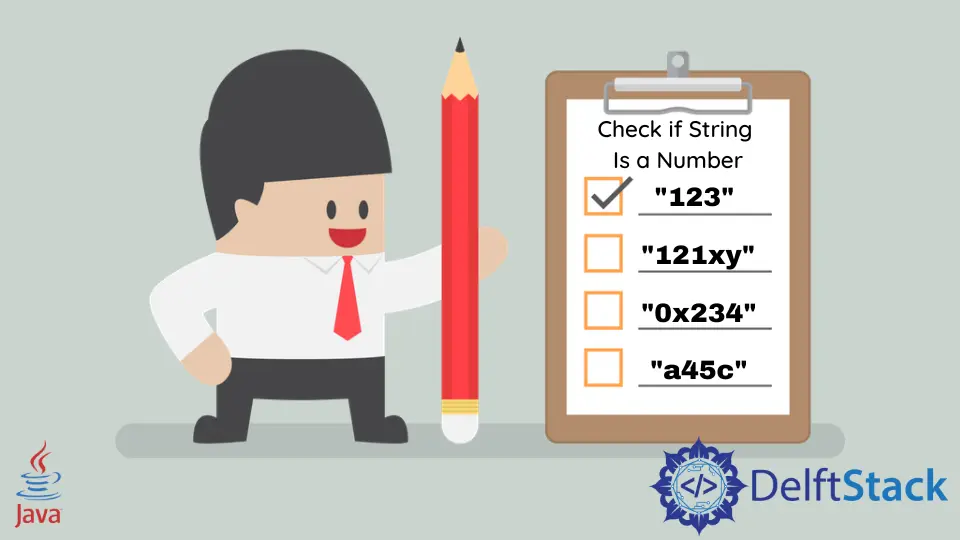
本教程將介紹在 Java 中如何檢查一個字串是否為數字,並列舉了一些示例程式碼來了解它。
檢查數字字串的方法有好幾種,如使用 regex、Double
類、Character
類或 Java 8 函式式方法等。
在 Java 中檢查一個字串是否是數字
如果且僅當字串包含數字(有效的數字位)時,它才是數字字串。例如,"123"
是一個有效的數字字串,而 "123a"
不是一個有效的數字字串,因為它包含一個字母。
為了檢查數字字串,我們可以使用 String
類的 matched()
方法,該方法以 regex
為引數,並返回一個布林值 true
或 false
。
public class SimpleTesting {
public static void main(String[] args) {
String str = "123";
boolean isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
str = "121xy";
isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
str = "0x234";
isNumeric = str.matches("[+-]?\\d*(\\.\\d+)?");
System.out.println(isNumeric);
}
}
輸出:
true
false
false
使用 Java 中的 Character
類檢查字串是否是數字
我們可以使用 Character
類的 isDigit()
方法來檢查迴圈中的每個字元。它返回 true
或 false
值。
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = true;
for (int i = 0; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
isNumeric = false;
}
}
System.out.println(isNumeric);
}
}
輸出:
true
在 Java 中使用 Apache 庫檢查一個字串是否是一個數字
如果你使用的是 Apache 庫,你可以使用 StringUtils
類的 isNumeric()
方法,如果它包含一個數字序列,則返回 true
。
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = StringUtils.isNumeric(str);
System.out.println(isNumeric);
str = "123xyz";
isNumeric = StringUtils.isNumeric(str);
System.out.println(isNumeric);
}
}
輸出:
true
false
使用 Java 中的 Double
類檢查一個字串是否是一個數字
我們可以使用 Double
類的 parseDouble()
方法,將一個字串轉換為 double
並返回一個 double
型別的值。如果它不能被解析,它會丟擲一個異常。
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
try {
Double.parseDouble(str);
System.out.println("It is a numerical string");
} catch (NumberFormatException e) {
System.out.println("It is not a numerical string");
}
}
}
輸出:
It is a numerical string
在 Java 8 中檢查一個字串是否是一個數字
如果你使用的是 Java 8 或更高版本,那麼你可以使用這個例子來檢查數字字串。這裡,Character
類的 isDigit()
方法被傳遞到 allMatch()
作為方法引用。
public class SimpleTesting {
public static void main(String[] args) {
String str = "1123";
boolean isNumeric = str.chars().allMatch(Character::isDigit);
System.out.println(isNumeric);
str = "ab234";
isNumeric = str.chars().allMatch(Character::isDigit);
System.out.println(isNumeric);
}
}
輸出:
true
false