在 Java 中獲取資源 URL 和內容
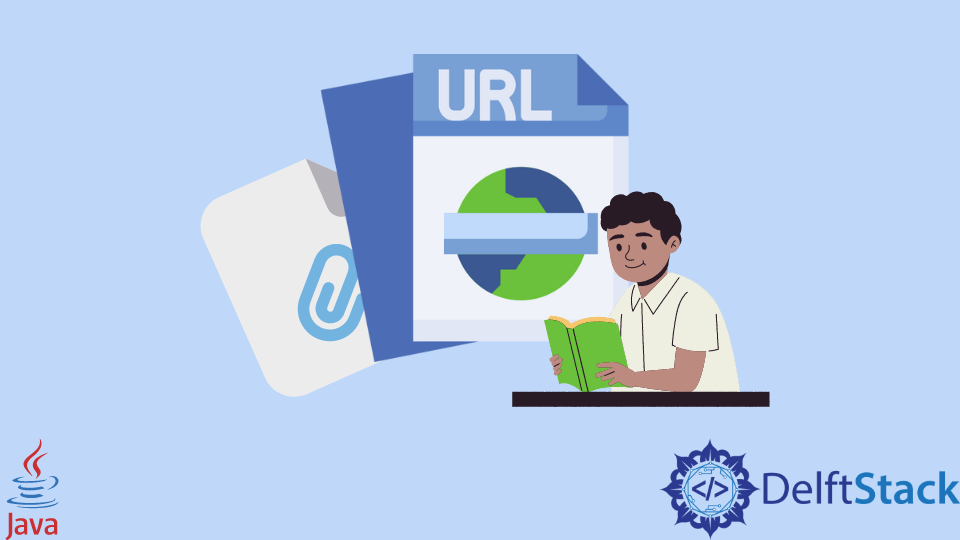
本教程將演示如何在 Java 中使用 getResource()
函式獲取資源 URL 和讀取資原始檔。
在 Java 中使用 getResource()
函式獲取資源 URL
我們將使用 Java 中的 getResource()
方法來獲取三個檔案的 URL:image.png、image1.png、resourcetext.txt。
我們將在 getResource()
函式的主體中以字串形式傳遞資源 URL。然後該函式搜尋給定的資源字串並返回一個包含 URL 的物件。
語法:
getResource(String);
public resource = yourclassname.getResource("Resource URL");
示例程式碼:
/*//you will learn how to get image URL in the following program
//import statements
*/
import java.net.URL;
import java.lang.*;
public class getImageUrl {
public static void main(String[] args) throws Exception {
getImageUrl obj = new getImageUrl();
@SuppressWarnings("rawtypes")
Class resource = obj.getClass();
URL imageurl = resource.getResource("/image.png");
System.out.println("Resource URL one is = " + imageurl);
URL imageurl2 = resource.getResource("/image2.png");
System.out.println("Resource URL two is = " + imageurl2);
URL texturl = resource.getResource("/textresource.txt");
System.out.println("Resource URL of the text file is = " + texturl);
}
}
輸出:
Resource URL one is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/image.png
Resource URL two is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/image2.png
Resource URL of the text file is = file:/C:/Users/NAME/Desktop/JAVA/get%20resource%20url%20java/bin/textresource.txt
如我們所見,我們將這三個檔案儲存在字串 URL 中。然後我們使用 obj.getClass()
方法來獲取接收影象 URL 的主類。
getResource()
函式是返回 URL 的函式。
在 Java 中使用 getResourceAsStream()
獲取資源內容
Java 保留了一個名為 getResourceAsStream()
的方法來讀取檔案。該函式返回一個 InputStream
物件,其中包含該類的指定資源。
對於下面的示例,我們將使用 getResourceAsStream()
來讀取此檔案:/get resource URL java/src/readfile/GetResourceReadFile.java
。還有字串 getresourcefromhere = "readfile/example.json";
是我們儲存 JSON 檔案的地方。
語法:
private InputStream getFileFromResourceAsStream(String getresourcefromhere) {
ClassLoader cL = getClass().getClassLoader();
InputStream inputStream = cL.getResourceAsStream(getresourcefromhere);
return inputStream;
}
如果你瞭解基本語法,請檢視以下完整程式。
該程式適用於每個平臺。你應該注意主類和檔案目錄管理。
//import necessary packages
package readfile;
import java.io.*;
import java.nio.charset.StandardCharsets;
//start function
public class GetResourceReadFile {
private static final String String = null;
public static void main(String[] args) throws IOException, Exception {
GetResourceReadFile app = new GetResourceReadFile();
//get resource file
String getresourcefromhere = "readfile/example.json";
//use inputStream to return object containing resource
InputStream getresourceandreadit = app.getFileFromResourceAsStream(getresourcefromhere);
printInputStream(getresourceandreadit);
}
private InputStream getFileFromResourceAsStream(String getresourcefromhere) {
//load class
ClassLoader cL = getClass().getClassLoader();
InputStream inputStream = cL.getResourceAsStream(getresourcefromhere);
return inputStream;
}
private static void printInputStream(InputStream r) {
try (InputStreamReader sR =
new InputStreamReader(r, StandardCharsets.UTF_8);
BufferedReader R = new BufferedReader(sR)) {
String GOT_IT = String;
//not necessary but will give you basic idea
if (GOT_IT == String) {
//you can print multiple files
while ((GOT_IT = R.readLine()) != null) {
//print file
System.out.println(GOT_IT);
}
}
}
catch (IOException e) {
e.printStackTrace();
}
}
}
輸出:
{
"File Name": "Demonstration File",
"File Type": "JSON FILE",
"File Reader": "getResource",
"File creation date:": 2/18/2022,
"Platform": {
"Langauge Type": "Programming",
"Langugae Name": "JAVA",
"Platform": "Oracle",
"Importance": "Very High"
},
"Development Environment": [
{ "JDK": "JAVA", "LATEST": "17" }
]
}
整個程式與前面帶有 URL 的示例相同。唯一的區別是 InputStream
和 ClassLoader cL = getClass().getClassLoader();
。
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn