在繼承中執行 Java 建構函式
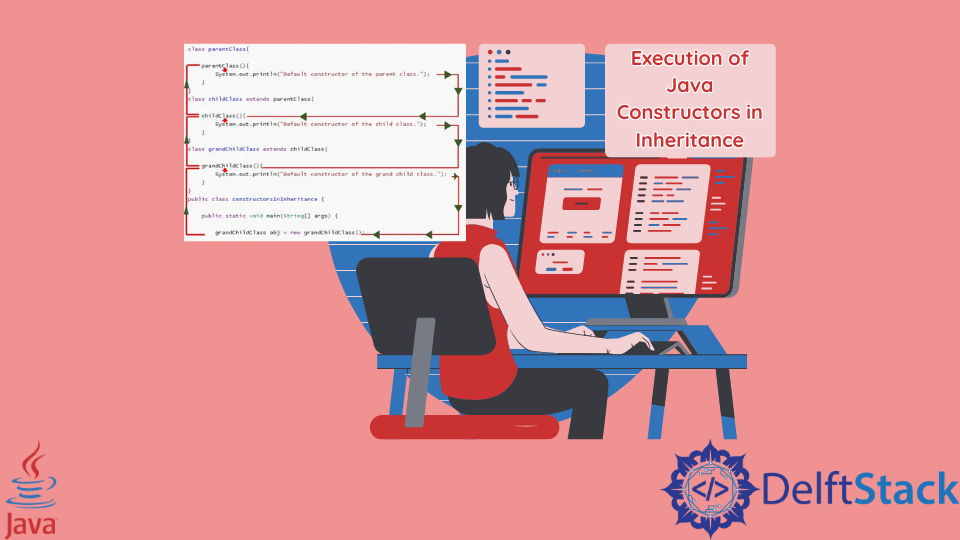
今天,我們將學習繼承中 Java 建構函式的執行。我們將在派生類(也稱為子類和子類)中看到預設建構函式和引數化建構函式的程式碼示例。
在繼承中執行 Java 建構函式
繼續這篇文章需要足夠的繼承知識。如果你正在閱讀本教程,我們假設你對 Java 繼承有深入的瞭解。
讓我們在使用 extends
關鍵字擴充套件父類(也稱為基類或超類)時瞭解 Java 建構函式的執行過程。
在繼承中執行預設 Java 建構函式
示例程式碼:
class parentClass{
parentClass(){
System.out.println("Default constructor of the parent class.");
}
}
class childClass extends parentClass{
childClass(){
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass{
grandChildClass(){
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
輸出:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
在這裡,我們有一個名為 constructorsInInheritance
的測試類,它建立 grandChildClass
的物件。
我們還有另外三個名為 parentClass
、childClass
、grandChildClass
的類,其中 grandChildClass
繼承 childClass
而 childClass
擴充套件了 parentClass
。
這裡,parentClass
預設建構函式由 childClass
建構函式自動呼叫。每當我們例項化子類時,都會自動執行父類的建構函式,然後是子類的建構函式。
觀察上面給出的輸出。如果仍然感到困惑,請參閱以下視覺說明。
如果我們在 main
方法中建立 childClass
的物件會怎樣?現在將如何執行預設建構函式?
parentClass
的建構函式將首先執行,然後 childClass
的建構函式將產生以下結果。
輸出:
Default constructor of the parent class.
Default constructor of the child class.
當父類具有預設和引數化建構函式時在繼承中執行 Java 建構函式
示例程式碼:
class parentClass{
parentClass(){
System.out.println("Default constructor of the parent class.");
}
parentClass(String name){
System.out.println("Hi " + name +
"! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass{
childClass(){
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass{
grandChildClass(){
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
輸出:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
在這裡,我們在 parentClass
中有一個引數化的建構函式。但是,仍然呼叫預設建構函式,因為我們在 main
方法中呼叫 grandChildClass()
預設建構函式,進一步呼叫父類預設建構函式。
我們還要在 childClass
和 grandChildClass
中編寫引數化建構函式。然後,在 main
函式中呼叫 grandChildClass
的引數化建構函式。
觀察呼叫了哪些建構函式,它們是預設的還是引數化的。
示例程式碼:
class parentClass{
parentClass(){
System.out.println("Default constructor of the parent class.");
}
parentClass(String name){
System.out.println("Hi " + name +
"! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass{
childClass(){
System.out.println("Default constructor of the child class.");
}
childClass(String name){
System.out.println("Hi " + name +
"! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass{
grandChildClass(){
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name){
System.out.println("Hi " + name +
"! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
輸出:
Default constructor of the parent class.
Default constructor of the child class.
Hi Mehvish! It's a parameterized constructor of the grand child class
上面的程式碼只呼叫了 grandChildClass
的引數化建構函式。我們使用 super()
呼叫 parentClass
、childClass
和 grandChildClass
的引數化建構函式。
請記住,父類建構函式呼叫必須在子類建構函式的第一行。
使用 super
呼叫父類和所有子類的引數化建構函式
示例程式碼:
class parentClass{
parentClass(){
System.out.println("Default constructor of the parent class.");
}
parentClass(String name){
System.out.println("Hi " + name +
"! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass{
childClass(){
System.out.println("Default constructor of the child class.");
}
childClass(String name){
super(name);
System.out.println("Hi " + name +
"! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass{
grandChildClass(){
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name){
super(name);
System.out.println("Hi " + name +
"! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
輸出:
Hi Mehvish! It's a parameterized constructor of the parent class
Hi Mehvish! It's a parameterized constructor of the child class
Hi Mehvish! It's a parameterized constructor of the grand child class
我們使用 super
關鍵字來呼叫引數化的父類建構函式。它指的是父類(超類或基類)。
我們用它來訪問父類的建構函式並呼叫父類的方法。
super
對於在父類和子類中具有確切名稱的方法非常有用。