在 Java 中把列舉型別轉為字元
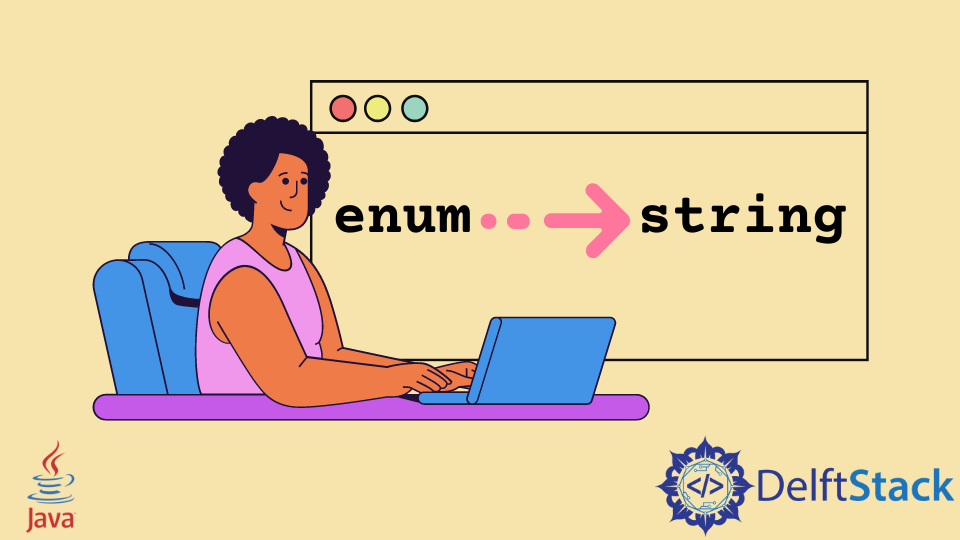
Java 中的 Enum
是一種特殊的資料型別或類,它擁有一組常量。我們也可以在列舉中新增建構函式和方法。要在 Java 中建立一個列舉,我們使用關鍵字 enum
,並像類一樣給它起一個名字。在這篇文章中,我們將介紹 Java 中將列舉轉換為字串的方法。
在 Java 中使用 name()
將列舉轉換為字串的方法
在第一個例子中,我們將使用 Enum
類的 name()
以字串形式返回列舉常量的確切名稱。下面,我們在類內部建立了一個列舉,但我們可以在類外或類內建立一個列舉。我們將這個列舉命名為 Directions
,它包含了作為列舉常量的方向名稱。
我們可以使用 name()
方法獲取任何常量。Directions.WEST.name()
將以字串形式返回 WEST
,儲存在字串變數 getWestInString
中,然後列印在輸出中。
public class EnumToString {
enum Directions {
NORTH,
SOUTH,
EAST,
WEST
}
public static void main(String[] args) {
String getWestInString = Directions.WEST.name();
System.out.println(getWestInString);
}
}
輸出:
WEST
在 Java 中使用 toString()
將列舉轉換為字串
就像 name()
一樣,我們有 toString()
方法,但是當涉及到使用一個列舉常量做任何重要的用途時,name()
是首選,因為它返回的是同一個常量,而 toString()
可以在列舉中被覆蓋。這意味著我們可以使用 toString()
修改作為字串返回的內容,我們將在下一個例子中看到。
在這個例子中,我們在需要轉換為字串的常量上使用 toString()
方法。
public class EnumToString {
enum Currencies {
USD,
YEN,
EUR,
INR
}
public static void main(String[] args) {
String getCurrency = Currencies.USD.toString();
System.out.println(getCurrency);
}
}
輸出:
USD
我們在上面已經討論過,我們可以重寫 toString()
方法來修改我們想用列舉常量返回的字串。在下面的例子中,我們有四種貨幣作為常量呼叫列舉建構函式,並傳遞一個字串作為引數。
每當常量看到 toString()
方法時,就會把字串名傳給 getCurrencyName
這個字串變數。現在我們要在列舉裡面覆蓋 toString()
方法,用字串返回 getCurrencyName
。
在 main()
方法中,我們使用 toString()
方法將 INR
常量作為字串獲取。我們可以在輸出中看到,修改後的字串被列印出來。我們也可以使用 Enum.values()
來列印一個列舉的所有值,它返回一個列舉常量陣列,然後在每個常量中迴圈,把它們列印成字串。
public class EnumToString {
enum Currencies {
USD("USD"),
YEN("YEN"),
EUR("EUR"),
INR("INR");
private final String getCurrencyName;
Currencies(String currencyName) {
getCurrencyName = currencyName;
}
@Override
public String toString() {
return "Currency: " + getCurrencyName;
}
}
public static void main(String[] args) {
String getCurrency = Currencies.INR.toString();
System.out.println("Your " + getCurrency);
Currencies[] allCurrencies = Currencies.values();
for (Currencies currencies : allCurrencies) {
System.out.println("All " + currencies);
}
}
}
輸出:
Your Currency: INR
All Currency: USD
All Currency: YEN
All Currency: EUR
All Currency: INR
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn相關文章 - Java String
- 如何在 Java 中以十六進位制字串轉換位元組陣列
- 如何在 Java 中執行字串到字串陣列的轉換
- 如何將 Java 字串轉換為位元組
- 如何從 Java 中的字串中刪除子字串
- 用 Java 生成隨機字串
- Java 中的交換方法