在 Java 中建立目錄
Mohd Ebad Naqvi
2023年1月30日
2021年6月29日
-
在 Java 中使用
mkdir()
函式建立目錄 -
在 Java 中使用
mkdirs()
函式建立目錄 -
在 Java 中使用
createDirectory()
函式建立目錄 -
在 Java 中使用
createDirectories()
函式建立目錄 -
在 Java 中使用
forceMkdir()
函式建立目錄
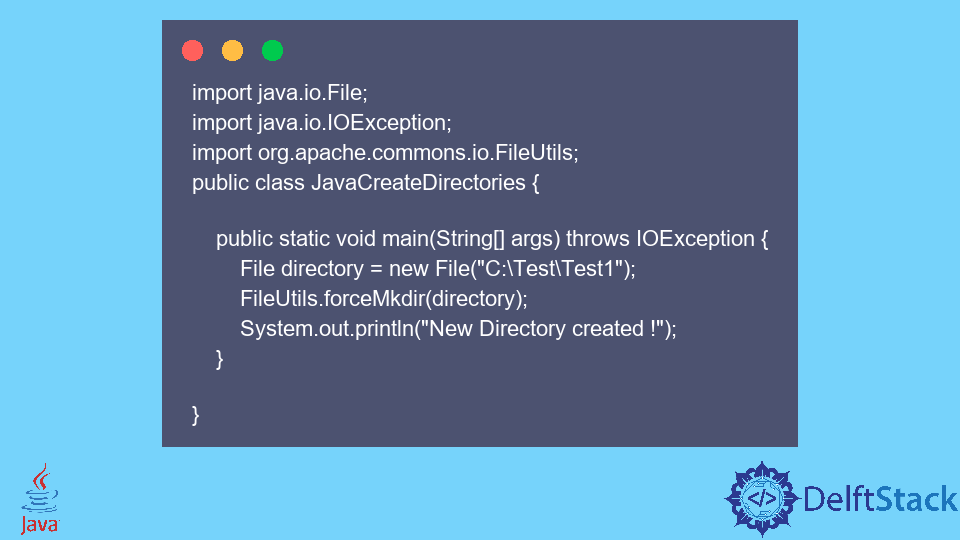
目錄是用於在計算機上儲存檔案的位置或檔案系統編目結構。我們在 Java 中處理不同的檔案處理操作。檔案物件用於建立新資料夾或目錄。
在本教程中,我們將在 Java 中建立一個目錄。
在 Java 中使用 mkdir()
函式建立目錄
首先,建立檔案類的例項。然後引數傳遞給這個例項,就是我們要製作的目錄路徑。最後,使用檔案物件呼叫 mkdir()
方法,建立所需的目錄。
例如,
import java.io.File;
import java.util.Scanner;
public class CreateDirectory {
public static void main(String args[]) {
System.out.println("Path of Directory? ");
Scanner obj = new Scanner(System.in);
String path = obj.next();
System.out.println("Directory Name? ");
path = path+obj.next();
File D = new File(path);
boolean D1 = D.mkdir();
if(D1){
System.out.println("Directory is created successfully");
}else{
System.out.println("Error !");
}
}
}
輸出:
Path of Directory?
C:/
Directory Name?
TestDirectory
Directory is created successfully
在 Java 中使用 mkdirs()
函式建立目錄
mkdir()
方法的缺點是 Java 一次只能使用它來建立一個目錄。這個缺點可以通過使用 mkdirs()
方法解決,因為我們可以使用它來建立目錄層次結構。即,父目錄中的子目錄等等。
例如,
import java.io.File;
import java.util.Scanner;
public class ABC {
public static void main(String args[]) {
System.out.println("Path? ");
Scanner obj = new Scanner(System.in);
String path = obj.next();
System.out.println("Name of Directory? ");
path = path+obj.next();
File D = new File(path);
boolean D1 = D.mkdirs();
if(D1){
System.out.println("Folder is created successfully");
}else{
System.out.println("Error Found!");
}
}
輸出:
Path?
C:/
Name of Directory?
TestDirectory/TestDirectory1
Folder is created successfully
使用 mkdirs()
方法在其父目錄 TestDirectory 中建立 TestDirectory1。這不能使用 mkdir()
方法完成。
在 Java 中使用 createDirectory()
函式建立目錄
Files.createDirectory()
也可以在 Java 中建立一個新目錄。如果已存在同名檔案,則丟擲 FileAlreadyExistsException
。
例如,
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class JavaCreateDirectory {
public static void main(String[] args) throws IOException {
String fileName = "C:\EbadTest\NewTest";
Path path = Paths.get(fileName);
if (!Files.exists(path)) {
Files.createDirectory(path);
System.out.println("New Directory created ! "+filename);
} else {
System.out.println("Directory already exists");
}
}
}
輸出:
New Directory created ! C:\EbadTest\NewTest
如果目錄已經存在,則程式碼將給出以下輸出。
Directory already exists
如果層次結構不存在,上面的程式碼將丟擲 NoSuchFileException
。
在 Java 中使用 createDirectories()
函式建立目錄
如果父目錄不存在,Files.createDirectories()
可以建立一個新目錄。它還可以建立目錄層次結構。如果目錄已經存在,createDirectories()
方法不會丟擲異常。
例如,
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class JavaCreateDirectories {
public static void main(String[] args) throws IOException {
String fileName = "C:\NonExisting1\NonExisting2";
Path path = Paths.get(fileName);
Files.createDirectories(path);
System.out.println("New Directory created ! "+fileName);
}
}
輸出:
New Directory created ! C:\NonExisting1\NonExisting2
在 Java 中使用 forceMkdir()
函式建立目錄
org.apache.commons.io.FileUtils
包具有 forceMkdir()
函式,可根據需要建立目錄和父目錄。如果與目錄同名的檔案或目錄已存在,則丟擲異常。
例如,
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
public class JavaCreateDirectories {
public static void main(String[] args) throws IOException {
File directory = new File("C:\Test\Test1");
FileUtils.forceMkdir(directory);
System.out.println("New Directory created !");
}
}
輸出:
New Directory created !