用 Java 製作倒數計時器
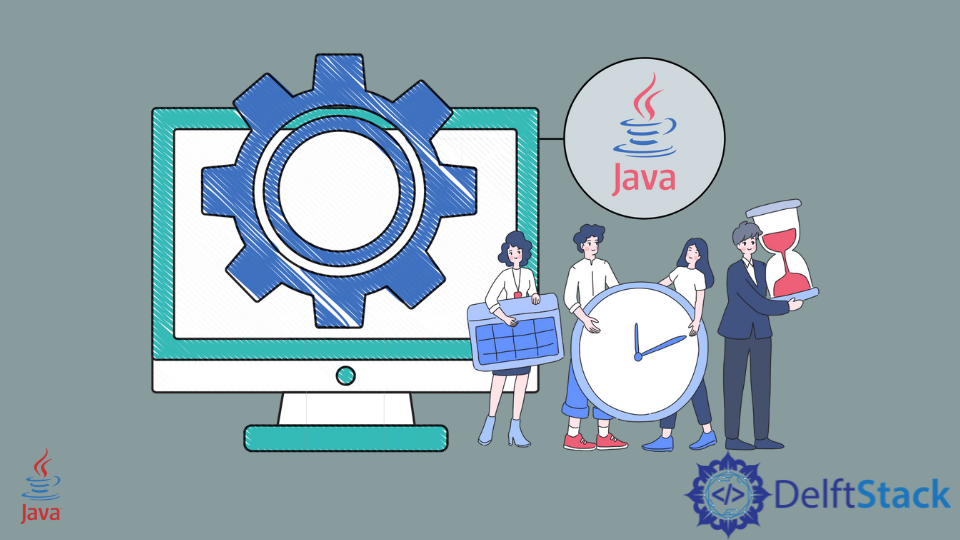
今天,我們將演示如何在不依賴任何第三方庫的情況下使用兩種方法在 Java 中製作倒數計時器。我們可以在 GUI 視窗或控制檯中使用計時器來測試它。看看我們下面的程式碼!
Java 中使用 ScheduledExecutorService
的倒數計時器
在第一個方法中,我們使用 ExecutorService
介面呼叫其方法 newScheduledThreadPool()
,該方法建立一個使用相同固定數量分配執行緒的執行緒池。在 newScheduledThreadPool()
方法中,我們傳遞了我們想要在池中的線程數。在這種情況下,我們只需要一個執行緒來執行計時器。
Executors.newScheduledThreadPool()
函式返回一個 ScheduledExecutorService
物件,我們將其命名為 scheduler
。接下來,我們從 Runnable
介面覆蓋 run()
方法。Runnable
例項用於執行執行緒。在 Runnable
中,我們建立了一個變數 countdownStarter
並使用我們想要啟動倒數計時器的秒數對其進行初始化。
現在在 run()
方法中,我們列印 countdownStarter
,並將其值減一。為了在計時器達到零時停止計時器,我們建立了一個條件來檢查 countdownStarter
值。如果它的值小於零,它會列印一條訊息並關閉 scheduler
,它是使用 scheduler.shutdown()
命令的 Executor Service。
最後,我們使用 scheduler
物件呼叫 scheduleAtFixedRate()
方法,該方法定期執行給定的操作並接受四個引數。第一個引數是 runnable
例項;第二個是第一次執行的時間延遲;三是動作之間的延遲。在這裡,正如第四個引數所暗示的那樣,我們將延遲設為應等於一秒。
import java.util.concurrent.*;
import static java.util.concurrent.TimeUnit.SECONDS;
public class Countdown {
public static void main(String[] args) {
final ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
final Runnable runnable = new Runnable() {
int countdownStarter = 20;
public void run() {
System.out.println(countdownStarter);
countdownStarter--;
if (countdownStarter < 0) {
System.out.println("Timer Over!");
scheduler.shutdown();
}
}
};
scheduler.scheduleAtFixedRate(runnable, 0, 1, SECONDS);
}
}
輸出:
20
19
18
17
16
15
14
13
12
11
10
9
8
7
6
5
4
3
2
1
0
Timer Over!
Java 中使用 Timer
和 TimerTask
的倒數計時器
在這個例子中,我們使用了 java.util
包附帶的兩個類,Timer
和 TimerTask
。這兩個類都用於排程在後臺執行緒中執行的任務。在這個例子中,我們使用 jFrame
物件在 GUI 視窗中顯示計時器。首先,我們建立一個 jFrame
物件,然後是一個顯示計時器文字的 jLabel
。我們將框架的佈局設定為 FlowLayout()
,並設定視窗的位置和大小。
接下來,我們將 jLabel
新增到框架中。為了建立倒數計時器,我們建立了一個 Timer
物件並呼叫它的方法 scheduleAtFixedRate()
,顧名思義,它以固定的速率安排和重複指定的任務。
現在,由於 scheduleAtFixedRate()
mate 將 TimerTask
物件作為它的第一個引數,我們使用 new TimerTask()
建立一個物件。對於第二個和第三個引數,它們安排要執行的任務以及每次執行之間的時間間隔(以毫秒為單位)。
下一步,我們需要建立一個變數並用我們想要開始倒計時的數字初始化它。然後,我們呼叫 run()
方法並將 jLabel
元件的文字設定為 i
。要停止計時器,我們呼叫 timer.cancel()
。
import javax.swing.*;
import java.awt.*;
import java.util.Timer;
import java.util.TimerTask;
public class Countdown {
public static void main(String[] args) {
JFrame jframe = new JFrame();
JLabel jLabel = new JLabel();
jframe.setLayout(new FlowLayout());
jframe.setBounds(500, 300, 400, 100);
jframe.add(jLabel);
jframe.setVisible(true);
Timer timer = new Timer();
timer.scheduleAtFixedRate(new TimerTask() {
int i = 20;
public void run() {
jLabel.setText("Time left: " + i);
i--;
if (i < 0) {
timer.cancel();
jLabel.setText("Time Over");
}
}
}, 0, 1000);
}
}
輸出:
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn