獲取 Java 中檔案的行數
Farkhanda Athar
2023年1月30日
2021年11月8日
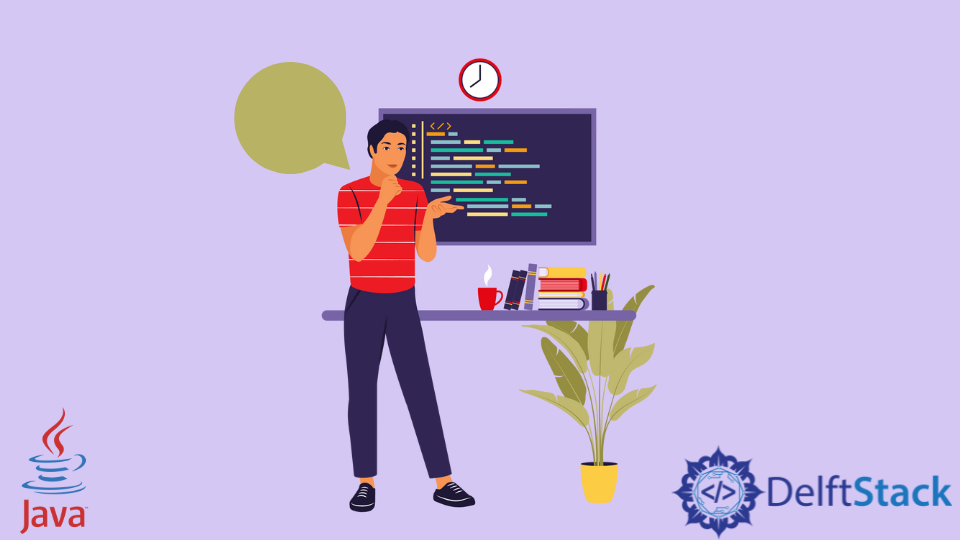
本文將解釋計算檔案中總行數的各種方法。
計算某檔案中的行數的過程包括四個步驟:
- 開啟檔案。
- 逐行讀取並在每行後將計數加一。
- 關閉檔案。
- 讀取計數。
這裡我們使用了兩種方法來計算檔案中的行數。這些方法是 Java File
和 Scanner
類。
使用 Java 中的 Scanner
類計算檔案中的行數
在這種方法中,使用了 Scanner
類的 nextLine()
方法,該方法訪問檔案的每一行。行數取決於 input.txt
檔案中的行數。該程式還列印檔案內容。
示例程式碼:
import java.io.File;
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int count = 0;
try {
File file = new File("input.txt");
Scanner sc = new Scanner(file);
while(sc.hasNextLine()) {
sc.nextLine();
count++;
}
System.out.println("Total Number of Lines: " + count);
sc.close();
} catch (Exception e) {
e.getStackTrace();
}
}
}
如果檔案由三行組成,如下所示。
This is the first line.
This is the second line.
This is the third line.
然後輸出將是,
輸出:
Total Number of Lines: 3
使用 java.nio.file
包計算檔案中的行數
為此,lines()
方法會將檔案的所有行作為流讀取,而 count()
方法將返回流中的元素數。
示例程式碼:
import java.nio.file.*;
class Main {
public static void main(String[] args) {
try {
Path file = Paths.get("input.txt");
long count = Files.lines(file).count();
System.out.println("Total Lines: " + count);
} catch (Exception e) {
e.getStackTrace();
}
}
}
輸出:
Total Lines: 3