GO 中如何在逐行高效地逐行讀取檔案
Wechuli Paul
2023年12月11日
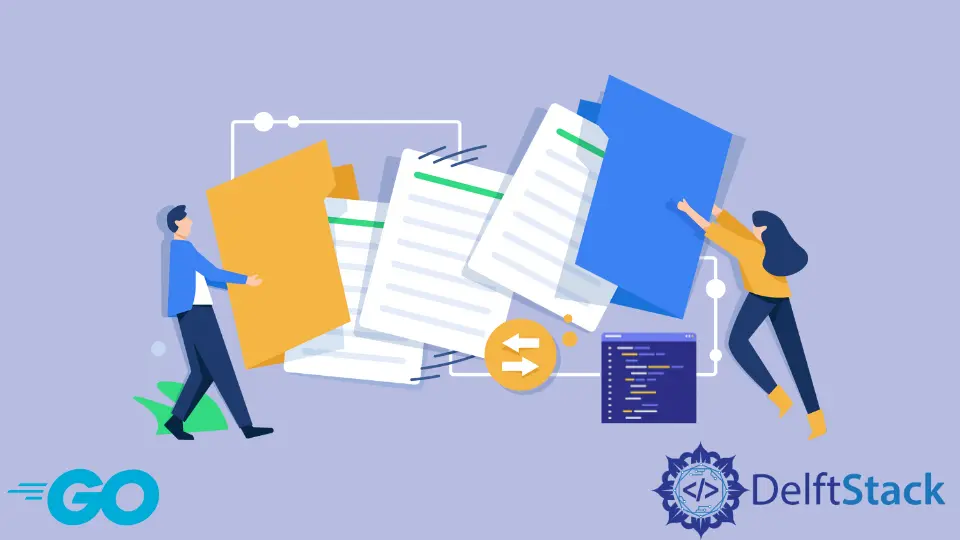
檔案 I/O,特別是對檔案的讀寫是程式語言中重要的功能。通常,你需要逐行讀取檔案。GO 提供了 bufio
軟體包,該軟體包基本上實現了緩衝的 I/O。bufio
提供有用的型別和函式,特別是用於讀取和寫入文字檔案。
包和必要的 Import
package main
import(
"fmt"
"bufio"
"log"
"os"
)
我們將需要從 GO 標準庫中匯入多個檔案:-
- OS - 用於獨立於平臺的 OS 函式介面
- FMT - 實現格式化的 I/O 函式
- log - 標準日誌記錄包
- bufio - 支援緩衝的 I/O
Go 中對檔案逐行讀取
在讀取檔案之前,首先需要使用 os.Open()
函式將其開啟,該函式返回指向檔案的指標型別。程式碼段中顯示的 test.txt
檔案需要已經存在在系統中(將路徑放置到檔案所在的位置)。
bufio.NewScanner(file)
函式返回 scanner
型別,該型別中的函式支援讀取檔案。
要逐行讀取檔案,我們需要使用兩種在新的 Scanner
的方法-Scan
,它會將 Scanner
前進到新的符記(在本例中為新行),和 Text
(或 Byte
)讀取呼叫 Scan
時生成的最新符記。
如果在讀取檔案時遇到任何錯誤,可以通過在新的 Scanner
上呼叫 Err()
方法來處理這些錯誤,該方法將返回 Scanner
遇到的第一個非檔案結尾錯誤。
func main(){
// open the file
file, err := os.Open("test.txt")
//handle errors while opening
if err != nil {
log.Fatalf("Error when opening file: %s", err)
}
fileScanner := bufio.NewScanner(file)
// read line by line
for fileScanner.Scan() {
fmt.Println(fileScanner.Text())
}
// handle first encountered error while reading
if err := fileScanner.Err(); err != nil {
log.Fatalf("Error while reading file: %s", err)
}
file.Close()
}
Go 中逐行讀取檔案的完整程式碼
下面提供了 main.go
中用於逐行讀取檔案的完整程式碼。
你需要輸入:
$ go run main.go
在終端中執行程式。
package main
import (
"bufio"
"fmt"
"log"
"os"
)
func main() {
// open the file
file, err := os.Open("test.txt")
//handle errors while opening
if err != nil {
log.Fatalf("Error when opening file: %s", err)
}
fileScanner := bufio.NewScanner(file)
// read line by line
for fileScanner.Scan() {
fmt.Println(fileScanner.Text())
}
// handle first encountered error while reading
if err := fileScanner.Err(); err != nil {
log.Fatalf("Error while reading file: %s", err)
}
file.Close()
}
在 Go 中配置 Scanner
行為
Scanner
型別具有 Split
函式,該函式接受 SplitFunc
函式來確定 Scanner
如何拆分給定的位元組片。預設的 SplitFunc
是 ScanLines
,它將返回文字的每一行,並刪除行尾標記。
例如,我們可以使用單詞進行拆分,如下面的程式碼片段所示:-
scanner.Split(bufio.ScanWords) //configure how the scanner behaves