Go 中的列舉器
Jay Singh
2023年1月30日
2022年4月22日
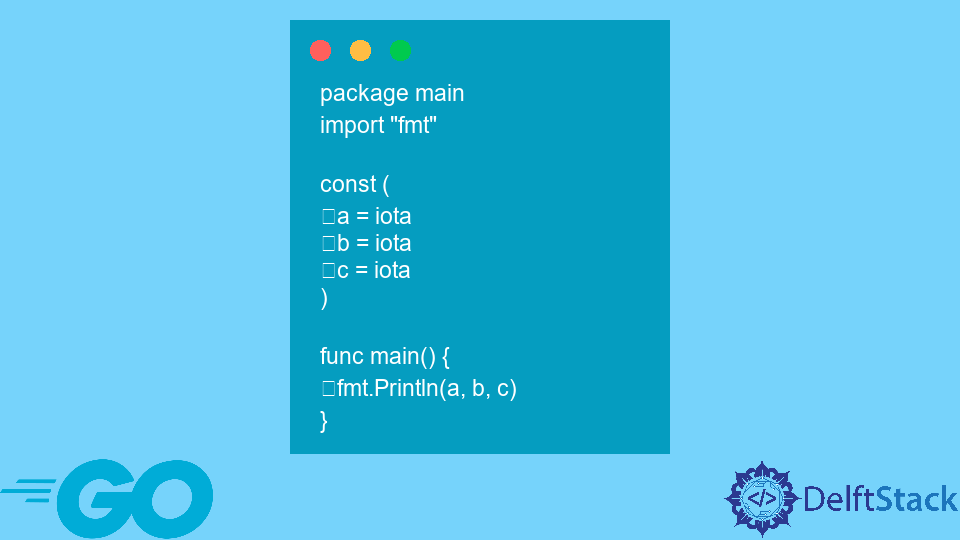
enum
(enumerator 的縮寫)用於設計複雜的常量分組,這些常量具有有意義的名稱,但值簡單且不同。
在 Go 中,沒有列舉
資料型別。我們使用預定義的識別符號 iota
,並且 enums
不是嚴格型別的。
在 Go 中使用 iota
表示 enums
iota
是一個常量識別符號,可以簡化自動遞增數字的宣告。它表示一個從零開始的整數常量。
iota
關鍵字表示數字常量 0, 1, 2,...
。原始碼中出現的術語 const
重置為 0
並隨著每個 const
規範而增加。
package main
import "fmt"
const (
a = iota
b = iota
c = iota
)
func main() {
fmt.Println(a, b, c)
}
輸出:
0 1 2
在 Go 中使用 iota
自動遞增數字宣告
使用這種方法,你可以避免在每個常量前面放置連續的 iota
。
package main
import "fmt"
const (
a = iota
b
c
)
func main() {
fmt.Println(a, b, c)
}
輸出:
0 1 2
在 Go 中使用 iota
建立常見行為
我們定義了一個名為 Direction
的簡單列舉
,它有四個潛在值:"east"
、"west"
、"north"
和"south"
。
package main
import "fmt"
type Direction int
const (
East = iota + 1
West
North
South
)
// Giving the type a String method to create common behavior
func (d Direction) String() string {
return [...]string{"East", "West", "North", "South"}[d-1]
}
// Giving the type an EnumIndex function allows you to provide common behavior.
func (d Direction) EnumIndex() int {
return int(d)}
func main() {
var d Direction = West
fmt.Println(d)
fmt.Println(d.String())
fmt.Println(d.EnumIndex())
}
輸出:
West
West
2