C# 中具有多個條件的 if 語句
Abdullahi Salawudeen
2023年1月30日
2022年4月20日
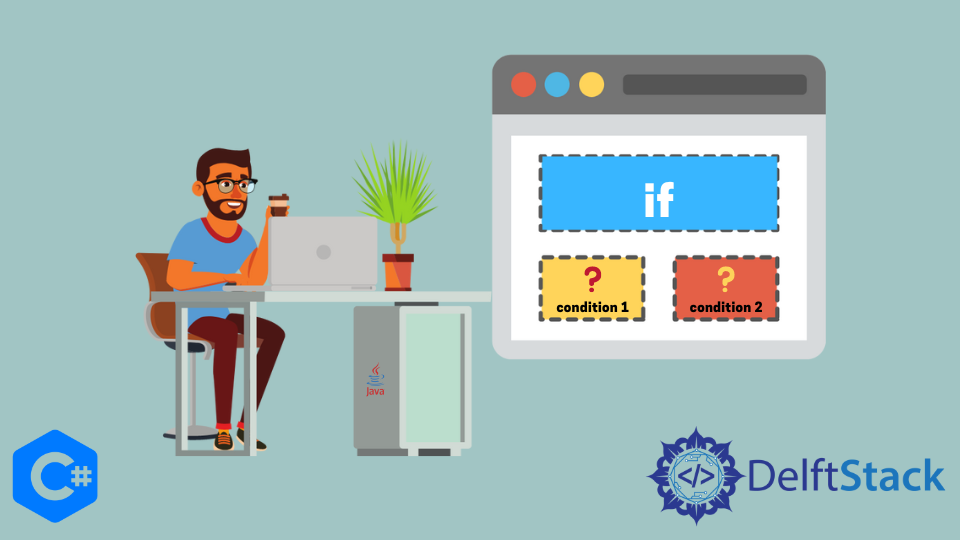
條件語句用於控制程式執行的流程,並根據條件是否為真來執行。C# 中有兩個條件分支語句:if
和 switch
語句。
本文將介紹在 C# 中使用帶有多個條件的 if
語句來返回語句。進一步的討論可通過 this reference 獲得。
在 C#
中使用運算子
運算子用於對 C# 中的變數和值執行不同的操作。運算子可分為四類:算術、賦值、比較和邏輯運算子。
比較運算子允許在 C# 中比較兩個值。C# 中有六個比較運算子。
< |
少於 | a < b |
> |
大於 | a > b |
== |
等於 | a == b |
<= |
小於或等於 | a <= b |
>= |
大於或等於 | a >= b |
!= |
不等於 | a != b |
邏輯運算子具有三個比較。
- 邏輯與 (
&&
) - 如果兩個比較語句都為真,則返回真。否則,它返回 false。 - 邏輯或 (
||
) - 如果一個或兩個比較語句為真,則返回真。只有當兩個比較語句都為假時,它才返回假。 - 邏輯非 (
!
) - 否定任何比較語句或引數。如果結果為假,則返回真,反之亦然。
我們可以單獨或組合使用邏輯運算子。
在 C#
中使用具有多個邏輯條件的 if
語句
程式碼片段:
using System;
class demo
{
public static void Main()
{
string a = "Abdul", b = "Salawu", c = "Stranger", A2 = "Age";
bool checkbox = true;
string columnname = "Abdullahi Salawudeen";
if (columnname != a && columnname != b && columnname != c
&& (checkbox || columnname != A2))
{
Console.WriteLine("Columnname is neither equal to a nor b nor c nor A2, but the check box is checked");
}
//the else statement is necessary to stop the program from executing infinitely
else{
Console.WriteLine("columnname is unknown and checkbox is false");
}
}
}
輸出:
Columnname is neither equal to a nor b nor c nor A2, but the check box is checked
C#
中的三元條件運算子
條件運算子 ?:
也稱為三元條件運算子,其工作方式類似於 if 語句。它計算一個布林表示式並返回兩個表示式之一的結果。
如果布林表示式為真,則返回第一個語句(即 ?
之後的語句),否則返回第二個語句(即 :
後的語句)。進一步的討論可通過此參考獲得。
語法:
condition ? consequent : alternative;
下面是使用具有多個邏輯條件的三元運算子的程式碼示例。
using System;
class demo
{
public static void Main()
{
string a = "Abdul", b = "Salawu", c = "Stranger", A2 = "Age";
bool checkbox = false;
string columnname = A2;
string x =(columnname != a && columnname != b && columnname != c
&& (checkbox || columnname != A2)) ? "Columnname is neither equal to a nor b bor c nor A2 nor is the check box true" : "columnname is unknown and checkbox is false";
Console.WriteLine(x);
}
}
輸出:
columnname is unknown and checkbox is false
Author: Abdullahi Salawudeen