如何在 C# 中重新命名檔案
Minahil Noor
2024年2月16日
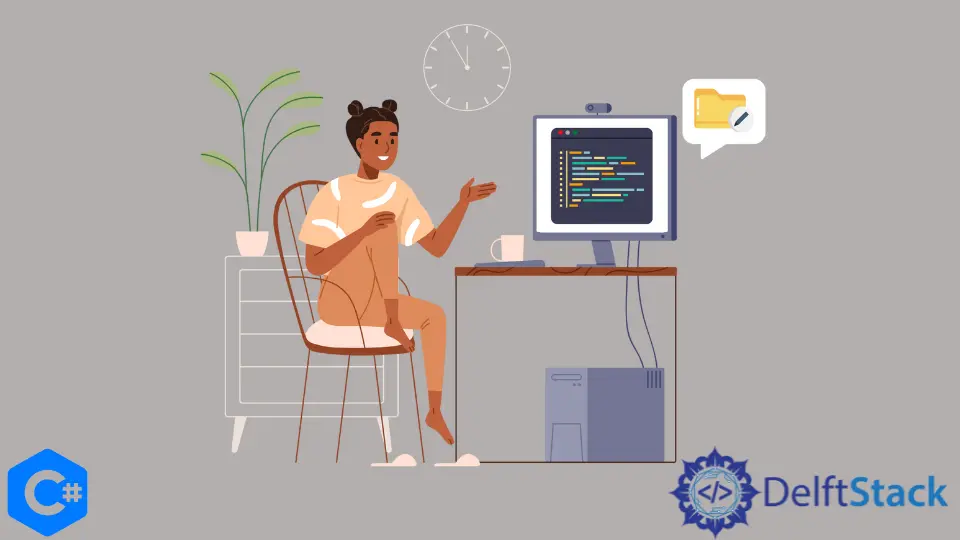
在本文中,我們將介紹使用 C# 程式碼重新命名檔案的不同方法。
- 使用
Move()
方法 - 使用
Copy()
方法
在 C# 中使用 Move()
方法重新命名檔案
我們將使用系統定義的方法 Move()
來重新命名一個檔案。我們將把舊檔案移動到一個新的檔案中,並使用新的名字。使用該方法的正確語法如下。
File.Move(string oldFile, string newFile);
內建方法 Move()
有兩個引數。它的詳細引數如下
引數 | 說明 | |
---|---|---|
oldFile |
強制 | 它是我們要重新命名的檔案。它是一個字串變數。 |
newFile |
強制 | 它是新檔案的新名稱和路徑 |
下面的程式顯示了我們如何使用 Move()
方法來重新命名一個檔案。
using System;
using System.IO;
class RenameFile {
static void Main() {
string oldName = "D:\myfolder\myfile.txt";
string newName = "D:\myfolder\mynewfile.txt";
System.IO.File.Move(oldName, newName);
}
}
該檔案必須存在於指定的目錄中,如果它不存在,那麼函式將丟擲 FileNotFoundException
錯誤。
在 C# 中使用 Copy()
方法重新命名檔案
我們將使用 Copy()
方法來重新命名一個檔案。該方法將檔案複製到一個新的檔案中,並將其目錄改為指定的目錄。使用該方法的正確語法如下。
File.Copy(string oldFile, string newFile);
內建方法 Copy()
有兩個引數。它的詳細引數如下。
引數 | 說明 | |
---|---|---|
oldFile |
強制 | 它是我們要複製到新檔案中的檔案。它是一個字串變數。 |
newFile |
強制 | 它是新檔案。它是一個字串變數。 |
下面的程式顯示了我們如何使用 Copy()
方法來重新命名一個檔案。
using System;
using System.IO;
public class RenameFile {
public static void Main() {
string oldFile = @"D:\oldfile.txt";
string newFile = @"D:\newfile.txt";
File.Copy(oldFile, newFile);
}
}