C# 隨機布林值
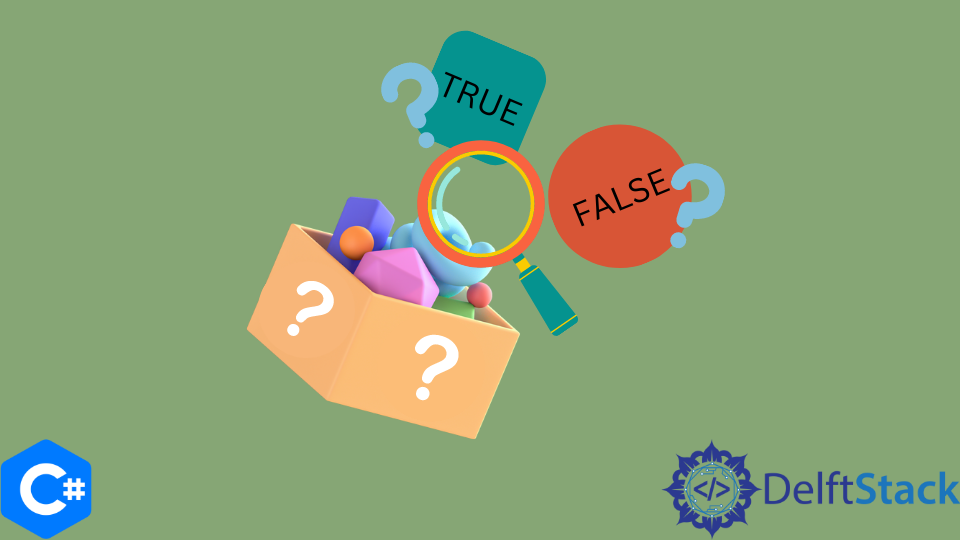
你是否遇到過必須以程式設計方式擲硬幣的情況?你有沒有想過在兩個資料庫之間隨機分配東西?
你試圖實現的目標是我們所說的隨機布林值
。boolean
變數只有兩個可能的值,true
或 false
。
當我們隨機生成這個布林值時,我們得到一個隨機布林值。本教程將展示如何使用 C# 建立一個 random boolean
。
我們還將看到其生成的最快和最理想的方式。
使用 C# 類 Random
中的 Next()
方法
C# 中的 Random
類為我們提供了一個隨機庫。它提供了一個偽隨機生成器,可以根據統計要求生成隨機序列。
儘管該類沒有像為 bytes
、integers
和 doubles
那樣明確地提供 boolean 方法。但它可以有效地使用它來生成它們,因為從程式設計師的角度來看,false
和 true
只不過是 0
和 1
。
第一種方法是使用 Next()
方法通過將 n
分配為 2
並減少僅構成 0
和 1
的容量來生成 [0,n)
範圍內的隨機整數.
// Generate n random booleans using C# class Random
using System;
public class RandomBoolean
{
public static void Main()
{
int n = 5;
var random = new Random();
for (int i = 0; i < n; i++)
{
Console.WriteLine(random.Next(2) == 1);
}
}
}
輸出:
False
True
True
False
False
// Note this is random and may not match with your actual output
使用 C# 類 Random
中的 NextDouble()
方法
在前面的示例中,我們看到了如何使用 Next()
生成隨機布林值。在這種方法中,我們將看到如何使用同一類 Random
中的另一種方法 NextDouble()
。
NextDouble()
返回一個介於 0.0
和 1.0
之間的隨機雙精度值。因此,我們可以在這兩個數字之間的任意位置新增分隔符,並根據分隔條件將生成的數字分為真
或假
。
例如,讓我們選擇雙值 0.3
作為分隔符,分隔條件為生成數> = 0.3
。因此,如果數字滿足條件,我們得到 true
,否則為 false
。
為了實現足夠的隨機分佈,分隔符的首選值為 0.5
。
// Generate n random booleans using C# class Random
using System;
public class RandomBoolean
{
public static void Main()
{
int n = 5;
var random = new Random();
for (int i = 0; i < n; i++)
{
Console.WriteLine(random.NextDouble()>=0.5);
}
}
}
輸出:
True
False
True
True
False
// Note this is random and may not match with your actual output
帖子中描述的兩種方法都相當快。
但是假設我們必須選擇最快的。在這種情況下,第二種方法似乎更短。
Next()
方法在內部返回 (int)(this.Sample()*maxValue)
,而 NextDouble()
僅返回 this.Sample()
,導致乘法和強制轉換的額外開銷。
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn