C# 中的優先佇列
Minahil Noor
2021年3月21日
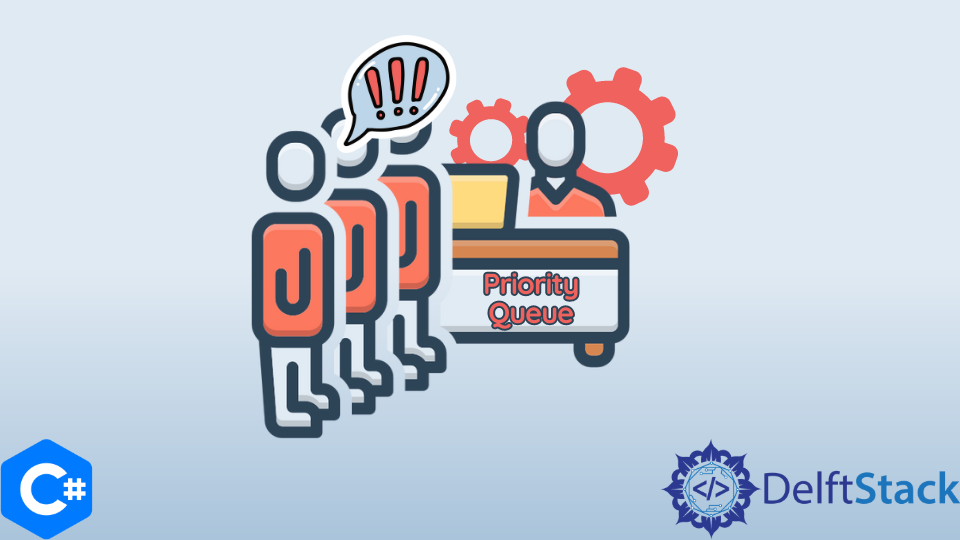
本文將介紹 C# 的優先佇列等效。
在 C# 中使用列表作為優先佇列
在 C# 中,優先佇列沒有特殊的類。但是我們可以使用陣列和列表來實現它。我們將使用列表資料結構實現優先佇列。優先佇列是一種抽象資料型別,具有與其元素相關聯的優先順序。將以始終將最高優先順序放在首位的方式建立列表。使用該列表實現優先佇列的演算法如下。
PUSH(HEAD, VALUE, PRIORITY)
Step 1: Create a new node with VALUE and PRIORITY
Step 2: Check if HEAD has lower priority. If true follow Steps 3-4 and end. Else goto Step 5.
Step 3: NEW -> NEXT = HEAD
Step 4: HEAD = NEW
Step 5: Set TEMP to head of the list
Step 6: While TEMP -> NEXT != NULL and TEMP -> NEXT -> PRIORITY > PRIORITY
Step 7: TEMP = TEMP -> NEXT
[END OF LOOP]
Step 8: NEW -> NEXT = TEMP -> NEXT
Step 9: TEMP -> NEXT = NEW
Step 10: End
POP(HEAD)
Step 2: Set the head of the list to the next node in the list. HEAD = HEAD -> NEXT.
Step 3: Free the node at the head of the list
Step 4: End
TOP(HEAD):
Step 1: Return HEAD -> VALUE
Step 2: End
push
操作會將元素新增到優先佇列,以使優先順序最高的元素始終位於最前面。彈出操作將從佇列中刪除具有最高優先順序的元素。最上面的操作將檢索最高優先順序的元素,而不會將其刪除。下面的程式顯示瞭如何從優先佇列中推送,彈出和放置頂部元素。
using System;
class PriorityQueue
{
public class Node
{
public int data;
public int priority;
public Node next;
}
public static Node node = new Node();
public static Node newNode(int d, int p)
{
Node temp = new Node();
temp.data = d;
temp.priority = p;
temp.next = null;
return temp;
}
public static int top(Node head)
{
return (head).data;
}
public static Node pop(Node head)
{
Node temp = head;
(head) = (head).next;
return head;
}
public static Node push(Node head,
int d, int p)
{
Node start = (head);
Node temp = newNode(d, p);
if ((head).priority > p)
{
temp.next = head;
(head) = temp;
}
else
{
while (start.next != null &&
start.next.priority < p)
{
start = start.next;
}
temp.next = start.next;
start.next = temp;
}
return head;
}
public static int isEmpty(Node head)
{
return ((head) == null) ? 1 : 0;
}
public static void Main(string[] args)
{
Node queue = newNode(1, 1);
queue = push(queue, 9, 2);
queue = push(queue, 7, 3);
queue = push(queue, 3, 0);
while (isEmpty(queue) == 0)
{
Console.Write("{0:D} ", top(queue));
queue = pop(queue);
}
}
}
輸出:
3 1 9 7
priority
值較小的元素具有較高優先順序。