在 C# 中的 Windows 窗體應用程式中播放 Mp3 檔案
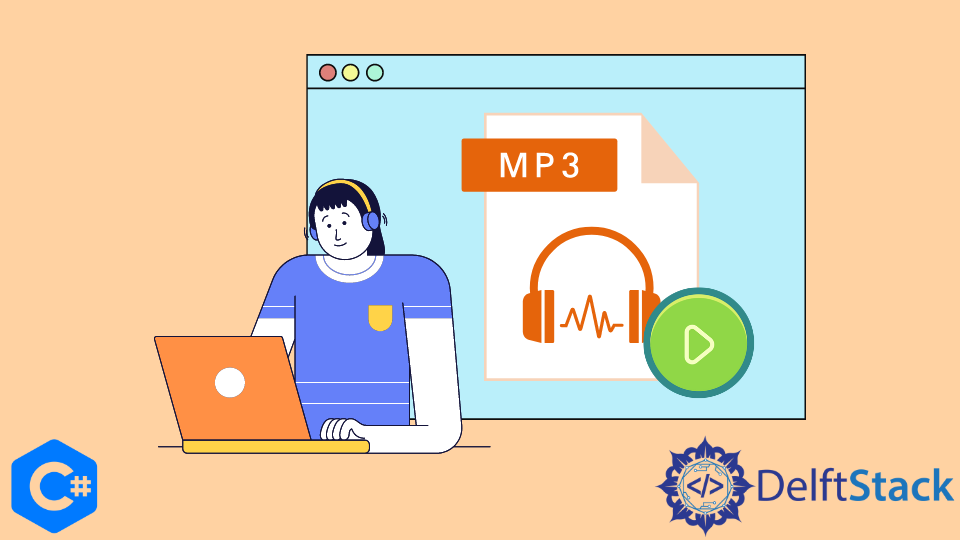
這篇文章將演示如何使用你的 C# Windows 窗體應用程式和 Windows Media Player 構建一個簡單的 C# mp3 播放器。
使用 C# Windows 窗體應用程式建立一個簡單的 Mp3 播放器
首先,我們將按照以下步驟建立一個新的 Windows 窗體。
-
開啟 Microsoft Visual Studio 2017 或更新版本。
-
在左上角,找到
檔案
並開啟一個新專案。 -
然後選擇
Windows Forms Application
,為其命名,然後單擊OK
。 -
點選
OK
後,會出現一個新的空窗體。
現在,我們將設計表單。
建立 Windows 窗體後,我們將對其進行設計。如果你的工具箱
中沒有 Windows Media Player 專案,請按照以下步驟操作。
-
右鍵單擊
Components
,然後單擊工具箱區域中的Choose Items
,將出現一個新視窗。 -
選擇
COM Components
選項卡,然後單擊列表中的Windows Media Player
項。
新增專案:
-
將
Windows Media Player
拖放到窗體上,然後調整其位置。 -
新增一個
ListBox
並將其命名為Audiolist
以選擇 mp3 檔案列表。 -
現在從工具箱中新增一個按鈕並將其命名為
ChooseButton
。我們使用一個按鈕來選擇一個播放列表,當你從列表框中選擇一個時,列表框將顯示將在Windows Media Player
中播放的音訊檔案的播放列表。
我們可以按照這些特定步驟建立一個 MP3 Player Windows 窗體。
需要的庫:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
首先,初始化兩個字串陣列型別的全域性變數 file
和 filepath
。
string[] file, filepath;
現在建立一個名為 fileOpen
的 OpenFileDialog
型別變數,它將用於啟動一個對話方塊以從儲存中選擇檔案。
OpenFileDialog fileOpen = new OpenFileDialog();
然後我們將應用一個條件並將以下內容作為引數傳遞。
if (fileOpen.ShowDialog() == System.Windows.Forms.DialogResult.OK)
如果該表示式為真,檔名將儲存在 file
變數中。
file = fileOpen.SafeFileNames;
使用以下命令,將檔案的整個路徑儲存在名為 filepath
的路徑變數中。
filepath = fileOpen.FileNames;
然後我們將使用 for
迴圈在 ListBox
中新增音訊檔案。
for (int i = 0; i < file.Length; i++){
Audiolist.Items.Add(file[i]);
}
之後,雙擊產生 ListBox
的點選事件。
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {
}
然後將此程式碼貼上到 Audiolist
正文中。此程式碼會將列表或該檔案的路徑傳輸到你的 Windows Media Player 中,無論你在 ListBox
中為其指定的名稱如何。
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {
axWindowsMediaPlayer1.URL = filepath[Audiolist.SelectedIndex];
}
下面是使用 C# Windows 窗體應用程式和 Windows Media Player 構建簡單 C# mp3 播放器的原始碼。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Mp3Player
{
public partial class Form1: Form
{
string[] file, filepath;
public Form1()
{
InitializeComponent();
}
private void ChooseButton_Click(object sender, EventArgs e){
OpenFileDialog fileOpen=new OpenFileDialog();
if (fileOpen.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
file = fileOpen.SafeFileNames;
filepath = fileOpen.FileNames;
for (int i = 0; i < file.Length; i++)
{
Audiolist.Items.Add(file[i]);
}
}
}
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e){
axWindowsMediaPlayer1.URL = filepath[Audiolist.SelectedIndex];
}
}
}
使用 DirectShow
功能在 C#
中建立 Mp3 Windows 窗體應用程式
使用 DirectShow
功能建立帶有 Windows Media Player 的 Mp3 Windows 表單應用程式。
Tlbimp
將 COM 型別庫中的型別定義轉換為通用語言的可執行程式集中的可比較定義。
Tlbimp.exe
的輸出是一個二進位制檔案(一個程式集),其中包含原始型別庫中宣告的型別的執行時後設資料。
要建立 QuartzTypeLib.dll
,請按照下列步驟操作。
-
首先執行
tlbimp
工具。 -
執行
TlbImp.exe %windir%\system32\quartz.dll
(此位置或目錄可能因裝置而異。) -
輸出將是
QuartzTypeLib.dll
。 -
在
Solution Explorer
中的專案名稱上單擊滑鼠右鍵,然後選擇Add
選單項,然後選擇Reference
。它會將QuartzTypeLib.dll
新增到你的專案中作為 COM 引用。 -
在你的專案中展開
References
並查詢QuartzTypeLib
參考。通過右鍵單擊並選擇屬性將Embed Interop Types
更改為 false。 -
禁用專案設定中的
Prefer 32-bit
設定。如果你不想獲得例外,請構建選項卡。
你可以使用此原始碼建立 Mp3 Windows 表單應用程式。
using QuartzTypeLib;
public sealed class DirectShowPlayer
{
private FilgraphManager fgm;
public void Play(string filepath){
FilgraphManager fgm= new FilgraphManager();
fgm.RenderFile(filepath);
fgm.Run();
}
public void Stop()
{
fgm?.Stop();
}
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn