在 C# 中將列表轉換為 IEnumerable
-
使用 C# 中的
as
關鍵字將列表轉換為 IEnumerable - 使用 C# 中的型別轉換方法將列表轉換為 IEnumerable
- 使用 C# 中的 LINQ 方法將列表轉換為 IEnumerable
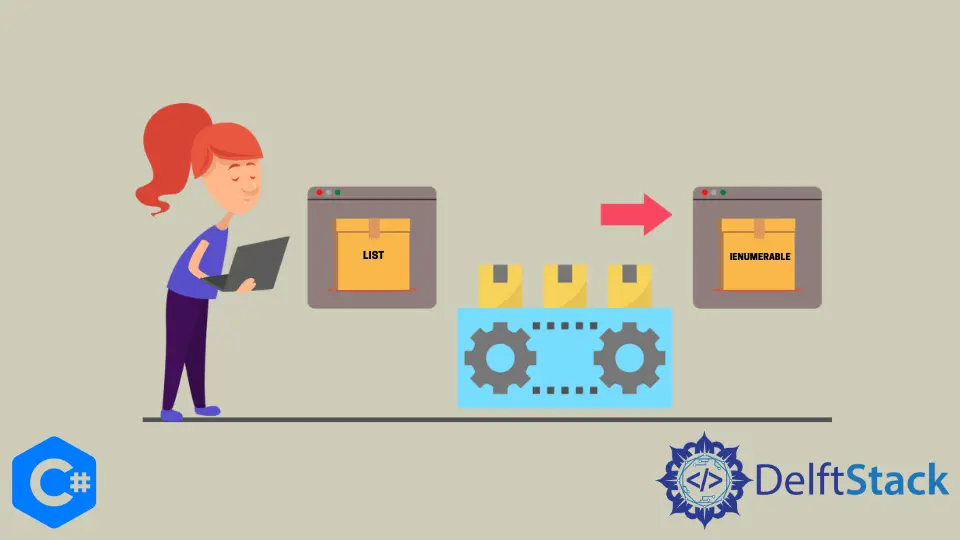
本教程將討論在 C# 中將列表轉換為 IEnumerable
的方法。
使用 C# 中的 as
關鍵字將列表轉換為 IEnumerable
我們可以使用 C# 中的 as
關鍵字將 List
資料結構轉換為 IEnumerable
資料結構。請參見以下示例。
using System;
using System.Collections.Generic;
namespace list_to_ienumerable {
class Program {
static void Main(string[] args) {
List<int> ilist = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> enumerable = ilist as IEnumerable<int>;
foreach (var e in enumerable) {
Console.WriteLine(e);
}
}
}
}
輸出:
1
2
3
4
5
在上面的程式碼中,我們使用 C# 中的 as
關鍵字將整數 ilist
的 List
轉換為整數 enumerable
的 IEnumerable
。
使用 C# 中的型別轉換方法將列表轉換為 IEnumerable
我們還可以使用型別轉換方法將 List
資料型別的物件儲存到 IEnumerable
資料型別的物件中,如以下程式碼示例所示。
using System;
using System.Collections.Generic;
namespace list_to_ienumerable {
class Program {
static void Main(string[] args) {
List<int> ilist = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> enumerable = (IEnumerable<int>)ilist;
foreach (var e in enumerable) {
Console.WriteLine(e);
}
}
}
}
輸出:
1
2
3
4
5
在上面的程式碼中,我們使用 C# 中的型別轉換方法將整數 ilist
的列表轉換為整數 enumerable
的 IEnumerable
。
使用 C# 中的 LINQ 方法將列表轉換為 IEnumerable
LINQ 將 SQL 查詢功能與 C# 中的資料結構整合在一起。我們可以使用 LINQ 的 AsEnumerable()
函式將列表轉換為 C# 中的 IEnumerable
。下面的程式碼示例向我們展示瞭如何使用 C# 中的 LINQ 的 AsEnumerable()
函式將 List
資料結構轉換為 IEnumerable
資料結構。
using System;
using System.Collections.Generic;
using System.Linq;
namespace list_to_ienumerable {
class Program {
static void Main(string[] args) {
List<int> ilist = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> enumerable = ilist.AsEnumerable();
foreach (var e in enumerable) {
Console.WriteLine(e);
}
}
}
}
輸出:
1
2
3
4
5
在上面的程式碼中,我們使用 C# 中的 ilist.AsEnumerable()
函式將整數 ilist
的 List
轉換為整數 enumerable
的 IEnumerable
。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn