在 C# 中讀取和寫入 INI 檔案
-
C#
中的INI
檔案是什麼 -
在
C# 中使用
WritePrivateProfileString` 來儲存 INI 檔案中新的和修改的設定 -
在
C# 中使用
ReadPrivateProfileString` 從一個 INI 檔案中讀取資料 -
在
C#
中讀取和寫入INI
檔案
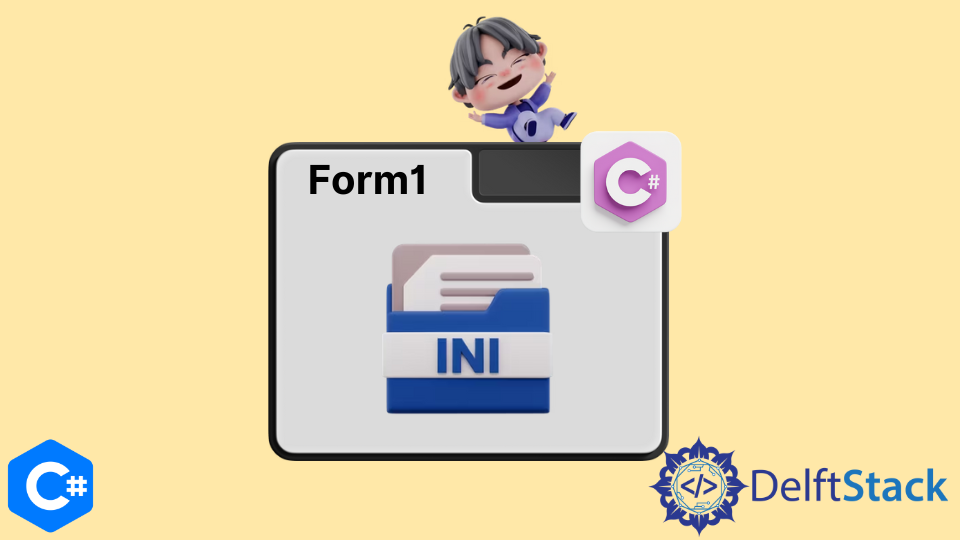
本教程教授如何在 C# 中讀取和寫入 INI
檔案。
C#
中的 INI
檔案是什麼
初始化檔案,通常稱為 INI
檔案,是用於儲存程式配置資訊的文字檔案。 .NET 框架本身不支援 INI
檔案。
你可以利用 Windows API 方法使用平臺呼叫服務來寫入和讀取檔案。本教程將構建一個表示 INI
檔案的類,並使用 Windows API 操作來操作它們。
要使用 INI
檔案,我們需要兩個 Windows API 函式,WritePrivateProfileString
和 ReadPrivateProfileString
。
在 C# 中使用
WritePrivateProfileString` 來儲存 INI 檔案中新的和修改的設定
在 INI
檔案中,WritePrivateProfileString
儲存新的和修改的設定。結構如下。
bool WritePrivateProfileString(string name,string key,string value,string filepath);
如果寫入成功,則該函式返回布林結果,如果寫入失敗,則返回 false。以下是引數。
- 要寫入的部分名稱由
name
指定。 - 要設定的鍵名由
key
指定。 - 鍵的值由
value
指定。 filepath
儲存要更新的INI
檔案的位置。
在 C# 中使用
ReadPrivateProfileString` 從一個 INI 檔案中讀取資料
ReadPrivateProfileString
從 INI
檔案中讀取資料。該函式根據其應用程式讀取單個值、節中的所有鍵名或所有部分的名稱。
int GetPrivateProfileString(string name,string key,string default,StringBuilder ReturnedVal,int maxsize,string filepath);
函式將返回值中的字元數作為無符號整數返回。以下是引數。
- 要讀取的部分名稱由
name
指定。如果將其設定為 null,則將返回所有部分名稱。 - 要讀取的鍵名由
key
指定。如果設定為 null 而name
不為 null,則返回的值將包括給定部分中鍵的名稱。 - 如果鍵不存在,
default
指定要返回的預設值。 ReturnedVal
接收用作緩衝區的字串。操作的結果被寫入緩衝區,修改字串的值。ReturnedVal
中將返回的字串的最大大小由maxsize
指定。這應該與緩衝區大小相同。如果從INI
檔案中讀取的值比ReturnedVal
長,它將被減少。- 要讀取的
INI
檔案的位置和名稱由filepath
指定。該檔案必須存在。
在 C#
中讀取和寫入 INI
檔案
讓我們建立一個類來儲存我們將使用的 Windows API 函式的結構,因為我們已經看到了它們的結構。
新增以下庫。
using System;
using System.IO;
using System.ComponentModel;
using System.Runtime.InteropServices;
using System.Text;
建立一個名為 ReadWriteINIfile
的新類並使用以下類程式碼。因為類本身沒有訪問修飾符,所以它會有一個內部作用域。
IniFile
類將訪問 API 函式而不將它們暴露給公眾。
public class ReadWriteINIfile{
[DllImport("kernel32")]
private static extern long WritePrivateProfileString(string name,string key,string val,string filePath);
[DllImport("kernel32")]
private static extern int GetPrivateProfileString(string section,string key,string def,StringBuilder retVal,int size,string filePath);
}
我們現在可以構造 ReadWriteINIfile
類,允許我們在不知道 API 操作的情況下讀取 INI
檔案。
將以下程式碼新增到類以建立建構函式。
public ReadWriteINIfile(string inipath)
{
path = inipath;
}
WritePrivateProfileString
用於用值填充 INI
檔案。下面的程式碼中使用了三個引數來接受節和鍵名以及要儲存的值。
在對 WritePrivateProfileString
的呼叫中,這些值與傳遞給建構函式的檔名組合在一起。
public void WriteINI(string name,string key,string value)
{
WritePrivateProfileString(name,key,value,this.path);
}
ReadINI
的引數是要讀取的 name
和 key
。上面程式碼的第一行定義了字串並將其值設定為幾個等於其最大長度的空格。
GetPrivateProfileString
函式接收此字串作為要填充的緩衝區。使用下面的程式碼。
public string ReadINI(string name,string key)
{
StringBuilder sb = new StringBuilder(255);
int ini = GetPrivateProfileString(name,key,"",sb,255,this.path);
return sb.ToString();
}
完整原始碼:
using System;
using System.IO;
using System.ComponentModel;
using System.Runtime.InteropServices;
using System.Text;
namespace Test{
class Program
{
static void Main(string[] args)
{ }
}
public class ReadWriteINIfile{
[DllImport("kernel32")]
private static extern long WritePrivateProfileString(string name,string key,string val,string filePath);
[DllImport("kernel32")]
private static extern int GetPrivateProfileString(string section,string key,string def,StringBuilder retVal,int size,string filePath);
public string path;
public ReadWriteINIfile(string inipath)
{
path = inipath;
}
public void WriteINI(string name,string key,string value)
{
WritePrivateProfileString(name,key,value,this.path);
}
public string ReadINI(string name,string key)
{
StringBuilder sb = new StringBuilder(255);
int ini = GetPrivateProfileString(name,key,"",sb,255,this.path);
return sb.ToString();
}
}
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn