在 C# 中將布林值轉換為整數
Abdullahi Salawudeen
2024年2月16日
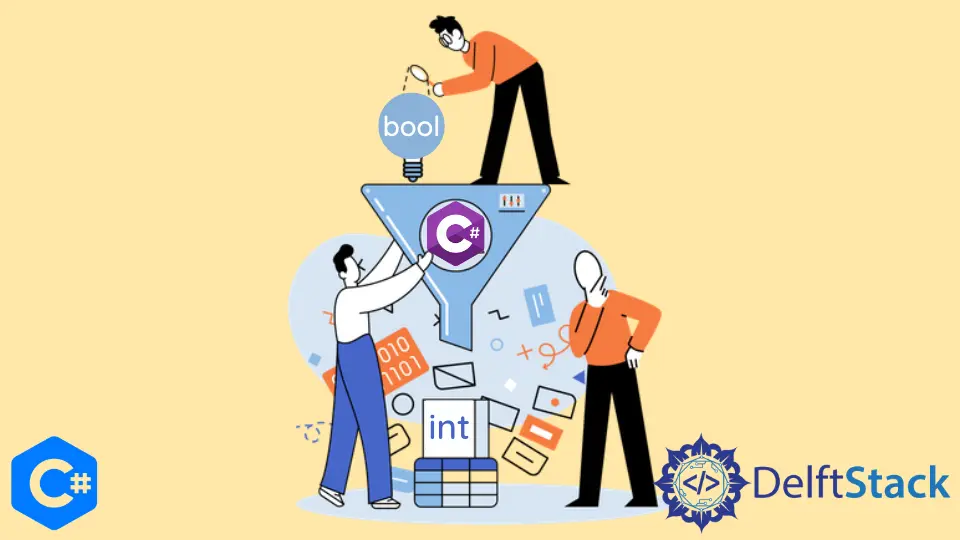
本文將介紹在 C# 中將布林資料型別轉換為整數。
在 C# 中使用 ConvertToInt32
語句將布林值轉換為整數
傳統上,沒有將資料型別從布林值隱式轉換為整數。但是,Convert.ToInt32()
方法將指定值轉換為 32 位有符號整數。
值得一提的是,Convert.ToInt32()
方法類似於 int.Parse()
方法,但 int.Parse()
方法只接受字串資料型別作為引數並丟擲錯誤當一個 null 作為引數傳遞時。
相比之下,Convert.ToInt32()
方法不受這些限制的影響。
進一步的討論可通過本參考文獻獲得。
下面是使用 Convert.ToInt32()
方法的程式碼示例。
// C# program to illustrate the
// use of Convert.ToInt32 statement
// and Convert.ToBoolean
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = Convert.ToInt32(boolinput);
bool boolRresult = Convert.ToBoolean(intRresult);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
輸出:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
下面是使用 int.Parse()
方法的程式碼示例。
// C# program to illustrate the
// use of int.Parse statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = int.Parse(boolinput);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
}
}
輸出:
program.cs(12,30): error CS1503: Argument 1: cannot convert from 'bool' to 'string'
使用 int.Parse
方法將 Boolean
資料型別轉換為 integer
會顯示上述錯誤。可以看出,該方法需要一個字串
作為引數,而不是一個布林
資料型別。
在 C# 中使用 switch
語句將布林值轉換為整數
switch
語句用於在程式執行期間有條件地分支。它決定要執行的程式碼塊。將表示式的值與每個案例的值進行比較,
如果匹配,則執行相關的程式碼塊。switch
表示式只計算一次。
下面是使用 switch
語句的程式碼示例。
// C# program to illustrate the
// use of switch statement
using System;
namespace Test {
class Program {
static void Main(string[] args) {
int i = 1;
bool b = true;
switch (i) {
case 0:
b = false;
Console.WriteLine("When integer is 0, boolean is:");
Console.WriteLine(b);
break;
case 1:
b = true;
Console.WriteLine("When integer is 1, boolean is:");
Console.WriteLine(b);
break;
}
switch (b) {
case true:
i = 1;
Console.WriteLine("When boolean is true, integer is:");
Console.WriteLine(i);
break;
case false:
i = 0;
Console.WriteLine("When boolean is false, integer is:");
Console.WriteLine(i);
break;
}
}
}
}
輸出:
When an integer is 1, boolean is:
True
When boolean is true, integer is:
1
在 C# 中使用三元條件運算子將布林值轉換為整數
條件運算子 ?:
,也稱為三元條件運算子,類似於 if
語句。它計算一個布林表示式並返回兩個表示式之一的結果。
如果布林表示式為真,則返回第一條語句(即 ?
之後的語句);返回第二個語句(即 :
之後的語句)。進一步的討論可通過本參考文獻獲得。
下面是一個程式碼示例。
// C# program to illustrate the
// use of ternary operator
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = boolinput ? 1 : 0;
bool boolRresult = (intRresult == 1) ? true : false;
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
輸出:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
在 C# 中使用 if
語句將布林值轉換為整數
if
語句在執行某些邏輯表示式後檢查特定條件是真還是假。每當表示式返回 true
時,都會返回數值 1,即 0。
類似地,當數值為 1 時,返回布林值 true
。否則,返回 false
。
下面是一個程式碼示例。
// C# program to illustrate the
// use of if statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intResult;
if (boolinput) {
intResult = 1;
} else {
intResult = 0;
}
bool boolResult;
if (intResult == 1) {
boolResult = true;
} else {
boolResult = false;
}
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intResult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolResult);
}
}
輸出:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True