在 C# 中向陣列中新增字串
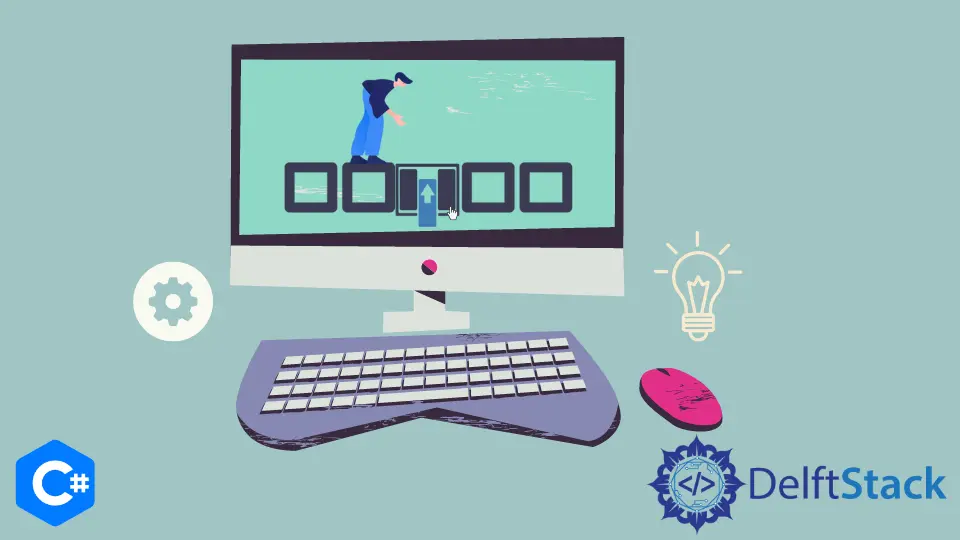
本教程將討論在 C# 中向一個完全填充的陣列新增新字串的方法。
使用 C# 中的 List.Add()
方法將字串新增到陣列
不幸的是,沒有內建的方法可以在 C# 中向陣列新增新值。在 C# 中,List 資料結構應該被用於動態分配和取消分配。但是,如果我們有一個包含一些重要資料的填充陣列,並且想向該陣列新增一個新元素,則可以遵循以下過程。Linq 用於在 C# 中的資料結構上整合 SQL 的查詢功能。我們可以使用 Linq 的 ToList()
方法將陣列轉換為列表,然後使用 C# 中的 List.Add()
方法將值新增到列表中。最後,我們可以使用 List.ToArray()
方法將列表轉換回陣列。下面的程式碼示例向我們展示瞭如何使用 C# 中的 List.Add()
方法將新元素新增到完全填充的陣列中。
using System;
using System.Collections.Generic;
using System.Linq;
namespace Array_Add {
class Program {
static void Main(string[] args) {
string[] arr = { "One", "Two", "Three" };
string newElement = "New Element";
List<string> list = new List<string>(arr.ToList());
list.Add(newElement);
arr = list.ToArray();
foreach (var e in arr) {
Console.WriteLine(e);
}
}
}
}
輸出:
One
Two
Three
New Element
我們在上面的程式碼中初始化了字串 arr
和字串變數 newElement
的陣列。我們使用 arr.ToList()
方法將 arr
陣列轉換為列表 list
。然後,我們使用 list.Add(newElement)
方法將 newElement
新增到 list
中。最後,我們使用 C# 中的 list.ToArray()
方法將 list
列表轉換回 arr
陣列。
使用 C# 中的 Array.Resize()
方法將字串新增到陣列
我們還可以使用以下程式碼將新元素新增到 C# 中完全填充的陣列中。Array.Resize()
方法更改 C# 中一維陣列的元素數。Array.Resize()
方法將對陣列及其新長度的引用作為引數,並調整陣列的大小。每次必須向其中新增一個新元素時,我們都可以將陣列大小增加一個元素。下面的程式碼示例向我們展示瞭如何使用 C# 中的 Array.Resize()
方法將一個新元素新增到一個完全填充的陣列中。
using System;
using System.Collections.Generic;
namespace Array_Add {
class Program {
static void Main(string[] args) {
string[] arr = { "One", "Two", "Three" };
string newElement = "New Element";
Array.Resize(ref arr, arr.Length + 1);
arr[arr.Length - 1] = newElement;
foreach (var e in arr) {
Console.WriteLine(e);
}
}
}
}
輸出:
One
Two
Three
New Element
我們在上面的程式碼中初始化了字串 arr
和 newElement
字串陣列。我們使用 Array.Resize()
函式將 arr
陣列的大小增加了一個元素,並將 newElement
分配給 arr
陣列的最後一個索引。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn