C++ STL 中的向量容器
-
在 C++ 中使用
std::vector
來構建動態陣列 -
在 C++ 中使用
std::vector
儲存struct
物件 -
在 C++ 中使用
std::vector::swap
函式交換向量元素
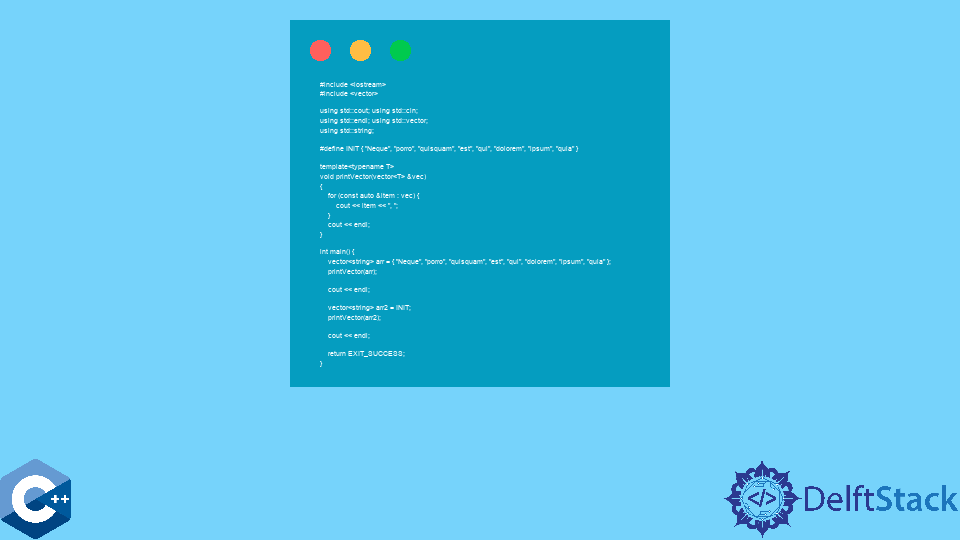
本文將介紹如何在 C++ 中使用 vector
容器的多種方法。
在 C++ 中使用 std::vector
來構建動態陣列
std::vector
是容器庫的一部分,它實現了可動態調整大小的陣列,該陣列將元素儲存在連續的儲存中。通常在結尾處新增 vector
元素,並帶有 push_back
或 emplace_back
內建函式。可以使用初始化程式列表輕鬆構建 vector
容器,如以下示例程式碼所示-arr
物件。同樣,可以將靜態初始化程式列表值儲存在巨集表示式中,並在需要時簡潔地表達初始化操作。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string;
#define INIT { "Neque", "porro", "quisquam", "est", "qui", "dolorem", "ipsum", "quia" }
template<typename T>
void printVector(vector<T> &vec)
{
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<string> arr = { "Neque", "porro", "quisquam", "est", "qui", "dolorem", "ipsum", "quia" };
printVector(arr);
cout << endl;
vector<string> arr2 = INIT;
printVector(arr2);
cout << endl;
return EXIT_SUCCESS;
}
在 C++ 中使用 std::vector
儲存 struct
物件
std::vector
可以用來儲存自定義結構體,並使用大括號列表表示法初始化它們,類似於前面的示例。注意,當使用 push_back
函式新增一個新的 struct
元素時,該值應作為支撐列表傳遞,並且如果 struct
中有多個巢狀結構,則應使用相應的符號。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string;
struct cpu {
string wine;
string country;
int year;
} typedef cpu;
int main() {
vector<cpu> arr3 = { {"Chardonnay", "France", 2018},
{"Merlot", "USA", 2010} };
for (const auto &item : arr3) {
cout << item.wine << " - "
<< item.country << " - "
<< item.year << endl;
}
arr3.push_back({"Cabernet", "France", 2015});
for (const auto &item : arr3) {
cout << item.wine << " - "
<< item.country << " - "
<< item.year << endl;
}
return EXIT_SUCCESS;
}
在 C++ 中使用 std::vector::swap
函式交換向量元素
std::vector
提供了多個內建函式來對其元素進行操作,其中之一就是 swap
。可以從 vector
物件中呼叫它,並以另一個 vector
作為引數交換它們的內容。另一個有用的函式是 size
,它可以在給定的向量物件中檢索當前元素計數。但是請注意,有一個類似的函式叫 capacity
,它返回當前分配的緩衝區中儲存的元素數量。有時會為後者分配更大的大小,以避免不必要地呼叫作業系統服務。下面的示例程式碼演示了兩個函式返回不同值的情況。
#include <iostream>
#include <vector>
using std::cout; using std::cin;
using std::endl; using std::vector;
template<typename T>
void printVector(vector<T> &vec)
{
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> arr4 = { 1, 12, 45, 134, 424 };
vector<int> arr5 = { 41, 32, 15, 14, 99 };
printVector(arr4);
arr4.swap(arr5);
printVector(arr4);
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
for (int i = 0; i < 10000; ++i) {
arr4.push_back(i * 5);
}
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
arr4.shrink_to_fit();
cout << "arr4 capacity: " << arr4.capacity() << " size: " << arr4.size()
<< endl;
return EXIT_SUCCESS;
}
輸出:
1, 12, 45, 134, 424,
41, 32, 15, 14, 99,
arr4 capacity: 5 size: 5
arr4 capacity: 10240 size: 10005
arr4 capacity: 10005 size: 10005
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn