在 C++ 中使用 STL 無序對映容器
-
使用
std::unordered_map
元素在 C++ 中宣告一個無序對映容器 -
使用
contains
成員函式檢查 C++ 對映中是否存在給定元素 -
使用
erase
成員函式從 C++ 中的對映中刪除特定元素 -
使用
insert
成員函式在 C++ 中向對映新增新元素
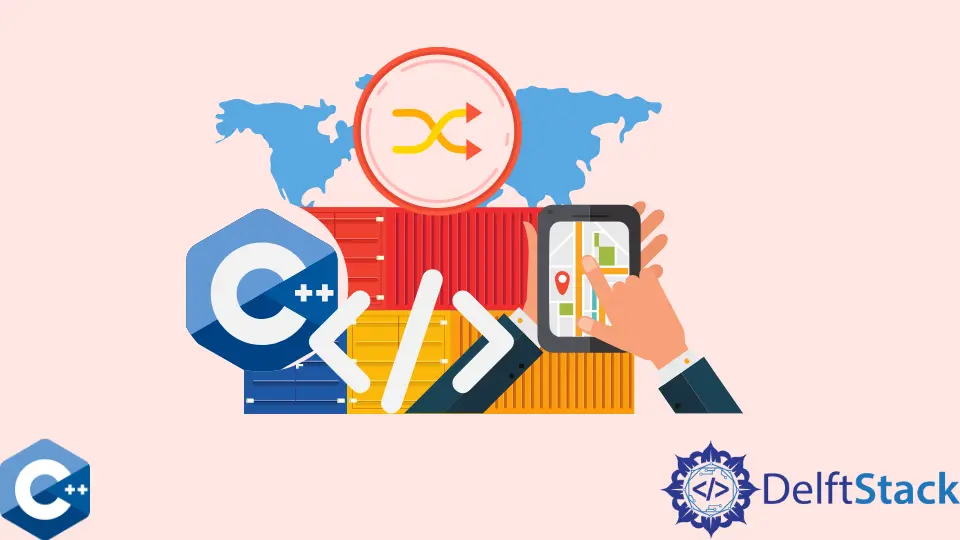
本文解釋瞭如何在 C++ 中使用 STL unordered_map
容器的幾種方法。
使用 std::unordered_map
元素在 C++ 中宣告一個無序對映容器
std::unordered_map
元素實現了一個鍵值對的關聯容器,其中每個鍵都是唯一的。與 std::map
不同,std::unordered_map
容器不按排序順序儲存元素。因此,容器主要用於元素查詢,位置無關緊要。此外,不能保證物件生命週期內元素的位置是固定的。因此,程式設計師應該將順序視為未定義。
以下示例演示了 unordered_map
容器的列表初始化,然後輸出兩個對映的內容。請注意,m1
元素的降序並不意味著它們已排序。
#include <iostream>
#include <map>
#include <unordered_map>
using std::cout;
using std::endl;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
std::map<int, string> m2 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
printMap(m1);
printMap(m2);
return EXIT_SUCCESS;
}
輸出:
4 : orange;
3 : grape;
2 : banana;
1 : apple;
1 : apple;
2 : banana;
3 : grape;
4 : orange;
使用 contains
成員函式檢查 C++ 對映中是否存在給定元素
自 C++20 更新以來,contains
成員函式已成為 std::unordered_map
容器的一部分。你可以使用該函式檢查 Map 中是否存在給定元素。此命令接受鍵值作為唯一引數,如果找到鍵,則返回 true
。該函式具有恆定的平均執行時間,最壞的情況是線性的,取決於容器本身的大小。
#include <iostream>
#include <map>
#include <unordered_map>
using std::cout;
using std::endl;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
for (int x : {1, 2, 3, 4, 5}) {
if (m1.contains(x)) {
cout << x << ": Found\n";
} else {
cout << x << ": Not found\n";
}
}
return EXIT_SUCCESS;
}
輸出:
1: Found
2: Found
3: Found
4: Found
5: Not found
使用 erase
成員函式從 C++ 中的對映中刪除特定元素
你可以使用 erase
成員函式從 Map 中刪除給定的鍵值對。該函式具有三個過載,第一個將迭代器帶到需要從容器中刪除的 map 元素。第二個函式過載需要兩個迭代器來指定範圍,該範圍將從 Map 中刪除。請注意,給定範圍必須是呼叫容器物件內的有效範圍。第三個過載可以獲取應該刪除的元素的鍵值。
在下面的示例中,我們刪除每個具有偶數作為鍵值的元素。
#include <iostream>
#include <map>
#include <unordered_map>
using std::cout;
using std::endl;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
for (auto it = m1.begin(); it != m1.end();) {
if (it->first % 2 == 0)
it = m1.erase(it);
else
++it;
}
printMap(m1);
return EXIT_SUCCESS;
}
輸出:
3 : grape;
1 : apple;
使用 insert
成員函式在 C++ 中向對映新增新元素
unordered_map
容器的另一個核心成員函式是 insert
函式,你可以使用它向 Map 新增新元素。該函式有多個過載,但我們使用第一個只接受鍵值對的過載。請注意,我們還使用 std::make_pair
函式來構造具有給定引數值的新對。
#include <iostream>
#include <map>
#include <unordered_map>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
vector<string> vec{"papaya", "olive", "melon"};
auto count = 0;
for (const auto& item : vec) {
m1.insert(std::make_pair(count, item));
}
printMap(m1);
return EXIT_SUCCESS;
}
輸出:
0 : papaya;
4 : orange;
3 : grape;
2 : banana;
1 : apple;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn