如何在 C++ 中對一個字串進行標記化
-
使用
find
和substr
函式在 C++ 中對一個字串進行標記化 -
在 C++ 中使用
std::stringstream
和getline
函式標記字串 -
在 C++ 中使用
istringstream
和copy
演算法對一個字串進行標記化
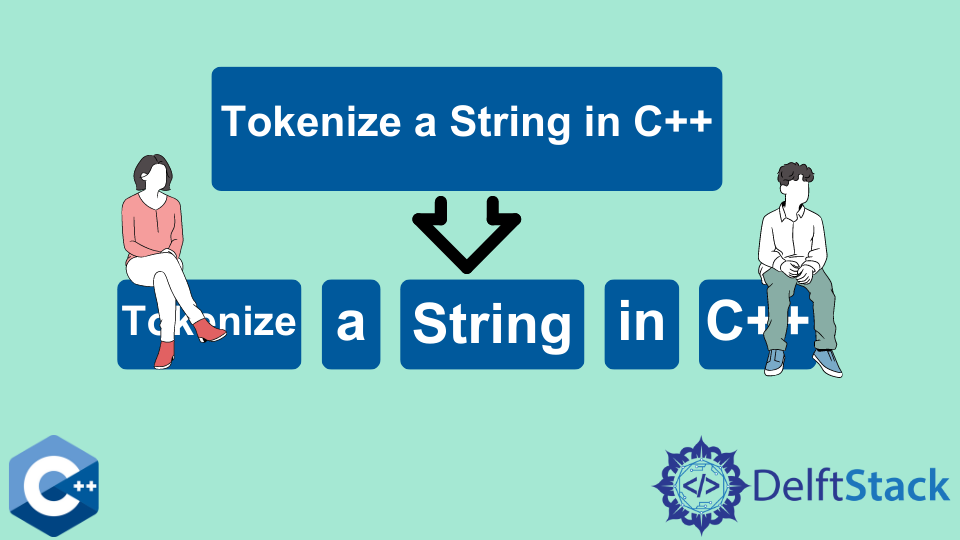
本文將介紹幾種在 C++ 中對字串進行標記化的方法。
使用 find
和 substr
函式在 C++ 中對一個字串進行標記化
std::string
類有一個內建的 find
函式,用於搜尋給定字串物件中的字元序列。find
函式返回在字串中找到的第一個字元的位置,如果沒有找到則返回 npos
。find
函式的呼叫插入到 if
語句中,對字串進行遍歷,直到提取出最後一個字串標記。
請注意,使用者可以指定任何字串型別的定界符,並將其傳遞給 find
方法。每次迭代時,標記都會被推送到字串的向量中,並通過 erase()
函式刪除已處理的部分。
#include <iostream>
#include <string>
#include <iterator>
#include <vector>
#include <algorithm>
using std::cout; using std::cin;
using std::endl; using std::string;
using std::vector;
string text = "Think you are escaping and run into yourself.";
int main(){
string delim = " ";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delim)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delim.length());
}
if (!text.empty())
words.push_back(text.substr(0, pos));
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
輸出:
Think
you
are
escaping
and
run
into
yourself.
在 C++ 中使用 std::stringstream
和 getline
函式標記字串
stringstream
可以用來提取一個要處理的字串,並使用 getline
提取標記,直到找到給定的定界符。注意,這個方法只適用於單字元定界符。
#include <iostream>
#include <string>
#include <sstream>
#include <iterator>
#include <vector>
#include <algorithm>
using std::cout; using std::cin;
using std::endl; using std::string;
using std::vector; using std::istringstream;
using std::stringstream;
int main(){
string text = "Think you are escaping and run into yourself.";
char del = ' ';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, del))
words.push_back(word);
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
輸出:
Think
you
are
escaping
and
run
into
yourself.
在 C++ 中使用 istringstream
和 copy
演算法對一個字串進行標記化
另外,也可以使用 <algorithm>
標頭檔案中的 copy
函式,在空白字元定界符上提取字串標記。在下面的例子中,我們只迭代並將標記流到標準輸出。為了用 copy
方法處理字串,我們將其插入 istringstream
中,並利用其迭代器。
#include <iostream>
#include <string>
#include <sstream>
#include <iterator>
#include <vector>
#include <algorithm>
using std::cout; using std::cin;
using std::endl; using std::string;
using std::vector; using std::istringstream;
using std::stringstream;
int main(){
string text = "Think you are escaping and run into yourself.";
string delim = " ";
vector<string> words{};
istringstream iss(text);
copy(std::istream_iterator<string>(iss),
std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
輸出:
Think
you
are
escaping
and
run
into
yourself.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn