在 C++ 中將字串轉換為整數陣列
-
使用
std::getline
和std::stoi
函式在 C++ 中將string
轉換為int
陣列 -
在 C++ 中使用
std::string::find
和std::stoi
函式將string
轉換為int
陣列 -
使用
std::copy
和std::remove_if
函式在 C++ 中將string
轉換為int
陣列
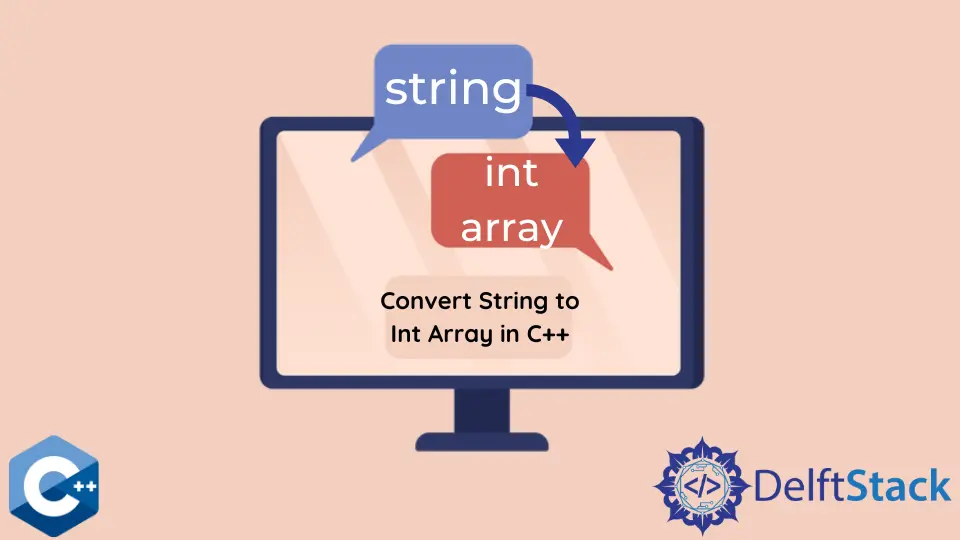
本文將演示有關如何在 C++ 中將字串轉換為整數陣列的多種方法。
使用 std::getline
和 std::stoi
函式在 C++ 中將 string
轉換為 int
陣列
std::stoi
用於將字串值轉換為帶符號的整數,它採用一個型別為 std::string
的強制性引數。可選地,該函式可以接受 2 個附加引數,其中第一個可用於儲存最後一個未轉換字元的索引。第三個引數可以選擇指定輸入的數字基數。注意,我們假設用逗號分隔的數字作為輸入字串,例如 .csv
檔案。因此,我們使用 getline
函式來解析每個數字,然後將該值傳遞給 stoi
。通過在每次迭代中呼叫 push_back
方法,我們還將每個數字儲存在 std::vector
容器中。
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<int> numbers;
int num;
stringstream text_stream(text);
string item;
while (std::getline(text_stream, item, ',')) {
numbers.push_back(stoi(item));
}
for (auto &n : numbers) {
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
輸出:
125
44
24
5543
111
在 C++ 中使用 std::string::find
和 std::stoi
函式將 string
轉換為 int
陣列
另外,我們可以利用 std::string
類的 find
內建方法來檢索逗號分隔符的位置,然後呼叫 substr
函式來獲取數字。然後,將 substr
結果傳遞到 stoi
,該 stoi
本身連結到 push_back
方法,以將數字儲存到 vector
中。請注意,while
迴圈後有一行是提取序列中最後一個數字所必需的。
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<int> numbers;
size_t pos = 0;
while ((pos = text.find(',')) != string::npos) {
numbers.push_back(stoi(text.substr(0, pos)));
text.erase(0, pos + 1);
}
numbers.push_back(stoi(text.substr(0, pos)));
for (auto &n : numbers) {
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
輸出:
125
44
24
5543
111
使用 std::copy
和 std::remove_if
函式在 C++ 中將 string
轉換為 int
陣列
提取整數的另一種方法是將 std::copy
演算法與 std::istream_iterator
和 std::back_inserter
結合使用。此解決方案將字串值儲存到向量
中,並將其輸出到 cout
流,但是可以輕鬆新增 std::stoi
函式以將每個元素轉換為 int
值。請注意,以下示例程式碼僅將數字儲存為字串值。
#include <iostream>
#include <iterator>
#include <sstream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::stoi;
using std::string;
using std::stringstream;
using std::vector;
int main(int argc, char *argv[]) {
string text = "125, 44, 24, 5543, 111";
vector<string> nums;
std::istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::back_inserter(nums));
for (auto &n : nums) {
n.erase(std::remove_if(n.begin(), n.end(), ispunct), n.end());
cout << n << endl;
}
exit(EXIT_SUCCESS);
}
輸出:
125
44
24
5543
111
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn