在 C++ 中對向量進行排序
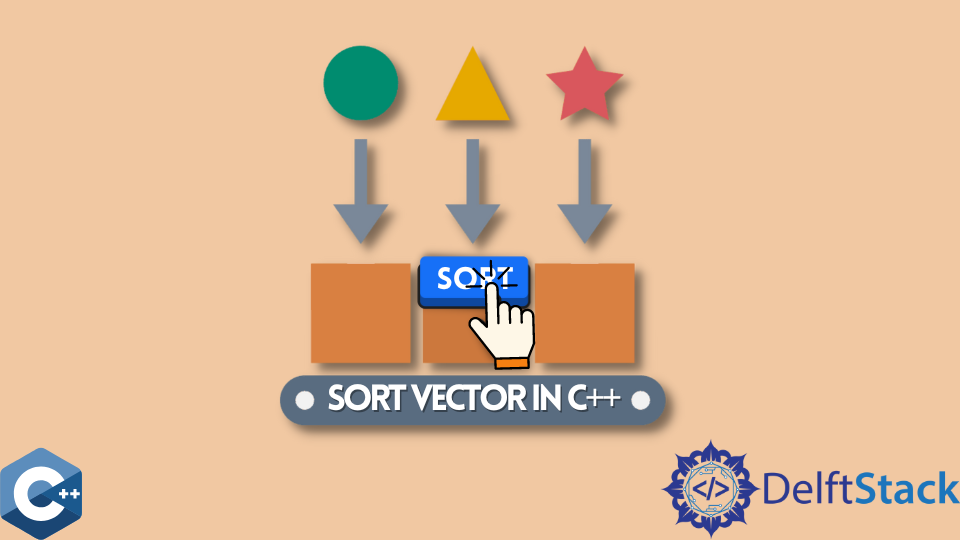
本文將演示如何在 C++ 中對向量進行排序的多種方法。
使用 std::sort
演算法對向量元素進行排序
std::sort
函式實現了一種通用演算法來處理不同的物件,並使用作為第三個引數傳遞的比較器函式對範圍內的給定元素進行排序。請注意,可以在不使用第三個引數的情況下使用該函式,在這種情況下,可以使用 operator<
對元素進行排序。以下示例程式碼演示了這種情況,其中元素的型別為字串,具有成員 operator<
,並且可以使用預設比較器進行排序。
#include <iostream>
#include <vector>
#include <algorithm>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string; using std::sort;
template<typename T>
void printVector(vector<T> &vec)
{
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<string> vec1 = { "highway",
"song",
"world",
"death",
"mom",
"historian",
"menu",
"woman" };
printVector(vec1);
sort(vec1.begin(), vec1.end());
printVector(vec1);
return EXIT_SUCCESS;
}
輸出:
highway, song, world, death, mom, historian, menu, woman,
death, highway, historian, menu, mom, song, woman, world,
使用帶有 Lambda 表示式的 std::sort
函式對結構體向量進行排序
或者,可以使用 lambda 表示式構造自定義比較器函式物件,以對使用者定義的結構進行排序。在這種情況下,我們有一個帶有不同資料成員的 struct cpu
,並且通過傳遞分別比較 value
或 property1
成員的函式物件來構造兩個 sort
呼叫。
#include <iostream>
#include <vector>
#include <algorithm>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string; using std::sort;
struct cpu {
string property1;
string property2;
string property3;
int value;
} typedef cpu;
void printVector(vector<cpu> &vec)
{
for (const auto &item : vec) {
cout << item.property1 << " : "
<< item.property2 << " : "
<< item.property3 << " : "
<< item.value << endl;
}
cout << endl;
}
int main() {
vector<cpu> vec3 = { {"WMP", "GR", "33", 2023},
{"TPS", "US", "31", 2020},
{"EOM", "GB", "36", 2021},
{"AAW", "GE", "39", 2024} };
printVector(vec3);
sort(vec3.begin(), vec3.end(), [] (cpu &x, cpu &y) { return x.value < y.value; });
sort(vec3.begin(), vec3.end(), [] (cpu &x, cpu &y) { return x.property1 < y.property1; });
printVector(vec3);
return EXIT_SUCCESS;
}
使用 std::sort
函式與自定義函式來排序結構體向量
請注意,以前的方法在較大的程式碼庫中很難使用,並且如果比較函式很複雜,可能會使程式碼變得相當龐大。另一個解決方案是將比較函式實現為結構體成員,並將它們宣告為 static
,以通過範圍運算子進行訪問。同樣,這些函式必須返回一個 bool
值,並且只有兩個引數。
#include <iostream>
#include <vector>
#include <algorithm>
using std::cout; using std::cin;
using std::endl; using std::vector;
using std::string; using std::sort;
struct cpu {
string property1;
string property2;
string property3;
int value;
public:
static bool compareCpusByValue(cpu &a, cpu &b) {
return a.value < b.value;
}
static bool compareCpusByProperty1(cpu &a, cpu &b) {
return a.property1 < b.property1;
}
} typedef cpu;
void printVector(vector<cpu> &vec)
{
for (const auto &item : vec) {
cout << item.property1 << " : "
<< item.property2 << " : "
<< item.property3 << " : "
<< item.value << endl;
}
cout << endl;
}
int main() {
vector<cpu> vec3 = { {"WMP", "GR", "33", 2023},
{"TPS", "US", "31", 2020},
{"EOM", "GB", "36", 2021},
{"AAW", "GE", "39", 2024} };
printVector(vec3);
sort(vec3.begin(), vec3.end(), cpu::compareCpusByProperty1);
sort(vec3.begin(), vec3.end(), cpu::compareCpusByValue);
printVector(vec3);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn