在 C++ 中按字母順序對字串排序
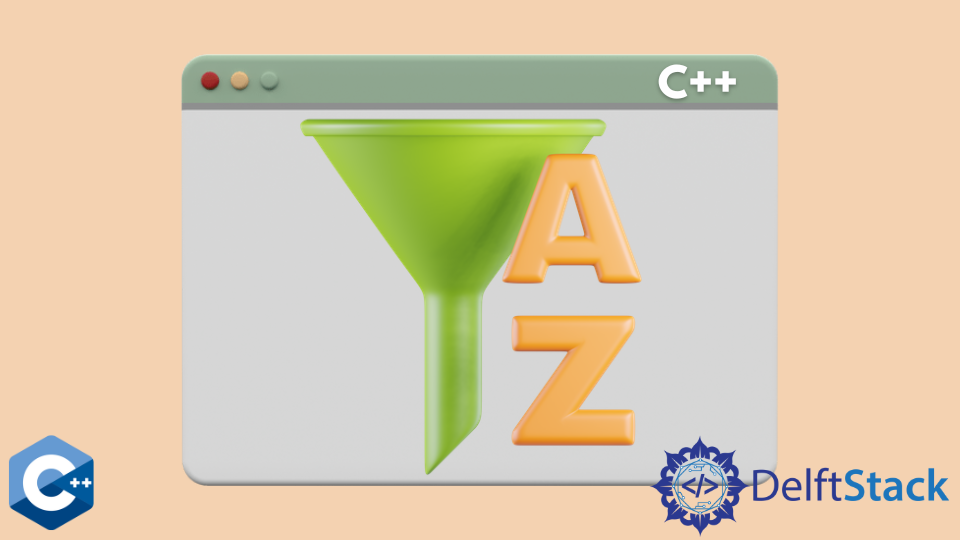
本文將演示如何在 C++ 中按字母順序對字串排序的多種方法。
使用 std::sort
演算法在 C++ 中按字母順序對字串進行排序
std::sort 是 STL 演算法庫的一部分,它為基於範圍的結構實現了通用的排序方法。該函式通常使用 operator<
對給定序列進行排序;因此,可以使用此預設行為按字母順序對字串物件進行排序。std::sort
只接受範圍說明符-第一個和最後一個元素迭代器。請注意,不能保證保留相等元素的順序。
在下面的示例中,我們演示了基本方案,其中對字串的向量進行了排序並列印到了 cout
流中。
#include <iostream>
#include <string>
#include <vector>
using std::cout; using std::sort;
using std::vector; using std::string;
using std::endl;
int main() {
vector<string> arr = { "raid", "implementation", "states", "all",
"the", "requirements", "parameter", "a",
"and", "or", "execution", "participate" };
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end());
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
輸出:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; all; and; execution; implementation; or; parameter; participate; raid; requirements; states; the;
另外,我們可以在 std::sort
方法中使用可選的第三個引數來指定確切的比較函式。在這種情況下,我們重新實現了前面的程式碼示例,以比較字串中的最後一個字母並對其進行相應的排序。注意比較函式應該有兩個引數,並返回一個 bool
值。下一個示例使用 lambda 表示式作為比較函式,並利用 back
內建函式檢索字串的最後一個字母。
#include <iostream>
#include <string>
#include <vector>
using std::cout; using std::sort;
using std::vector; using std::string;
using std::endl;
int main() {
vector<string> arr = { "raid", "implementation", "states", "all",
"the", "requirements", "parameter", "a",
"and", "or", "execution", "participate" };
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[] (string &s1, string &s2) { return s1.back() < s2.back(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
輸出:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; raid; and; the; participate; all; implementation; execution; parameter; or; states; requirements;
使用 std::sort
演算法在 C++ 中按長度對字串排序
對字串向量進行排序的另一種有用情況是按其長度排序。我們將使用與前面的示例程式碼相同的 lambda 函式結構,只是將 back
方法更改為 size
。不過請注意,該比較函式一定不能修改傳遞給它的物件。
#include <iostream>
#include <string>
#include <vector>
using std::cout; using std::sort;
using std::vector; using std::string;
using std::endl;
int main() {
vector<string> arr = { "raid", "implementation", "states", "all",
"the", "requirements", "parameter", "a",
"and", "or", "execution", "participate" };
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[] (string &s1, string &s2) { return s1.size() < s2.size(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
輸出:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; or; all; the; and; raid; states; parameter; execution; participate; requirements; implementation;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn