如何在 C++ 中從函式中返回一個字串
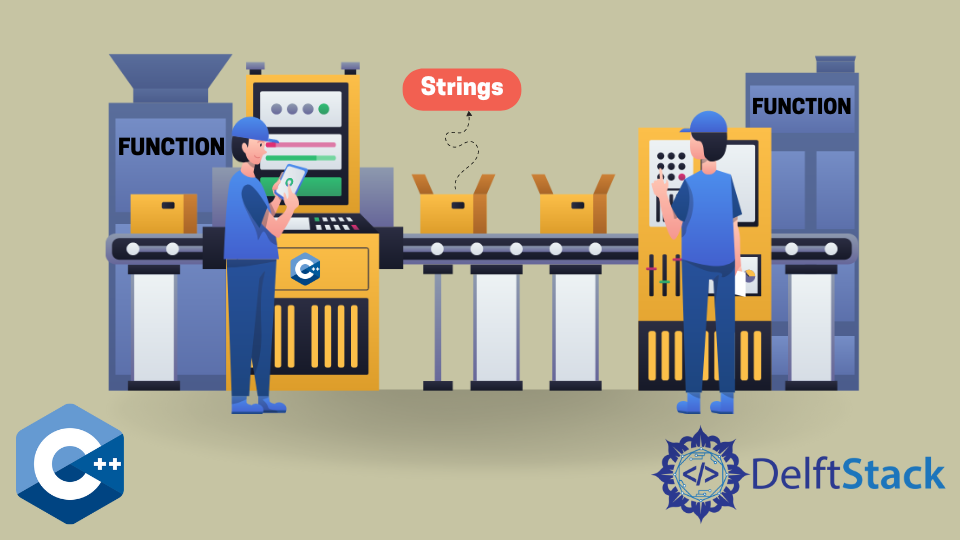
本文介紹了幾種在 C++ 中如何從函式中返回字串的方法。
使用 std::string func()
從 C++ 中的函式中返回字串
按值返回是從函式返回字串物件的首選方法。因為 std::string
類有 move
建構函式,所以即使是長字串,通過值返回也是高效的。如果一個物件有一個 move
建構函式,那麼它就被稱為具有移動語義的特性。移動語義意味著在函式返回時,物件不會被複制到不同的位置,因此,提供更快的函式執行時間。
#include <iostream>
#include <algorithm>
#include <iterator>
using std::cout; using std::endl;
using std::string; using std::reverse;
string ReverseString(string &s){
string rev(s.rbegin(), s.rend());
return rev;
}
int main() {
string str = "This string shall be reversed";
cout << str << endl;
cout << ReverseString(str) << endl;
return EXIT_SUCCESS;
}
輸出:
This string shall be reversed
desrever eb llahs gnirts sihT
使用 std::string &func()
記法從函式中返回字串
這個方法使用了通過引用返回的符號,這可以作為解決這個問題的另一種方法。儘管通過引用返回是返回大型結構或類的最有效的方法,但在這種情況下,與前一種方法相比,它不會帶來額外的開銷。注意,你不應該用引用來替換函式中宣告的區域性變數,這會導致懸空引用。
#include <iostream>
#include <algorithm>
#include <iterator>
using std::cout; using std::endl;
using std::string; using std::reverse;
string &ReverseString(string &s) {
reverse(s.begin(), s.end());
return s;
}
int main() {
string str = "Let this string be reversed";
cout << str << endl;
cout << ReverseString(str) << endl;
return EXIT_SUCCESS;
}
輸出:
Let this string be reversed
desrever eb gnirts siht teL
使用 char *func()
記法從函式中返回字串
另外,我們也可以使用 char *
從函式中返回一個字串物件。請記住,std::string
類以連續陣列的形式儲存字元。因此,我們可以通過呼叫內建的 data()
方法返回一個指向該陣列中第一個 char
元素的指標。然而,當返回 std::string
物件的空端字元陣列時,請確保不要使用類似的 c_str()
方法,因為它取代了指向第一個 char
元素的 const
指標。
#include <iostream>
#include <algorithm>
#include <iterator>
using std::cout; using std::endl;
using std::string; using std::reverse;
char *ReverseString(string &s) {
reverse(s.begin(), s.end());
return s.data();
}
int main() {
string str = "This string must be reversed";
cout << str << endl;
cout << ReverseString(str) << endl;
return EXIT_SUCCESS;
}
輸出:
This string must be reversed
desrever eb tsum gnirts sihT
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn