在 C++ 中通過引用傳遞向量
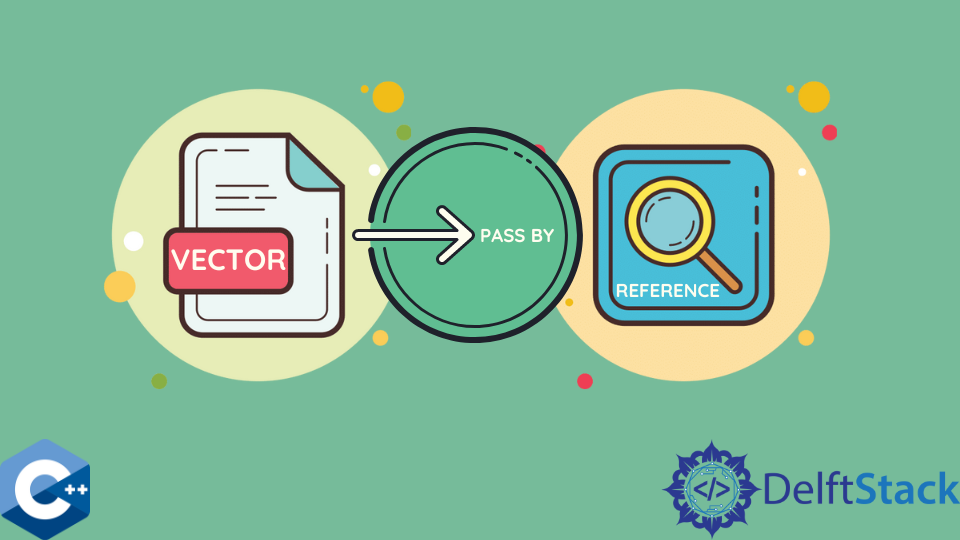
本文將演示有關如何在 C++ 中通過引用傳遞向量的多種方法。
使用 vector<T> &arr
表示法通過 C++ 中的引用傳遞向量
std::vector
是在 C++ 中儲存陣列的一種常用方法,因為它們為動態物件提供了多個內建函式來處理儲存的元素。請注意,vector
可能會佔用很大的記憶體,因此在將其傳遞給函式時應仔細考慮。通常,最佳做法是通過引用傳遞並在功能範圍內避開整個物件的副本。
在下面的示例中,我們演示了一個函式,該函式通過引用獲取單個整數向量並修改其元素。在主函式中的 multiplyByTwo
呼叫之前和之後,將列印 vector
元素。請注意,即使我們將返回值儲存在新變數 arr_mult_by2
中,我們也可以使用原始 arr
名稱訪問它,因為元素是在同一物件中修改的,並且沒有返回新副本。
#include <iostream>
#include <vector>
#include <iterator>
using std::cout;
using std::endl;
using std::vector;
using std::copy;
using std::string;
vector<int> &multiplyByTwo(vector<int> &arr){
for (auto &i : arr) {
i *= 2;
}
return arr;
}
int main() {
vector<int> arr = {1,2,3,4,5,6,7,8,9,10};
cout << "arr - ";
copy(arr.begin(), arr.end(),
std::ostream_iterator<int>(cout,"; "));
cout << endl;
auto arr_mult_by2 = multiplyByTwo(arr);
cout << "arr_mult_by2 - ";
copy(arr_mult_by2.begin(), arr_mult_by2.end(),
std::ostream_iterator<int>(cout,"; "));
cout << endl;
return EXIT_SUCCESS;
}
輸出:
arr - 1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
arr_mult_by2 - 2; 4; 6; 8; 10; 12; 14; 16; 18; 20;
使用 const vector<T> &arr
表示法通過 C++ 中的引用傳遞向量
另一方面,可以保證所傳遞的引用可以在函式定義中進行修改。const
限定符關鍵字提供了此功能,它告訴編譯器禁止在當前函式作用域中對給定物件進行任何修改。請注意,這似乎是可選的細節,不需要在開發人員中強調,但是有時這些關鍵字可以幫助編譯器優化機器程式碼以獲得更好的效能。
#include <iostream>
#include <vector>
#include <iterator>
using std::cout;
using std::endl;
using std::vector;
using std::copy;
using std::string;
vector<int> &multiplyByTwo(vector<int> &arr){
for (auto &i : arr) {
i *= 2;
}
return arr;
}
void findInteger(const vector<int> &arr) {
int integer = 10;
for (auto &i : arr) {
if (i == integer) {
cout << "found - " << integer << " in the array" << endl;
return;
}
}
cout << "couldn't find - " << integer << " in the array" << endl;
}
int main() {
vector<int> arr = {1,2,3,4,5,6,7,8,9,10};
auto arr_mult_by2 = multiplyByTwo(arr);
findInteger(arr);
findInteger(arr_mult_by2);
return EXIT_SUCCESS;
}
輸出:
found - 10 in the array
found - 10 in the array
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn