在 C++ 中使用 std::map::find 函式
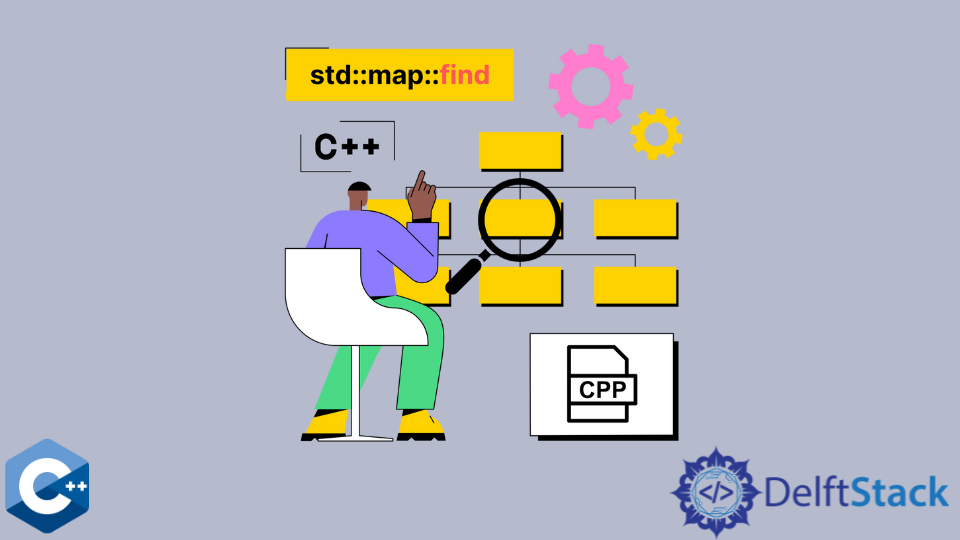
本文解釋瞭如何在 C++ 中使用 std::map::find
函式及其一些替代方法。
在 C++ 中使用 std::map::find
函式查詢具有給定鍵值的元素
std::map
物件是 C++ 標準模板庫中的關聯容器之一,它實現了一個排序的資料結構,儲存鍵值。請注意,鍵在 std::map
容器中是唯一的。因此,如果使用現有鍵插入新元素,則操作沒有任何效果。儘管如此,如果鍵匹配,std::map
類中的一些特殊成員函式可以為現有對分配新值(例如 insert_or_assign
函式)。
std::map
容器的優點包括快速搜尋、插入和刪除操作,這些操作可以在對數時間內進行。搜尋操作由 find
成員提供,它接受對鍵的引用。如果在 std::map
物件中找到給定的鍵,則返回對應元素的迭代器。另一方面,假設在容器中找不到給定的鍵,則返回尾後迭代器(map::end()
)。
以下程式碼片段演示了一個簡單的使用場景,其中 string
對的 map
容器用任意值初始化,然後搜尋具有 "h"
值的鍵。因此,我們使用 if-else
語句處理返回的迭代器,以列印元素對或輸出未找到給定鍵的訊息。
#include <iostream>
#include <map>
using std::cout; using std::cin;
using std::endl; using std::map;
using std::string;
int main(){
std::map<string, string> m1 = {{"h", "htop"},
{"k", "ktop"},
{"t", "ttop"},
{"r", "rtop"},
{"w", "wtop"},
{"p", "ptop"},};
string key = "h";
auto item = m1.find(key);
if (item != m1.end()) {
cout << "Key exists! - {" <<
item->first << ";" << item->second << "}\n";
} else {
cout << "Key does not exist!" << endl;
}
return EXIT_SUCCESS;
}
輸出:
Key exists! - {h;htop}
使用 contains
成員函式檢查 C++ 對映中是否存在給定元素
如果使用者需要確認具有給定值的一對是否存在於 map
物件中,可以使用成員函式 contains
。自 C++20 版本以來,該函式一直是 std::map
容器的一部分,因此你應該知道編譯器版本才能執行以下程式碼片段。contains
函式獲取對鍵的引用,如果找到,則返回 bool
值 true
。這個成員函式的時間複雜度也是對數的。
#include <iostream>
#include <map>
using std::cout; using std::cin;
using std::endl; using std::map;
using std::string;
int main(){
std::map<string, string> m1 = {{"h", "htop"},
{"k", "ktop"},
{"t", "ttop"},
{"r", "rtop"},
{"w", "wtop"},
{"p", "ptop"},};
string key = "h";
if (m1.contains(key)) {
cout << "Key Exists!" << endl;
} else {
cout << "Key does not exist!" << endl;
}
return EXIT_SUCCESS;
}
輸出:
Key Exists!
或者,可以使用 cout
成員函式來檢查具有給定鍵的元素對是否存在於 map
中。通常,cout
函式用於檢索具有給定鍵的元素數量,但由於 std::map
僅儲存唯一的鍵值,如果找到該元素,該過程將返回 1
。否則,返回零值以指示未找到元素。
#include <iostream>
#include <map>
using std::cout; using std::cin;
using std::endl; using std::map;
using std::string;
int main(){
std::map<string, string> m1 = {{"h", "htop"},
{"k", "ktop"},
{"t", "ttop"},
{"r", "rtop"},
{"w", "wtop"},
{"p", "ptop"},};
string key = "h";
if (m1.count(key)) {
cout << "Key Exists!" << endl;
} else {
cout << "Key does not exist!" << endl;
}
return EXIT_SUCCESS;
}
輸出:
Key Exists!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn