C++ 中的 INT_MAX 和 INT_MIN 巨集表示式
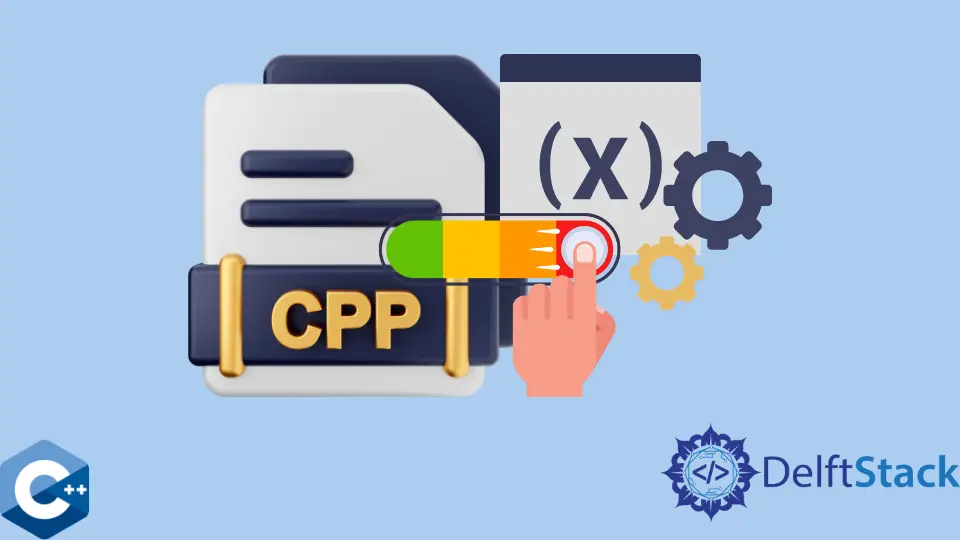
本文將演示有關如何在 C++ 中利用 INT_MAX 和 INT_MIN 巨集表示式的多種方法。
使用 INT_MIN
和 INT_MAX
來訪問 C++ 中特定於型別的限制
C++ 語言定義了多種內建資料型別,並規定了它們應占用多少記憶體以及相應的最大/最小值。像整數這樣的資料型別通常用於需要考慮其可能出現的最大值和最小值的計算中。儘管限制取決於特定型別的儲存大小,但這些限制會根據硬體平臺而有所不同。因此,我們需要使用固定的控制代碼訪問這些值,因此需要使用巨集表示式 INT_MIN
和 INT_MAX
。這些對應於 signed int
資料型別的最小值和最大值。下面的示例演示了 <climits>
標頭檔案下可用的多個巨集表示式。
#include <climits>
#include <iostream>
int main() {
printf("CHAR_BIT = %d\n", CHAR_BIT);
printf("MB_LEN_MAX = %d\n", MB_LEN_MAX);
printf("\n");
printf("CHAR_MIN = %+d\n", CHAR_MIN);
printf("CHAR_MAX = %+d\n", CHAR_MAX);
printf("SCHAR_MIN = %+d\n", SCHAR_MIN);
printf("SCHAR_MAX = %+d\n", SCHAR_MAX);
printf("UCHAR_MAX = %u\n", UCHAR_MAX);
printf("\n");
printf("SHRT_MIN = %+d\n", SHRT_MIN);
printf("SHRT_MAX = %+d\n", SHRT_MAX);
printf("USHRT_MAX = %u\n", USHRT_MAX);
printf("\n");
printf("INT_MIN = %+d\n", INT_MIN);
printf("INT_MAX = %+d\n", INT_MAX);
printf("UINT_MAX = %u\n", UINT_MAX);
printf("\n");
printf("LONG_MIN = %+ld\n", LONG_MIN);
printf("LONG_MAX = %+ld\n", LONG_MAX);
printf("ULONG_MAX = %lu\n", ULONG_MAX);
printf("\n");
printf("LLONG_MIN = %+lld\n", LLONG_MIN);
printf("LLONG_MAX = %+lld\n", LLONG_MAX);
printf("ULLONG_MAX = %llu\n", ULLONG_MAX);
printf("\n");
return EXIT_SUCCESS;
}
輸出:
CHAR_BIT = 8
MB_LEN_MAX = 16
CHAR_MIN = -128
CHAR_MAX = +127
SCHAR_MIN = -128
SCHAR_MAX = +127
UCHAR_MAX = 255
SHRT_MIN = -32768
SHRT_MAX = +32767
USHRT_MAX = 65535
INT_MIN = -2147483648
INT_MAX = +2147483647
UINT_MAX = 4294967295
LONG_MIN = -9223372036854775808
LONG_MAX = +9223372036854775807
ULONG_MAX = 18446744073709551615
LLONG_MIN = -9223372036854775808
LLONG_MAX = +9223372036854775807
ULLONG_MAX = 18446744073709551615
使用 INT_MIN
和 INT_MAX
在 C++ 中生成隨機數
隨機數通常是在某個固定範圍之間生成的。具有數字資料型別限制以相應地指定範圍引數,並且不會溢位生成的結果,這很有用。以下示例程式碼利用 uniform_int_distribution
來限制所生成數字的範圍。在這種情況下,我們指定了 INT_MAX
和 INT_MIN
值以從最大可用範圍中檢索整數。
#include <climits>
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
int main() {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_int_distribution<int> distr(INT_MIN, INT_MAX);
for (int n = 0; n < 6; ++n) {
cout << distr(eng) << "\n";
}
cout << endl;
return EXIT_SUCCESS;
}
-895078088
1662821310
-636757160
1830410618
1031005518
-438660615
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn