在 C++ 中使用抽象類實現介面
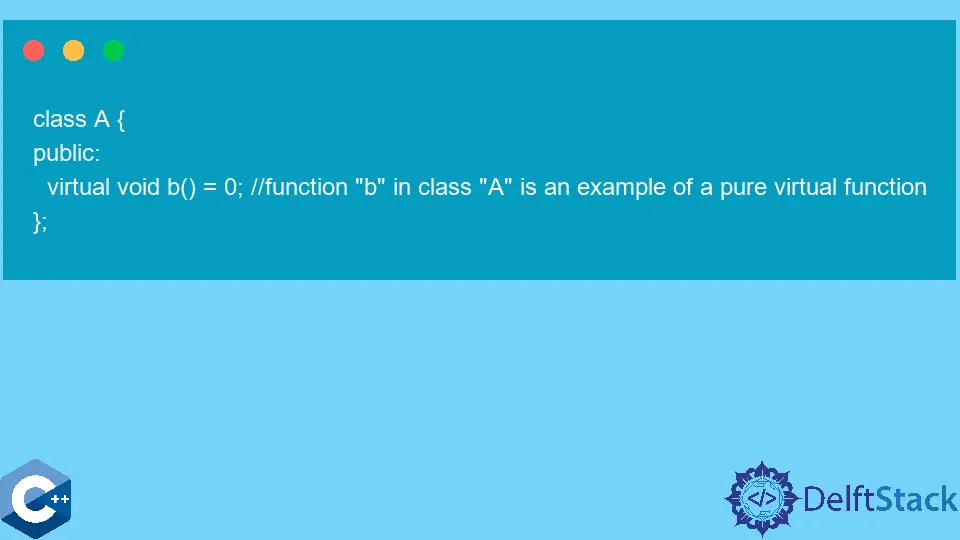
在 Java 中,多重繼承是使用 interface
實現的。interface
就像一個模板,因為它包含必要的佈局,但它是抽象的或沒有方法體。
對於 C++,不需要介面,因為 C++ 支援多重繼承,這與 Java 不同。在 C++ 中,介面的功能可以使用抽象類來實現。
C++ 中抽象類的概念
抽象類是具有至少一個純虛擬函式的類。只能宣告一個純虛擬函式,它不能有定義,它是通過在宣告中賦值 0
來宣告的。
抽象類對於使程式碼可重用和可擴充套件非常有用。這是因為可以建立許多從父類繼承但具有唯一定義的派生類。
此外,抽象類不能被例項化。
例子:
class A {
public:
virtual void b() = 0; // function "b" in class "A" is an example of a pure
// virtual function
};
在函式宣告期間,試圖在定義函式時使其成為純虛擬函式是行不通的。
使用 C++ 在程式中實現純虛擬函式
考慮一個以 shape
作為父類的示例。當涉及到形狀時,它們有許多不同的型別,當我們需要計算屬性時,例如面積,我們計算它的方式因形狀而異。
rectangle
和 triangle
作為從 shape
的父類繼承的派生類。然後,我們將在它們自己的派生類中為每個形狀(矩形
和三角形
)提供具有所需定義的純虛擬函式。
原始碼:
#include <iostream>
using namespace std;
class Shape {
protected:
int length;
int height;
public:
virtual int Area() = 0;
void getLength() { cin >> length; }
void getHeight() { cin >> height; }
};
class Rectangle : public Shape {
public:
int Area() { return (length * height); }
};
class Triangle : public Shape {
public:
int Area() { return (length * height) / 2; }
};
int main() {
Rectangle rectangle;
Triangle triangle;
cout << "Enter the length of the rectangle: ";
rectangle.getLength();
cout << "Enter the height of the rectangle: ";
rectangle.getHeight();
cout << "Area of the rectangle: " << rectangle.Area() << endl;
cout << "Enter the length of the triangle: ";
triangle.getLength();
cout << "Enter the height of the triangle: ";
triangle.getHeight();
cout << "Area of the triangle: " << triangle.Area() << endl;
return 0;
}
輸出:
Enter the length of the rectangle: 2
Enter the height of the rectangle: 4
Area of the rectangle: 8
Enter the length of the triangle: 4
Enter the height of the triangle: 6
Area of the triangle: 12
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.