如何在 C++ 中刪除字串兩邊字元
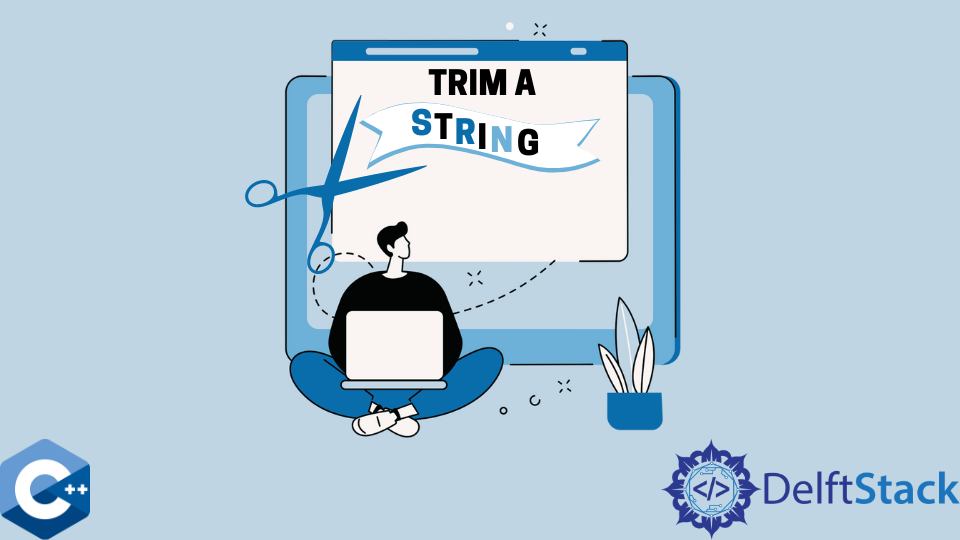
本文將介紹如何在 C++ 中刪除字串兩邊的字元。
使用 erase()
、find_first_not_of()
和 find_last_not_of()
方法實現刪除字串兩邊字元
由於標準的 C++ 庫中不包含用於字串修剪的函式,因此你需要自己實現這些函式,或者使用外部庫,如 Boost(參見字串演算法)。
在下面的例子中,我們將演示如何使用 2 個內建的 std::string
方法來構造自定義函式。首先,我們實現了 leftTrim
函式,它從字串的左側刪除作為引數傳遞的字元。我們任意指定要刪除的字元 .
、,
、/
和空格。
leftTrim
函式呼叫 find_first_not_of
方法,以查詢給定引數中第一個不等於 char
的字元,並返回一個位置給找到的字元。然後,erase
方法刪除從開始直到找到的位置的字元範圍。
#include <iostream>
#include <string>
using std::cout; using std::cin;
using std::endl; using std::string;
string& leftTrim(string& str, string& chars)
{
str.erase(0, str.find_first_not_of(chars));
return str;
}
int main(){
string chars_to_trim = ".,/ ";
string text = ",., C++ Standard";
cout << text << endl;
leftTrim(text, chars_to_trim);
cout << text << endl;
return EXIT_SUCCESS;
}
輸出:
,., C++ Standard
C++ Standard
或者,我們可以使用 trimLeft
函式從字串的右側刪除給定的字元。在這種情況下,我們利用 find_last_not_of
方法,搜尋最後一個等於沒有作為引數傳遞的字元。相應地,呼叫 erase
方法,引數為 found position + 1
。
注意,這兩個函式都是對字串進行原地操作,而不是返回刪除字元後的副本。
#include <iostream>
#include <string>
using std::cout; using std::cin;
using std::endl; using std::string;
string& rightTrim(string& str, string& chars)
{
str.erase(str.find_last_not_of(chars) + 1);
return str;
}
int main(){
string chars_to_trim = ".,/ ";
string text = "C++ Standard /././";
cout << text << endl;
rightTrim(text, chars_to_trim);
cout << text << endl;
return EXIT_SUCCESS;
}
輸出:
C++ Standard /././
C++ Standard
最後,我們可以結合前面的函式實現 trimString
函式,刪除兩邊的字元。該函式的引數與左/右版本相同。trimString
呼叫 leftTrim
,將 rigthTrim
函式的結果作為引數傳給它。你可以交換這些函式呼叫的位置而不改變程式的正確性。
#include <iostream>
#include <string>
using std::cout; using std::cin;
using std::endl; using std::string;
string& leftTrim(string& str, string& chars)
{
str.erase(0, str.find_first_not_of(chars));
return str;
}
string& rightTrim(string& str, string& chars)
{
str.erase(str.find_last_not_of(chars) + 1);
return str;
}
string& trimString(string& str, string& chars)
{
return leftTrim(rightTrim(str, chars), chars);
}
int main(){
string chars_to_trim = ".,/ ";
string text = ",,, .. C++ Standard ...";
cout << text << endl;
trimString(text, chars_to_trim);
cout << text << endl;
return EXIT_SUCCESS;
}
,,, .. C++ Standard ...
C++ Standard
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn