如何在 C++ 中從函式中返回向量
Jinku Hu
2023年10月12日
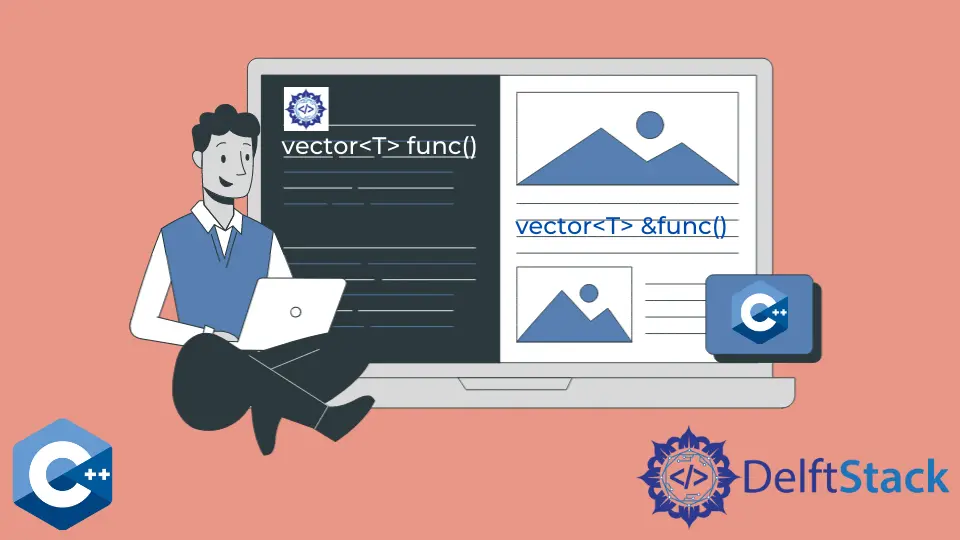
本文將介紹如何在 C++ 中有效地從函式中返回一個向量。
使用 vector<T> func()
記法從函式中返回向量
如果我們返回一個在函式中宣告的向量變數,那麼用值返回是首選方法。這種方法的效率來自於它的移動語義。它意味著返回一個向量並不複製物件,從而避免浪費額外的速度/空間。實際上,它將指標指向返回的向量物件,因此提供了比複製整個結構或類更快的程式執行時間。
#include <iostream>
#include <iterator>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
vector<int> multiplyByFour(vector<int> &arr) {
vector<int> mult;
mult.reserve(arr.size());
for (const auto &i : arr) {
mult.push_back(i * 4);
}
return mult;
}
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
vector<int> arrby4;
arrby4 = multiplyByFour(arr);
cout << "arr - | ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, " | "));
cout << endl;
cout << "arrby4 - | ";
copy(arrby4.begin(), arrby4.end(), std::ostream_iterator<int>(cout, " | "));
cout << endl;
return EXIT_SUCCESS;
}
輸出:
arr - | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
arrby4 - | 4 | 8 | 12 | 16 | 20 | 24 | 28 | 32 | 36 | 40 |
使用 vector<T> &func()
符號從函式中返回向量
這個方法使用了通過引用返回的符號,最適合於返回大型結構和類。注意,不要返回函式本身宣告的區域性變數的引用,因為這會導致懸空引用。在下面的例子中,我們通過引用傳遞 arr
向量,並將其也作為引用返回。
#include <iostream>
#include <iterator>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
vector<int> &multiplyByFive(vector<int> &arr) {
for (auto &i : arr) {
i *= 5;
}
return arr;
}
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
vector<int> arrby5;
cout << "arr - | ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, " | "));
cout << endl;
arrby5 = multiplyByFive(arr);
cout << "arrby5 - | ";
copy(arrby5.begin(), arrby5.end(), std::ostream_iterator<int>(cout, " | "));
cout << endl;
return EXIT_SUCCESS;
}
輸出:
arr - | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
arrby5 - | 5 | 10 | 15 | 20 | 25 | 30 | 35 | 40 | 45 | 50 |
作者: Jinku Hu
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn